A simple yet powerful library for controlling graphical displays. Multiple display controllers are supported using inheritance.
Dependents: mbed_rifletool Hexi_Bubble_Game Hexi_Catch-the-dot_Game Hexi_Acceleromagnetic_Synth
SSD1306_SPI.cpp
00001 /* NeatGUI Library 00002 * Copyright (c) 2013 Neil Thiessen 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "SSD1306_SPI.h" 00018 00019 SSD1306_SPI::SSD1306_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dc) : Display(128, 64), m_SPI(mosi, miso, sclk), m_CS(cs), m_DC(dc) 00020 { 00021 //Deselect the display 00022 m_CS = 1; 00023 00024 //Set the SPI format to 8 bit data, high steady state clock, second edge capture 00025 m_SPI.format(8, 3); 00026 00027 //Set the SPI frequency to 10MHz 00028 m_SPI.frequency(10000000); 00029 } 00030 00031 bool SSD1306_SPI::open() 00032 { 00033 //Init sequence for 128x64 OLED module 00034 writeCommand(CMD_DISPLAYOFF); 00035 writeCommand(CMD_SETDISPLAYCLOCKDIV); 00036 writeCommand(0x80); 00037 writeCommand(CMD_SETMULTIPLEX); 00038 writeCommand(0x3F); 00039 writeCommand(CMD_SETDISPLAYOFFSET); 00040 writeCommand(0x0); 00041 writeCommand(CMD_SETSTARTLINE | 0x0); 00042 writeCommand(CMD_CHARGEPUMP); 00043 writeCommand(CMD_CHARGEPUMPON); 00044 writeCommand(CMD_MEMORYMODE); 00045 writeCommand(0x00); 00046 writeCommand(CMD_SEGREMAP | 0x1); 00047 writeCommand(CMD_COMSCANDEC); 00048 writeCommand(CMD_SETCOMPINS); 00049 writeCommand(0x12); 00050 writeCommand(CMD_SETCONTRAST); 00051 writeCommand(0xCF); 00052 writeCommand(CMD_SETPRECHARGE); 00053 writeCommand(0xF1); 00054 writeCommand(CMD_SETVCOMDETECT); 00055 writeCommand(0x40); 00056 writeCommand(CMD_DISPLAYALLON_RESUME); 00057 writeCommand(CMD_NORMALDISPLAY); 00058 00059 //Return success 00060 return true; 00061 } 00062 00063 void SSD1306_SPI::flush() 00064 { 00065 //Select low col 0, hi col 0, line 0 00066 writeCommand(CMD_SETLOWCOLUMN | 0x0); 00067 writeCommand(CMD_SETHIGHCOLUMN | 0x0); 00068 writeCommand(CMD_SETSTARTLINE | 0x0); 00069 00070 //Set DC to data and select the chip 00071 m_DC = 1; 00072 m_CS = 0; 00073 00074 //Write the entire buffer at once 00075 for (int i = 0; i < 1024; i++) 00076 m_SPI.write(m_Buffer[i]); 00077 00078 //Deselect the chip 00079 m_CS = 1; 00080 } 00081 00082 Display::State SSD1306_SPI::state() 00083 { 00084 //Return the base class's state 00085 return Display::state(); 00086 } 00087 00088 void SSD1306_SPI::state(State s) 00089 { 00090 //Check what the requested state is 00091 if (s == Display::DISPLAY_ON) { 00092 //Turn the display on 00093 writeCommand(CMD_DISPLAYON); 00094 } else if (s == Display::DISPLAY_OFF) { 00095 //Turn the display off 00096 writeCommand(CMD_DISPLAYOFF); 00097 } 00098 00099 //Update the base class 00100 Display::state(s); 00101 } 00102 00103 void SSD1306_SPI::drawPixel(int x, int y, unsigned int c) 00104 { 00105 //Range check the pixel 00106 if ((x < 0) || (x >= width()) || (y < 0) || (y >= height())) 00107 return; 00108 00109 //Make sure the color is fully opaque 00110 if ((c >> 24) != 255) 00111 return; 00112 00113 //Determine the pixel byte index 00114 unsigned short byteIndex = x + (y / 8) * width(); 00115 00116 //Set or clear the pixel 00117 if ((c & 0x00FFFFFF) > 0) 00118 m_Buffer[byteIndex] |= (1 << (y % 8)); 00119 else 00120 m_Buffer[byteIndex] &= ~(1 << (y % 8)); 00121 } 00122 00123 void SSD1306_SPI::writeCommand(char command) 00124 { 00125 //Set DC to command and select the display 00126 m_DC = 0; 00127 m_CS = 0; 00128 00129 //Write the command byte 00130 m_SPI.write(command); 00131 00132 //Deselect the display 00133 m_CS = 1; 00134 } 00135 00136 void SSD1306_SPI::writeData(char data) 00137 { 00138 //Set DC to data and select the display 00139 m_DC = 1; 00140 m_CS = 0; 00141 00142 //Write the data byte 00143 m_SPI.write(data); 00144 00145 //Deselect the display 00146 m_CS = 1; 00147 }
Generated on Tue Jul 12 2022 20:26:18 by
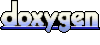