esb gcc program
Fork of esb_gcc by
Embed:
(wiki syntax)
Show/hide line numbers
nrf_esb.h
Go to the documentation of this file.
00001 /* Copyright (c) 2011 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 * $LastChangedRevision: 39629 $ 00012 */ 00013 00014 /** 00015 * @file 00016 * @brief Enhanced ShockBurst API. 00017 * 00018 */ 00019 00020 #ifndef NRF_ESB_H__ 00021 #define NRF_ESB_H__ 00022 00023 #include <stdbool.h> 00024 #include <stdint.h> 00025 #include "nrf_esb_constants.h" 00026 00027 00028 /** 00029 * @defgroup esb_02_api Application Programming Interface (API) 00030 * @{ 00031 * @ingroup modules_02_esb 00032 * @brief Enhanced ShockBurst Application Programming Interface (API). 00033 */ 00034 00035 00036 /** 00037 * @enum nrf_esb_mode_t 00038 * @brief Enumerator used for selecting the ESB mode. 00039 */ 00040 typedef enum 00041 { 00042 NRF_ESB_MODE_PTX, ///< Primary Transmitter mode 00043 NRF_ESB_MODE_PRX, ///< Primary Receiver mode 00044 } nrf_esb_mode_t; 00045 00046 /** 00047 * @enum nrf_esb_packet_t 00048 * @brief Enumerator used for selecting TX packet type used in 00049 * PTX mode. 00050 */ 00051 typedef enum 00052 { 00053 NRF_ESB_PACKET_USE_ACK, ///< PTX packet requires ACK. 00054 NRF_ESB_PACKET_NO_ACK, ///< PTX packet does not require ACK. 00055 } nrf_esb_packet_t ; 00056 00057 00058 /** 00059 * @enum nrf_esb_base_address_length_t 00060 * @brief Enumerator used for selecting the base address length. 00061 */ 00062 typedef enum 00063 { 00064 NRF_ESB_BASE_ADDRESS_LENGTH_2B, ///< 2 byte address length 00065 NRF_ESB_BASE_ADDRESS_LENGTH_3B, ///< 3 byte address length 00066 NRF_ESB_BASE_ADDRESS_LENGTH_4B ///< 4 byte address length 00067 } nrf_esb_base_address_length_t; 00068 00069 00070 /** 00071 * @enum nrf_esb_output_power_t 00072 * @brief Enumerator used for selecting the TX output power. 00073 */ 00074 typedef enum 00075 { 00076 NRF_ESB_OUTPUT_POWER_4_DBM, ///< 4 dBm output power. 00077 NRF_ESB_OUTPUT_POWER_0_DBM, ///< 0 dBm output power. 00078 NRF_ESB_OUTPUT_POWER_N4_DBM, ///< -4 dBm output power. 00079 NRF_ESB_OUTPUT_POWER_N8_DBM, ///< -8 dBm output power. 00080 NRF_ESB_OUTPUT_POWER_N12_DBM, ///< -12 dBm output power. 00081 NRF_ESB_OUTPUT_POWER_N16_DBM, ///< -16 dBm output power. 00082 NRF_ESB_OUTPUT_POWER_N20_DBM ///< -20 dBm output power. 00083 } nrf_esb_output_power_t; 00084 00085 00086 /** 00087 * @enum nrf_esb_datarate_t 00088 * @brief Enumerator used for selecting the radio data rate. 00089 */ 00090 typedef enum 00091 { 00092 NRF_ESB_DATARATE_250_KBPS, ///< 250 Kbps datarate 00093 NRF_ESB_DATARATE_1_MBPS, ///< 1 Mbps datarate 00094 NRF_ESB_DATARATE_2_MBPS, ///< 1 Mbps datarate 00095 } nrf_esb_datarate_t; 00096 00097 00098 /** 00099 * @enum nrf_esb_crc_length_t 00100 * @brief Enumerator used for selecting the CRC length. 00101 */ 00102 typedef enum 00103 { 00104 NRF_ESB_CRC_OFF, ///< CRC check disabled 00105 NRF_ESB_CRC_LENGTH_1_BYTE, ///< CRC check set to 8-bit 00106 NRF_ESB_CRC_LENGTH_2_BYTE ///< CRC check set to 16-bit 00107 } nrf_esb_crc_length_t; 00108 00109 /** 00110 * @enum nrf_esb_xosc_ctl_t 00111 * @brief Enumerator used for specifying whether switching the 00112 * external 16 MHz oscillator on/off shall be handled automatically 00113 * inside ESB or manually by the application. 00114 */ 00115 typedef enum 00116 { 00117 NRF_ESB_XOSC_CTL_AUTO, ///< Switch XOSC on/off automatically 00118 NRF_ESB_XOSC_CTL_MANUAL ///< Switch XOSC on/off manually 00119 } nrf_esb_xosc_ctl_t; 00120 00121 /******************************************************************************/ 00122 /** @name General API functions 00123 * @{ */ 00124 /******************************************************************************/ 00125 00126 /** 00127 * @brief Initialize ESB. 00128 * 00129 * @param mode The mode to initialize ESB in. 00130 * 00131 * @retval true If ESB initialized. 00132 * @retval false If ESB failed to initialize. 