
local fix version of myBlueUSB (http://mbed.org/users/networker/code/myBlueUSB/). - merge deleted files which are required to compile. - enable echo back of received data via RFCOMM.
Dependencies: AvailableMemory FatFileSystem mbed myUSBHost
AutoEvents.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "USBHost.h" 00026 #include "Utils.h" 00027 00028 #define AUTOEVT(_class,_subclass,_protocol) (((_class) << 16) | ((_subclass) << 8) | _protocol) 00029 #define AUTO_KEYBOARD AUTOEVT(CLASS_HID,1,1) 00030 #define AUTO_MOUSE AUTOEVT(CLASS_HID,1,2) 00031 00032 u8 auto_mouse[4]; // buttons,dx,dy,scroll 00033 u8 auto_keyboard[8]; // modifiers,reserved,keycode1..keycode6 00034 u8 auto_joystick[4]; // x,y,buttons,throttle 00035 00036 void AutoEventCallback(int device, int endpoint, int status, u8* data, int len, void* userData) { 00037 int evt = (int)userData; 00038 switch (evt) { 00039 case AUTO_KEYBOARD: 00040 printf("AUTO_KEYBOARD "); 00041 break; 00042 case AUTO_MOUSE: 00043 printf("AUTO_MOUSE "); 00044 break; 00045 default: 00046 printf("HUH "); 00047 } 00048 printfBytes("data",data,len); 00049 USBInterruptTransfer(device,endpoint,data,len,AutoEventCallback,userData); 00050 } 00051 00052 // Establish transfers for interrupt events 00053 void AddAutoEvent(int device, InterfaceDescriptor* id, EndpointDescriptor* ed) { 00054 if ((ed->bmAttributes & 3) != ENDPOINT_INTERRUPT || !(ed->bEndpointAddress & 0x80)) 00055 return; 00056 00057 // Make automatic interrupt enpoints for known devices 00058 u32 evt = AUTOEVT(id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00059 u8* dst = 0; 00060 int len; 00061 switch (evt) { 00062 case AUTO_MOUSE: 00063 dst = auto_mouse; 00064 len = sizeof(auto_mouse); 00065 break; 00066 case AUTO_KEYBOARD: 00067 dst = auto_keyboard; 00068 len = sizeof(auto_keyboard); 00069 break; 00070 default: 00071 printf("Interrupt endpoint %02X %08X\n",ed->bEndpointAddress,evt); 00072 break; 00073 } 00074 if (dst) { 00075 printf("Auto Event for %02X %08X\n",ed->bEndpointAddress,evt); 00076 USBInterruptTransfer(device,ed->bEndpointAddress,dst,len,AutoEventCallback,(void*)evt); 00077 } 00078 } 00079 00080 void PrintString(int device, int i) { 00081 u8 buffer[256]; 00082 int le = GetDescriptor(device,DESCRIPTOR_TYPE_STRING,i,buffer,255); 00083 if (le < 0) 00084 return; 00085 char* dst = (char*)buffer; 00086 for (int j = 2; j < le; j += 2) 00087 *dst++ = buffer[j]; 00088 *dst = 0; 00089 printf("%d:%s\n",i,(const char*)buffer); 00090 } 00091 00092 // Walk descriptors and create endpoints for a given device 00093 int StartAutoEvent(int device, int configuration, int interfaceNumber) { 00094 u8 buffer[255]; 00095 int err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00096 if (err < 0) 00097 return err; 00098 00099 int len = buffer[2] | (buffer[3] << 8); 00100 u8* d = buffer; 00101 u8* end = d + len; 00102 while (d < end) { 00103 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) { 00104 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00105 if (id->bInterfaceNumber == interfaceNumber) { 00106 d += d[0]; 00107 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) { 00108 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00109 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00110 d += d[0]; 00111 } 00112 } 00113 } 00114 d += d[0]; 00115 } 00116 return 0; 00117 } 00118 00119 // Implemented in main.cpp 00120 int OnDiskInsert(int device, unsigned char in, unsigned char out); 00121 00122 // Implemented in TestShell.cpp 00123 int OnBluetoothInsert(int device); 00124 00125 EndpointDescriptor* GetNextEndpoint(unsigned char *d) { 00126 while (d) { 00127 switch (d[1]) { 00128 case DESCRIPTOR_TYPE_INTERFACE: 00129 case DESCRIPTOR_TYPE_CONFIGURATION: 00130 return 0; 00131 case DESCRIPTOR_TYPE_ENDPOINT: 00132 return (EndpointDescriptor*)d; 00133 default: 00134 printf("Skipping descriptor %02X (%d bytes)\n",d[1],d[0]); 00135 } 00136 d += d[0]; 00137 } 00138 return 0; 00139 } 00140 00141 EndpointDescriptor* GetEndpoint(InterfaceDescriptor* interfaceDesc, int i) { 00142 unsigned char *d = (unsigned char*)interfaceDesc; 00143 int n = interfaceDesc->bNumEndpoints; 00144 int j = 0; 00145 if (i >= n) 00146 return 0; 00147 d += d[0];//move to first descriptor after the interface descriptor 00148 while (j < n) { 00149 switch (d[1]) { 00150 case DESCRIPTOR_TYPE_INTERFACE: 00151 case DESCRIPTOR_TYPE_CONFIGURATION: 00152 return 0; 00153 case DESCRIPTOR_TYPE_ENDPOINT: 00154 if (i == j) 00155 return (EndpointDescriptor*)d; 00156 j++; 00157 break; 00158 default: 00159 printf("Skipping descriptor %02X (%d bytes)\n",d[1],d[0]); 00160 } 00161 d += d[0]; 00162 } 00163 return 0; 00164 } 00165 00166 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc) { 00167 printf("LoadDevice %d %02X:%02X:%02X\n",device,interfaceDesc->bInterfaceClass,interfaceDesc->bInterfaceSubClass,interfaceDesc->bInterfaceProtocol); 00168 char s[128]; 00169 for (int i = 1; i < 3; i++) { 00170 if (GetString(device,i,s,sizeof(s)) < 0) 00171 break; 00172 printf("%d: %s\n",i,s); 00173 } 00174 00175 switch (interfaceDesc->bInterfaceClass) { 00176 case CLASS_MASS_STORAGE: 00177 if (interfaceDesc->bInterfaceSubClass == 0x06 && interfaceDesc->bInterfaceProtocol == 0x50) { 00178 unsigned char epin = 0x81; 00179 unsigned char epout = 0x01; 00180 for (int i = 0; i < interfaceDesc->bNumEndpoints; i++){ 00181 EndpointDescriptor* ep = GetEndpoint(interfaceDesc, i); 00182 if (ep){ 00183 if (ep->bEndpointAddress & 0x7F) 00184 if (ep->bEndpointAddress & 0x80) 00185 epin = ep->bEndpointAddress; 00186 else 00187 epout = ep->bEndpointAddress; 00188 }else break; 00189 } 00190 printf("In = %02X, Out = %02X\n", epin, epout); 00191 OnDiskInsert(device, epin, epout); // it's SCSI! 00192 } 00193 break; 00194 case CLASS_WIRELESS_CONTROLLER: 00195 if (interfaceDesc->bInterfaceSubClass == 0x01 && interfaceDesc->bInterfaceProtocol == 0x01) 00196 OnBluetoothInsert(device); // it's bluetooth! 00197 break; 00198 default: 00199 StartAutoEvent(device,1,0); 00200 break; 00201 } 00202 }
Generated on Tue Jul 12 2022 18:48:53 by
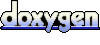