
A test utility for mma8452
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 //#include <bitset> 00003 #include "MMA8452.h" 00004 #include "helperFunctions.h" 00005 00006 // I2C i2c(p28,p27); 00007 Accelerometer_MMA8452 accelerometer(p28, p27, 40000); 00008 //Accelerometer_MMA8452 acclerometer(p28, p29); 00009 00010 Serial pc(USBTX, USBRX); 00011 DigitalOut led1(LED1); 00012 DigitalOut led2(LED2); 00013 DigitalOut led3(LED3); 00014 00015 00016 00017 int main() { 00018 led1 = 0; 00019 led2 = 0; 00020 led3 = 0; 00021 00022 char x_buffer[2]; // used to store a bit array from the x axis acclerometer 00023 char y_buffer[2]; // this represents the raw data value from the acclerometer 00024 char z_buffer[2]; // which is converted from 12bit to 16bit value 2s compliment. 00025 00026 x_buffer[0] = 0x00; 00027 x_buffer[1] = 0x00; 00028 y_buffer[0] = 0x00; 00029 y_buffer[1] = 0x00; 00030 z_buffer[0] = 0x00; 00031 z_buffer[0] = 0x00; 00032 00033 int deviceSystemMode(0); 00034 00035 // just playing with address values 00036 char mcu_address = 0x00; 00037 pc.printf("\nmcu_address is: 0x%x ", mcu_address); 00038 mcu_address = MMA8452_ADDRESS; 00039 pc.printf("\nmcu_address is: 0x%x ", mcu_address); 00040 mcu_address = (MMA8452_ADDRESS<< 1); // shifting address by 1 bit as i2c is a 7 bit encoding, and this is 8bit encoded 00041 pc.printf("\nmcu_address is now : 0x%x ", mcu_address); 00042 00043 wait(0.5); 00044 //init 00045 //set active mode 00046 pc.printf("\nWriting to master register\n"); 00047 00048 int deviceStatus = 0; 00049 if (accelerometer.get_Status(deviceStatus)==0) 00050 { 00051 pc.printf("\nGot the device Status - device status which is: 0x%x \n", deviceStatus); 00052 } 00053 else 00054 { 00055 pc.printf("\nFailed to get the device status. The id is set as: 0x%x ", deviceStatus); 00056 } 00057 00058 00059 int ControlRegister(0); 00060 if (accelerometer.get_CTRL_Reg1(ControlRegister)==0) 00061 { 00062 pc.printf("\nGot contents of Control Register1 which is: 0x%x \n", ControlRegister); 00063 00064 00065 } 00066 else 00067 { 00068 pc.printf("\nFailed to get the device status. The id is set as: 0x%x ", deviceStatus); 00069 } 00070 00071 00072 wait(5); 00073 00074 for (int i=0;i<=50;i++) 00075 { 00076 00077 if (accelerometer.activate()==0) 00078 { 00079 led1 = 1; 00080 led2 = 1; 00081 led3 = 1; 00082 pc.printf("\nActivated chip\n"); 00083 wait(0.5); 00084 00085 00086 for(int i=0; i<10;i++) 00087 { 00088 00089 /* 00090 if (accelerometer.read_x_raw(x_buffer)==0) 00091 { 00092 //pc.printf("\nThe x-axis LSB is: 0x%x", x); 00093 pc.printf("The value of my x_buffer[0] in digit form is: %d in hex: 0x%x \n",x_buffer[0],x_buffer[0]); 00094 pc.printf("The value of my x_buffer[1] in digit form is: %d in hex: 0x%x \n",x_buffer[1],x_buffer[1]); 00095 print2bytebinchar(x_buffer); 00096 00097 } 00098 else 00099 { 00100 pc.printf("\nError - could not get xaxis LSB! ....\n"); 00101 } 00102 00103 */ 00104 00105 00106 /* 00107 if (accelerometer.read_y_raw(y_buffer)==0) 00108 { 00109 //pc.printf("\nThe x-axis LSB is: 0x%x", x); 00110 pc.printf("The value of my y_buffer[0] in digit form is: %d in hex: 0x%x \n",y_buffer[0],y_buffer[0]); 00111 pc.printf("The value of my y_buffer[1] in digit form is: %d in hex: 0x%x \n",y_buffer[1],y_buffer[1]); 00112 print2bytebinchar(y_buffer); 00113 00114 } 00115 else 00116 { 00117 pc.printf("\nError - could not get yaxis LSB! ....\n"); 00118 } 00119 00120 */ 00121 00122 00123 00124 if (accelerometer.read_z_raw(z_buffer)==0) 00125 { 00126 //pc.printf("\nThe x-axis LSB is: 0x%x", x); 00127 pc.printf("The value of my z_buffer[0] in digit form is: %d in hex: 0x%x \n",z_buffer[0],z_buffer[0]); 00128 pc.printf("The value of my z_buffer[1] in digit form is: %d in hex: 0x%x \n",z_buffer[1],z_buffer[1]); 00129 print2bytebinchar(z_buffer); 00130 00131 } 00132 else 00133 { 00134 pc.printf("\nError - could not get zaxis LSB! ....\n"); 00135 } 00136 00137 00138 00139 wait(0.5); 00140 } 00141 } 00142 else 00143 { 00144 pc.printf("Could not activate the chip\n"); 00145 } 00146 00147 00148 int deviceID= 0; 00149 if (accelerometer.get_DeviceID(deviceID)==0) 00150 { 00151 pc.printf("\nGot the who am I - device id which is: 0x%x \n", deviceID); 00152 } 00153 else 00154 { 00155 pc.printf("\nFailed to get the device id. The id is set as: 0x%x ", deviceID); 00156 00157 } 00158 00159 if (accelerometer.get_Status(deviceStatus)==0) 00160 { 00161 pc.printf("\nGot the device Status - device system mode which is: 0x%x \n", deviceSystemMode); 00162 } 00163 else 00164 { 00165 pc.printf("\nFailed to get the device system mode. The id is set as: 0x%x ", deviceSystemMode); 00166 00167 } 00168 00169 wait(2); 00170 00171 pc.printf("In loop %d, of repeated initialisation.\n",i); 00172 i++; 00173 } 00174 00175 //get analog data 00176 pc.printf("Set system into standby mode........."); 00177 00178 if(accelerometer.standby()==0) 00179 { 00180 pc.printf("Device set into standby...\n"); 00181 } 00182 else 00183 { 00184 pc.printf("Device failed to set into standby...\n"); 00185 } 00186 00187 pc.printf("\nGet system mode, it should now be standby! ...\n"); 00188 if (accelerometer.get_SystemMode(deviceSystemMode)==0) 00189 { 00190 pc.printf("\nGot the device System Mode - device system mode which is: 0x%x \n", deviceSystemMode); 00191 } 00192 else 00193 { 00194 pc.printf("\nFailed to get the device system mode. The id is set as: 0x%x ", deviceSystemMode); 00195 00196 } 00197 00198 }
Generated on Mon Jul 18 2022 20:09:07 by
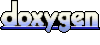