00133 */ 00134 bool nrf_esb_init(nrf_esb_mode_t mode); 00135 00136 00137 /** 00138 * @brief Enable ESB. 00139 * 00140 * Equivalent to setting CE high in legacy ESB. 00141 * 00142 * When enabled the behaviour described for the current ESB mode will apply. 00143 */ 00144 void nrf_esb_enable(void); 00145 00146 /** 00147 * @brief Disable ESB. 00148 * 00149 * Equivalent to setting CE low in legacy ESB. 00150 * 00151 * When calling this function ESB will begin disabling, 00152 * and will be fully disabled when ESB calls nrf_esb_disabled(). 00153 * If there are any pending notifications (callbacks) , or if any new notifications 00154 * are being added to the internal notification queue while ESB is disabling, 00155 * these will be sent to the application before ESB is fully disabled. 00156 * 00157 * After ESB has been fully disabled, no more notifications will be 00158 * sent to the application. 00159 */ 00160 void nrf_esb_disable(void); 00161 00162 /** Check whether ESB is enabled or disabled. 00163 * 00164 * @retval true If ESB is enabled. 00165 * @retval false If ESB is disabled. 00166 */ 00167 bool nrf_esb_is_enabled(void); 00168 00169 /** @} */ 00170 00171 00172 /******************************************************************************/ 00173 /** @name functions 00174 * @{ */ 00175 /******************************************************************************/ 00176 00177 /** 00178 * @brief TX success callback. 00179 * 00180 * In PTX mode this function is called after the PTX has sent a packet 00181 * and received the corresponding ACK packet from a PRX. 00182 * 00183 * In PRX mode this function is called after a payload in ACK is assumed 00184 * successfully transmitted, that is when the PRX received a new packet 00185 * (new PID or CRC) and the previous ACK sent to a PTX contained a 00186 * payload. 00187 * 00188 * @param tx_pipe The pipe on which the ACK packet was received. 00189 * 00190 * @param rssi Received signal strength indicator in dBm of measured ACK. 00191 * 00192 * As the RSSI measurement requires a minimum on-air duration of the received 00193 * packet, the measured RSSI value will not be reliable when ALL of the following 00194 * criteria are met: 00195 * 00196 * - Datarate = 2 Mbps 00197 * - Payload length = 0 00198 * - CRC is off 00199 * 00200 */ 00201 void nrf_esb_tx_success(uint32_t tx_pipe, int32_t rssi); 00202 00203 00204 /** 00205 * @brief TX failed callback (PTX mode only). 00206 * 00207 * This is called after the maximum number of TX attempts 00208 * were reached for a packet. The packet is deleted from the TX FIFO. 00209 * 00210 * Note that when NRF_ESB_PACKET_NO_ACK is used this callback is 00211 * always made after sending a packet. 00212 * @sa nrf_esb_set_max_number_of_tx_attempts(). 00213 * 00214 * @param tx_pipe The pipe that failed to send a packet. 00215 */ 00216 void nrf_esb_tx_failed(uint32_t tx_pipe); 00217 00218 00219 /** 00220 * @brief RX data ready callback. 00221 * 00222 * PTX mode: This is called after an ACK is received from a PRX containing a 00223 * payload. 00224 * 00225 * PRX mode: This is called after a packet is received from a PTX ACK is 00226 * received from a PRX containing a payload. 00227 * 00228 * @param rx_pipe is the pipe on which a packet was received. 00229 * This value must be < NRF_ESB_CONST_PIPE_COUNT. 00230 * 00231 * @param rssi Received signal strength indicator in dBm of packet. 00232 * 00233 * As the RSSI measurement requires a minimum on-air duration of the received 00234 * packet, the measured RSSI value will not be reliable when ALL of the following 00235 * criteria are met: 00236 * 00237 * - Datarate = 2 Mbps 00238 * - Payload length = 0 00239 * - CRC is off 00240 * 00241 */ 00242 void nrf_esb_rx_data_ready(uint32_t rx_pipe, int32_t rssi); 00243 00244 00245 /** 00246 * @brief Disabled callback. 00247 * 00248 * This is called after ESB enters the disabled state. 