Version 1.0 - Library for Parallax OFN module 27903
Fork of OFN by
ofn.cpp
00001 00002 //@Authors: Guru Das Srinagesh, Neha Kadam 00003 /* Copyright (c) March, 2015 MIT License 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00008 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in all copies or 00012 * substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00015 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00016 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00017 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00019 */ 00020 00021 #include "ofn.h" 00022 00023 OFN::OFN(PinName sda, PinName scl): i2c(sda, scl){ 00024 i2c.frequency(400000); // Set I2C frequency to 400kHz (as per datasheet) 00025 MOTREG_ADDR = 0x02; 00026 DELTA_X_ADDR = 0x03; 00027 DELTA_Y_ADDR = 0x04; 00028 } 00029 00030 bool OFN::checkMotion(){ 00031 char tx[1], rx[1]; 00032 tx[0] = MOTREG_ADDR; 00033 i2c.write((DEVICE_ADDR<<1)&0xFE, tx, 1, true); 00034 i2c.read((DEVICE_ADDR<<1)|0x01, rx, 1); 00035 MotReg = rx[0]; 00036 00037 moved = MotReg & 0x80; 00038 00039 return moved; 00040 } 00041 00042 int OFN::readX(){ 00043 char dxAdd[1]; 00044 dxAdd[0] = DELTA_X_ADDR; 00045 if(moved){ 00046 i2c.write((DEVICE_ADDR<<1)&0xFE, dxAdd , 1, true); 00047 i2c.read((DEVICE_ADDR<<1)|0x01, raw_delta_X, 1); 00048 delta_X = (raw_delta_X[0] > 127)? raw_delta_X[0] - 255 : raw_delta_X[0]; 00049 } 00050 return delta_X; 00051 } 00052 00053 int OFN::readY(){ 00054 char dyAdd[1]; 00055 dyAdd[0] = DELTA_Y_ADDR; 00056 if(moved){ 00057 i2c.write((DEVICE_ADDR<<1)&0xFE, dyAdd, 1, true); 00058 i2c.read((DEVICE_ADDR<<1)|0x01, raw_delta_Y, 1); 00059 delta_Y = (raw_delta_Y[0] > 127)? raw_delta_Y[0] - 255 : raw_delta_Y[0]; 00060 } 00061 return delta_Y; 00062 } 00063 00064 int OFN::readOrientation(){ 00065 char tx[1], rx[1]; 00066 int orientReg; 00067 tx[0] = OFN_ORIENTATION_CTRL; 00068 i2c.write((DEVICE_ADDR<<1)&0xFE, tx, 1, true); 00069 i2c.read((DEVICE_ADDR<<1)|0x01, rx, 1); 00070 orientReg = rx[0]; 00071 return orientReg; 00072 }
Generated on Tue Jul 12 2022 19:51:47 by
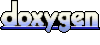