A simple yet powerful library for controlling graphical displays. Multiple display controllers are supported using inheritance.
Dependents: mbed_rifletool Hexi_Bubble_Game Hexi_Catch-the-dot_Game Hexi_Acceleromagnetic_Synth
SSD1306_I2C.h
00001 /* NeatGUI Library 00002 * Copyright (c) 2013 Neil Thiessen 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef SSD1306_I2C_H 00018 #define SSD1306_I2C_H 00019 00020 #include "mbed.h" 00021 #include "Display.h" 00022 00023 /** SSD1306_I2C class. 00024 * Used for controlling an SSD1306-based OLED display connected to i2c. 00025 */ 00026 class SSD1306_I2C : public Display 00027 { 00028 public: 00029 /** Represents the different I2C address possibilities for the SSD1306 00030 */ 00031 enum Address { 00032 ADDRESS_0 = (0x3C << 1), /**< SA0 pin = 0 */ 00033 ADDRESS_1 = (0x3D << 1), /**< SA0 pin = 1 */ 00034 }; 00035 00036 /** Create an SSD1306 object connected to the specified I2C pins with the specified I2C slave address 00037 * 00038 * @param sda The I2C data pin. 00039 * @param scl The I2C clock pin. 00040 * @param addr The I2C slave address. 00041 */ 00042 SSD1306_I2C(PinName sda, PinName scl, Address addr); 00043 00044 /** Create an SSD1306 object connected to the specified SPI pins with the specified /CS and DC pins 00045 * 00046 * @param mosi The SPI data out pin. 00047 * @param miso The SPI data in pin. 00048 * @param sclk The SPI clock pin. 00049 * @param sclk The SPI chip select pin. 00050 * @param sclk The data/command pin. 00051 */ 00052 //SSD1306_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dc); 00053 00054 /** Probe for the SSD1306 and initialize it if present 00055 * 00056 * @returns 00057 * 'true' if the device exists on the bus, 00058 * 'false' if the device doesn't exist on the bus. 00059 */ 00060 virtual bool open(); 00061 00062 /** Send the buffer to the SSD1306 00063 */ 00064 virtual void flush(); 00065 00066 /** Get the current state of the SSD1306 00067 * 00068 * @returns The current state as a Display::State enum. 00069 */ 00070 virtual Display::State state(); 00071 00072 /** Set the state of the SSD1306 00073 * 00074 * @param mode The new state as a Display::State enum. 00075 */ 00076 virtual void state(State s); 00077 00078 //void display(); 00079 00080 /** Draw a single pixel at the specified coordinates 00081 * 00082 * @param x The X coordinate. 00083 * @param y The Y coordinate. 00084 * @param c The color of the pixel as a 32-bit ARGB value. 00085 */ 00086 virtual void drawPixel(int x, int y, unsigned int c); 00087 00088 private: 00089 //Commands 00090 enum Command { 00091 CMD_SETCONTRAST = 0x81, 00092 CMD_DISPLAYALLON_RESUME = 0xA4, 00093 CMD_DISPLAYALLON = 0xA5, 00094 CMD_NORMALDISPLAY = 0xA6, 00095 CMD_INVERTDISPLAY = 0xA7, 00096 CMD_DISPLAYOFF = 0xAE, 00097 CMD_DISPLAYON = 0xAF, 00098 CMD_SETDISPLAYOFFSET = 0xD3, 00099 CMD_SETCOMPINS = 0xDA, 00100 CMD_SETVCOMDETECT = 0xDB, 00101 CMD_SETDISPLAYCLOCKDIV = 0xD5, 00102 CMD_SETPRECHARGE = 0xD9, 00103 CMD_SETMULTIPLEX = 0xA8, 00104 CMD_SETLOWCOLUMN = 0x00, 00105 CMD_SETHIGHCOLUMN = 0x10, 00106 CMD_SETSTARTLINE = 0x40, 00107 CMD_MEMORYMODE = 0x20, 00108 CMD_COMSCANINC = 0xC0, 00109 CMD_COMSCANDEC = 0xC8, 00110 CMD_SEGREMAP = 0xA0, 00111 CMD_CHARGEPUMP = 0x8D, 00112 CMD_CHARGEPUMPON = 0x14, 00113 CMD_CHARGEPUMPOFF = 0x10, 00114 CMD_ACTIVATE_SCROLL = 0x2F, 00115 CMD_DEACTIVATE_SCROLL = 0x2E, 00116 CMD_SET_VERTICAL_SCROLL_AREA = 0xA3, 00117 CMD_RIGHT_HORIZONTAL_SCROLL = 0x26, 00118 CMD_LEFT_HORIZONTAL_SCROLL = 0x27, 00119 CMD_VERTICAL_AND_RIGHT_HORIZONTAL_SCROLL = 0x29, 00120 CMD_VERTICAL_AND_LEFT_HORIZONTAL_SCROLL = 0x2A 00121 }; 00122 00123 //Control bytes for the I2C interface 00124 enum I2CControlByte { 00125 CONTROL_COMMAND = 0x00, 00126 CONTROL_DATA = 0x40 00127 }; 00128 00129 //I2C interface variables 00130 I2C m_I2C; 00131 const int m_ADDR; 00132 00133 //Back buffer 00134 char m_Buffer[1025]; 00135 00136 //Command and data helpers 00137 void writeCommand(char command); 00138 void writeData(char data); 00139 }; 00140 00141 #endif
Generated on Tue Jul 12 2022 20:26:18 by
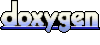