00249 * There is no further CPU use by ESB, the radio is disabled and the timer is 00250 * powered down. 00251 */ 00252 void nrf_esb_disabled(void); 00253 00254 /** @} */ 00255 00256 00257 /******************************************************************************/ 00258 /** @name Packet transmission and receiving functions 00259 * @{ */ 00260 /******************************************************************************/ 00261 00262 /** 00263 * @brief Add a packet to the tail of the TX FIFO. 00264 * 00265 * In PTX mode, the packet will be transmitted at the next occation when ESB is enabled. 00266 * In PRX mode, the payload will be piggybacked to onto an ACK. 00267 * 00268 * @param payload Pointer to the payload. 00269 * @param payload_length The number of bytes of the payload to transmit. 00270 * @param pipe The pipe for which to add the payload. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00271 * @param packet_type Specifies whether an ACK is required (ignored when in 00272 * PRX mode, or when the dynamic ack feature is disabled). 00273 * @sa nrf_esb_enable_dyn_ack() 00274 * 00275 * @retval true If the packet was successfully added to the TX FIFO. 00276 * @retval false If pipe was invalid, payload pointer was NULL, payload length 00277 was invalid, insufficient space in FIFO memory pool or 00278 insufficient packets in TX queue. 00279 */ 00280 bool nrf_esb_add_packet_to_tx_fifo(uint32_t pipe, uint8_t * payload, uint32_t payload_length, nrf_esb_packet_t packet_type); 00281 00282 00283 /** 00284 * @brief Fetch a packet from the head of the RX FIFO. 00285 * 00286 * @param payload Pointer to copy the payload to. 00287 * @param payload_length Pointer to copy the payload length to. The 00288 * payload length is given in bytes (0 to NRF_ESB_CONST_MAX_PAYLOAD_LENGTH). 00289 * @param pipe Pipe for which to add the payload. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00290 * 00291 * @retval true If the fetch was successful. 00292 * @retval false If there was no packet in the FIFO or the payload pointer 00293 * was NULL. 00294 */ 00295 bool nrf_esb_fetch_packet_from_rx_fifo(uint32_t pipe, uint8_t * payload, uint32_t* payload_length); 00296 00297 00298 /** 00299 * @brief Get the number of packets residing in the TX FIFO on a specific 00300 * pipe. 00301 * 00302 * @param pipe The pipe for which to check. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00303 * 00304 * @retval The number of packets in the TX FIFO for the pipe. 00305 */ 00306 uint32_t nrf_esb_get_tx_fifo_packet_count(uint32_t pipe); 00307 00308 00309 /** 00310 * @brief Get the number of packets residing in the RX FIFO on a specific 00311 * pipe. 00312 * 00313 * @param pipe The pipe for which to check. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00314 * 00315 * @retval The number of packets in the RX FIFO for the pipe. 00316 */ 00317 uint32_t nrf_esb_get_rx_fifo_packet_count(uint32_t pipe); 00318 00319 00320 /** 00321 * @brief Flush the RX FIFO for a specific pipe. 00322 * 00323 * Delete all the packets and free the memory of the TX FIFO for a 00324 * specific pipe. 00325 * 00326 * Note that it is not allowed to flush a TX FIFO when 00327 * ESB is enabled. 00328 * 00329 * @param pipe The pipe for which to flush. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00330 */ 00331 void nrf_esb_flush_tx_fifo(uint32_t pipe); 00332 00333 00334 /** 00335 * @brief Flush the RX FIFO for a specific pipe. 00336 * 00337 * Delete all the packets and free the memory of the RX FIFO for a 00338 * specific pipe. 00339 * 00340 * @param pipe The pipe for which to flush. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00341 */ 00342 void nrf_esb_flush_rx_fifo(uint32_t pipe); 00343 00344 00345 /** 00346 * @brief Get the total number of transmission attempts 00347 * used for sending the previous successful packet. 00348 * 00349 * The value applies to the packet for which the latest 00350 * nrf_esb_tx_data_sent callback was made. 00351 * 00352 * @return The number of transmission attempts for the 00353 * previous transmitted packet. 