A simple yet powerful library for controlling graphical displays. Multiple display controllers are supported using inheritance.
Dependents: mbed_rifletool Hexi_Bubble_Game Hexi_Catch-the-dot_Game Hexi_Acceleromagnetic_Synth
ILI9341.h
00001 /* NeatGUI Library 00002 * Copyright (c) 2013 Neil Thiessen 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef ILI9341_H 00018 #define ILI9241_H 00019 00020 #include "mbed.h" 00021 #include "Display.h" 00022 00023 /** ILI9341 class. 00024 * Used for controlling an ILI9341-based TFT display. 00025 */ 00026 class ILI9341 : public Display 00027 { 00028 public: 00029 /** Create an ILI9341 object connected to the specified SPI pins with the specified /CS and DC pins 00030 * 00031 * @param mosi The SPI data out pin. 00032 * @param miso The SPI data in pin. 00033 * @param sclk The SPI clock pin. 00034 * @param sclk The SPI chip select pin. 00035 * @param sclk The data/command pin. 00036 */ 00037 ILI9341(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dc); 00038 00039 /** Probe for the ILI9341 and initialize it if present 00040 * 00041 * @returns 00042 * 'true' if the device exists on the bus, 00043 * 'false' if the device doesn't exist on the bus. 00044 */ 00045 virtual bool open(); 00046 00047 /** Send the buffer to the ILI9341 00048 */ 00049 virtual void flush(); 00050 00051 /** Get the current state of the ILI9341 00052 * 00053 * @returns The current state as a Display::State enum. 00054 */ 00055 virtual Display::State state(); 00056 00057 /** Set the state of the ILI9341 00058 * 00059 * @param mode The new state as a Display::State enum. 00060 */ 00061 virtual void state(State s); 00062 00063 //void display(); 00064 00065 /** Draw a single pixel at the specified coordinates 00066 * 00067 * @param x The X coordinate. 00068 * @param y The Y coordinate. 00069 * @param c The color of the pixel as a 32-bit ARGB value. 00070 */ 00071 virtual void drawPixel(int x, int y, unsigned int c); 00072 00073 private: 00074 //Commands 00075 /*enum Command { 00076 CMD_SETCONTRAST = 0x81, 00077 CMD_DISPLAYALLON_RESUME = 0xA4, 00078 CMD_DISPLAYALLON = 0xA5, 00079 CMD_NORMALDISPLAY = 0xA6, 00080 CMD_INVERTDISPLAY = 0xA7, 00081 CMD_DISPLAYOFF = 0xAE, 00082 CMD_DISPLAYON = 0xAF, 00083 CMD_SETDISPLAYOFFSET = 0xD3, 00084 CMD_SETCOMPINS = 0xDA, 00085 CMD_SETVCOMDETECT = 0xDB, 00086 CMD_SETDISPLAYCLOCKDIV = 0xD5, 00087 CMD_SETPRECHARGE = 0xD9, 00088 CMD_SETMULTIPLEX = 0xA8, 00089 CMD_SETLOWCOLUMN = 0x00, 00090 CMD_SETHIGHCOLUMN = 0x10, 00091 CMD_SETSTARTLINE = 0x40, 00092 CMD_MEMORYMODE = 0x20, 00093 CMD_COMSCANINC = 0xC0, 00094 CMD_COMSCANDEC = 0xC8, 00095 CMD_SEGREMAP = 0xA0, 00096 CMD_CHARGEPUMP = 0x8D, 00097 CMD_CHARGEPUMPON = 0x14, 00098 CMD_CHARGEPUMPOFF = 0x10, 00099 CMD_ACTIVATE_SCROLL = 0x2F, 00100 CMD_DEACTIVATE_SCROLL = 0x2E, 00101 CMD_SET_VERTICAL_SCROLL_AREA = 0xA3, 00102 CMD_RIGHT_HORIZONTAL_SCROLL = 0x26, 00103 CMD_LEFT_HORIZONTAL_SCROLL = 0x27, 00104 CMD_VERTICAL_AND_RIGHT_HORIZONTAL_SCROLL = 0x29, 00105 CMD_VERTICAL_AND_LEFT_HORIZONTAL_SCROLL = 0x2A 00106 };*/ 00107 00108 //Interface variables 00109 SPI m_SPI; 00110 DigitalOut m_CS; 00111 DigitalOut m_DC; 00112 00113 //Command and data helpers 00114 void writeCommand(char command); 00115 void writeData8(char data); 00116 void writeData16(unsigned short data); 00117 }; 00118 00119 #endif
Generated on Tue Jul 12 2022 20:26:18 by
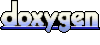