00354 */ 00355 uint16_t nrf_esb_get_tx_attempts(void); 00356 00357 00358 /** 00359 * @brief Specify that the previous used 2 bit packet ID (PID) shall be reused for 00360 * a given pipe. 00361 * 00362 * This function can be used for continue retransmitting a packet that previously failed 00363 * to be transmitted. 00364 00365 * Example: 00366 * 1. Upload initial packet: 00367 * nrf_esb_add_packet_to_tx_fifo(PIPE_NUMBER, my_tx_payload, TX_PAYLOAD_LENGTH, NRF_ESB_PACKET_USE_ACK); 00368 * 2. If the initial packet fails to be transmitted, specify the PID to be reused: 00369 * nrf_esb_reuse_pid(PIPE_NUMBER); 00370 * 3. Continue re-transmission of the packet by re-uploading it to the TX FIFO: 00371 * nrf_esb_add_packet_to_tx_fifo(PIPE_NUMBER, my_tx_payload, TX_PAYLOAD_LENGTH, NRF_ESB_PACKET_USE_ACK); 00372 * 00373 * @param pipe is the pipe for which to reuse the PID. 00374 */ 00375 void nrf_esb_reuse_pid(uint32_t pipe); 00376 00377 /** @} */ 00378 00379 00380 /******************************************************************************/ 00381 /** @name Configuration functions 00382 * 00383 * Configuration 'set' functions may only be called while ESB is disabled. The 00384 * new parameter comes into effect when ESB is enabled again. 00385 * 00386 * Configuration 'get' functions may be called at any time. 00387 * 00388 * @{ */ 00389 /******************************************************************************/ 00390 00391 00392 /** 00393 * @brief Set the mode. 00394 * 00395 * @param mode The mode to be used. 00396 * See nrf_esb_mode_t for a list of valid modes. 00397 * 00398 * @retval true if the mode was set properly. 00399 * @retval false if the mode is invalid. 00400 */ 00401 bool nrf_esb_set_mode(nrf_esb_mode_t mode); 00402 00403 00404 /** 00405 * @brief Get function counterpart to nrf_esb_set_mode(). 00406 * 00407 * @return The current ESB mode. 00408 */ 00409 nrf_esb_mode_t nrf_esb_get_mode(void); 00410 00411 00412 /** 00413 * @brief Set the base address length. 00414 * 00415 * @param length The base address length. 00416 * 00417 * @retval true If the address length was set. 00418 * @retval false If the length was invalid. 00419 */ 00420 bool nrf_esb_set_base_address_length(nrf_esb_base_address_length_t length); 00421 00422 00423 /** 00424 * @brief Get function counterpart to nrf_esb_set_base_address_length(). 00425 * 00426 * @return The current base_address length. 00427 */ 00428 nrf_esb_base_address_length_t nrf_esb_get_base_address_length(void); 00429 00430 00431 /** 00432 * @brief Set the base address for pipe 0. 00433 * 00434 * The full on-air address for each pipe is composed of a multi-byte base address 00435 * and a prefix address byte. 00436 * 00437 * For packets to be received correctly, the most significant byte of 00438 * the base address should not be an alternating sequence of 0s and 1s i.e. 00439 * it should not be 0x55 or 0xAA. 00440 * 00441 * @param base_address is the 4 byte base address. The parameter is 00442 * 4 bytes, however only the least L significant bytes are used, where L is 00443 * set by nrf_esb_set_base_address_length(). 00444 * 00445 * @retval true if base_address_0 was set. 00446 * @retval false if ESB was enabled. 00447 */ 00448 bool nrf_esb_set_base_address_0(uint32_t base_address); 00449 00450 00451 /** 00452 * @brief Get function counterpart to nrf_esb_set_base_address_0(). 00453 * 00454 * @return Base address 0. 00455 */ 00456 uint32_t nrf_esb_get_base_address_0(void); 00457 00458 00459 /** 00460 * @brief Set the base address for pipes 1-7. 00461 * 00462 * Pipes 1 through 7 share base_address_1. @sa nrf_esb_set_base_address_0. 00463 * 00464 * @param base_address is the 4 byte base address. The parameter is 00465 * 4 bytes, however only the least L significant bytes are used, where L is 00466 * set by nrf_esb_set_base_address_length(). 00467 * 00468 * @retval true If base_address_1 was set. 00469 * @retval false If ESB was enabled. 00470 */ 00471 bool nrf_esb_set_base_address_1(uint32_t base_address); 00472 00473 00474 /** 00475 * @brief Get function counterpart to nrf_esb_set_base_address_1(). 00476 * 00477 * @return Base address 1. 00478 */ 00479 uint32_t nrf_esb_get_base_address_1(void); 00480 00481 00482 /** 00483 * @brief Set the address prefix byte for a specific pipe. 00484 * 00485 * Each pipe should have its own unique prefix byte. 00486 * 00487 * @param pipe The pipe that the address should apply to. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00488 * @param address The address prefix byte. 00489 * 00490 * @retval true If the address prefix byte was set. 00491 * @retval false If ESB was enabled or if the pipe was invalid. 00492 */ 00493 bool nrf_esb_set_address_prefix_byte(uint32_t pipe, uint8_t address); 00494 00495 00496 /** 00497 * @brief Get function counterpart to nrf_esb_set_address_prefix_byte(). 00498 * 00499 * @param pipe the pipe for which to get the address. This value must be < NRF_ESB_CONST_PIPE_COUNT. 00500 * @param out_address is the pointer in which to return the address byte. 00501 * 00502 * @retval true If the value was set. 00503 * @retval false If ESB was enabled, or the pipe was invalid, 00504 * or the out_address pointer was NULL. 00505 */ 00506 bool nrf_esb_get_address_prefix_byte(uint32_t pipe, uint8_t* out_address); 00507 00508 00509 /** 00510 * @brief Set which pipes the node shall listen on in PRX mode. 00511 * 00512 * This value is a bitmap, and each bit corresponds to a given pipe number. 00513 * Bit 0 set to "1" enables pipes 0, bit 1 set to "1" enables pipe 1 00514 * and so forth. 00515 * The maximum number of pipes is defined by NRF_CONST_ESB_PIPE_COUNT. 00516 * 00517 * @param pipes A bitmap specifying which pipes to monitor. 00518 * 00519 * @retval true If the bitmap was set. 00520 * @retval false If ESB was enabled. 00521 */ 00522 bool nrf_esb_set_enabled_prx_pipes(uint32_t pipes); 00523 00524 00525 /** 00526 * @brief Get function counterpart to nrf_esb_set_enabled_prx_pipes(). 00527 * 00528 * @return Bitmap holding the current enabled pipes. 00529 */ 00530 uint32_t nrf_esb_get_enabled_prx_pipes(void); 00531 00532 00533 /** 00534 * @brief Set the retransmission delay. 00535 * 00536 * The retransmission delay is the delay from the start of a packet 00537 * that failed to receive the ACK until the start of the retransmission 00538 * attempt. 00539 * 00540 * The minimum value of the retransmission delay is dependent of the 00541 * radio data rate and the payload size(s).(@sa nrf_esb_set_datarate()). 00542 * As a rule of thumb, when using 32 byte payloads in each direction (forward and ACK): 00543 * 00544 * - For NRF_ESB_DATARATE_2_MBPS the retransmission delay must be >= 600 us. 00545 * - For NRF_ESB_DATARATE_1_MBPS the retransmission delay must >= 900 us. 00546 * - For NRF_ESB_DATARATE_250_KBPS the retransmission delay must be >= 2700 us. 00547 * 00548 * @param delay_us The delay in microseconds between each 00549 * retransmission attempt. 00550 * 00551 * @retval true If the retransmit delay was set. 00552 * @retval false If ESB was enabled. 00553 */ 00554 bool nrf_esb_set_retransmit_delay(uint32_t delay_us); 00555 00556 00557 /** 00558 * @brief Get function counterpart to nrf_esb_set_retransmit_delay(). 00559 * 00560 * @return The current retransmission delay. 00561 */ 00562 uint32_t nrf_esb_get_retransmit_delay(void); 00563 00564 00565 /** 00566 * @brief Set the maximum number of TX attempts 00567 * that can be used for a single packet. 00568 * 00569 * @param attempts The maximum number of TX attempts. 00570 * 0 indicates that a packet can use a infinite number of attempts. 00571 * 00572 * @retval false If ESB was enabled. 00573 */ 00574 bool nrf_esb_set_max_number_of_tx_attempts(uint16_t attempts); 00575 00576 00577 /** 00578 * @brief Get function counterpart to nrf_esb_set_max_number_of_retransmits(). 00579 * 00580 * @return The current number of maximum retransmission attempts. 00581 */ 00582 uint16_t nrf_esb_get_max_number_of_tx_attempts(void); 00583 00584 00585 /** 00586 * @brief Set the Radio Frequency (RF) channel. 00587 * 00588 * The valid channels are in the range 0 <= channel <= 125, where the 00589 * actual centre frequency is (2400 + channel) MHz. 00590 * 00591 * @param channel The RF Channel to use. 00592 * 00593 * @return false If ESB was enabled. 00594 */ 00595 bool nrf_esb_set_channel(uint32_t channel); 00596 00597 00598 /** 00599 * @brief Get function counterpart to nrf_esb_set_channel(). 00600 * 00601 * @return The current RF channel. 00602 */ 00603 uint32_t nrf_esb_get_channel(void); 00604 00605 00606 /** 00607 * @brief Set the radio TX output power. 00608 * 00609 * @param power The output power. 00610 * 00611 * @return false If the output_power was invalid. 00612 */ 00613 bool nrf_esb_set_output_power(nrf_esb_output_power_t power); 00614 00615 00616 /** 00617 * @brief Get function counterpart to nrf_esb_set_output_power(). 00618 * 00619 * @return The output power. 00620 */ 00621 nrf_esb_output_power_t nrf_esb_get_output_power(void); 00622 00623 00624 /** 00625 * @brief Set the radio datarate. 00626 * 00627 * @param datarate Datarate. 00628 * 00629 * @retval false If the datarate was invalid. 00630 */ 00631 bool nrf_esb_set_datarate(nrf_esb_datarate_t datarate); 00632 00633 00634 /** 00635 * @brief Get function counterpart to nrf_esb_set_datarate(). 00636 * 00637 * @return The current datarate. 00638 */ 00639 nrf_esb_datarate_t nrf_esb_get_datarate(void); 00640 00641 00642 /** 00643 * @brief Set the CRC length. 00644 * 00645 * The CRC length should be the same on both PTX and PRX in order 00646 * to ensure correct operation. 00647 * 00648 * @param length The CRC length. 00649 * 00650 * @retval false If ESB was enabled or the length was invalid. 00651 */ 00652 bool nrf_esb_set_crc_length(nrf_esb_crc_length_t length); 00653 00654 00655 /** 00656 * @brief Get function counterpart to nrf_esb_set_crc_length(). 00657 * 00658 * @return The current CRC length. 00659 */ 00660 nrf_esb_crc_length_t nrf_esb_get_crc_length(void); 00661 00662 00663 /** 00664 * @brief Set whether start/stop of external oscillator (XOSC) shall be handled 00665 * automatically inside ESB or manually by the application. 00666 * 00667 * When controlling the XOSC manually from the application it is 00668 * required that the XOSC is started before ESB is enabled. 00669 * 00670 * When start/stop of the XOSC is handled automatically by ESB, 00671 * the XOSC will only be running when needed, that is when the radio 00672 * is being used or when ESB needs to maintain synchronization. 00673 * 00674 * It is required that the XOSC is started in order for the radio to be 00675 * able to send or receive any packets. 00676 * 00677 * @param xosc_ctl setting for XOSC control. 00678 * 00679 * @retval true if the parameter was set. 00680 * @retval false if Gazell was enabled or the xosc_ctl value was invalid. 00681 */ 00682 bool nrf_esb_set_xosc_ctl(nrf_esb_xosc_ctl_t xosc_ctl); 00683 00684 00685 /** 00686 * @brief Enable dynamic ACK feature. After initialization this feature is disabled. 00687 * 00688 * The dynamic ACK feature must be enabled in order for the @b packet_type 00689 * parameter in the nrf_esb_add_packet_to_tx_fifo() function to have any effect, 00690 * or for the ACK bit of received packets to be evaluated. 00691 * 00692 * When the dynamic ACK feature is disabled, all packets will be ACK'ed. 00693 */ 00694 void nrf_esb_enable_dyn_ack(void); 00695 00696 /** 00697 * @brief Disable dynamic ACK feature. 00698 * 00699 * @sa nrf_esb_enable_dyn_ack() 00700 */ 00701 void nrf_esb_disable_dyn_ack(void); 00702 00703 00704 /** 00705 * Get function counterpart for nrf_esb_set_xosc_ctl(); 00706 * 00707 * @return The XOSC control setting. 00708 */ 00709 nrf_esb_xosc_ctl_t nrf_esb_get_xosc_ctl(void); 00710 00711 00712 /** @} */ 00713 /** @} */ 00714 #endif
Generated on Thu Jul 14 2022 23:58:30 by
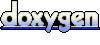