
Play battleship against another mbed over an ethernet internet connection
Dependencies: 4DGL-uLCD-SE EthernetInterface mbed-rtos mbed
Fork of Working_Get_Example_Ethernet by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include <string> 00004 #include "uLCD_4DGL.h" 00005 00006 Serial pc(USBTX,USBRX); 00007 00008 extern "C" void mbed_mac_address(char * mac) { 00009 00010 // define your own MAC Address ba:d7:05:47:1f:c6 00011 mac[0] = 0xba; 00012 mac[1] = 0xd7; 00013 mac[2] = 0x05; 00014 mac[3] = 0x47; 00015 mac[4] = 0x1f; 00016 mac[5] = 0xc6; 00017 00018 }; 00019 00020 //int main() { 00021 // pc.baud(9600); 00022 // pc.printf("Running\n"); 00023 // EthernetInterface eth; 00024 // eth.init(); //Use DHCP 00025 // wait(15); 00026 // eth.connect(); 00027 // wait(15); 00028 // pc.printf("MAC is %s\n", eth.getMACAddress()); 00029 // pc.printf("IP Address is %s\n", eth.getIPAddress()); 00030 // 00031 // TCPSocketConnection sock; 00032 // sock.connect("192.184.82.3", 5000); 00033 // 00034 // char http_cmd[300] = "GET /create_board?playerNum=1&gameNum=144&board=1111000000000000000000000000000000000000000000000000000000000000 HTTP/1.0\n\n"; 00035 // sock.send_all(http_cmd, sizeof(http_cmd)-1); 00036 // 00037 // //Instead of printing here, do what you did before to convert the string, and just append buffer to a string (below the break), 00038 // // replacing pc.printf 00039 // //Then we can do string find to snipe the values we want (have to do math to grab the right amount) 00040 // //Implemented example here, testStr now holds the received text (so you can search it) 00041 // string testStr = ""; 00042 // char buffer[600]; 00043 // int ret; 00044 // while (true) { 00045 // ret = sock.receive(buffer, sizeof(buffer)-1); 00046 // if (ret <= 0) 00047 // break; 00048 // buffer[ret] = '\0'; 00049 // pc.printf("Received %d chars from server:\n%s\n", ret, buffer); 00050 // string conv(buffer); 00051 // testStr = testStr + conv; 00052 // } 00053 // pc.printf("Stringified %s\n", testStr); 00054 // sock.close(); 00055 // wait(0.2); 00056 // sock.connect("192.184.82.3", 5000); 00057 // wait(0.2); 00058 // strcpy(http_cmd, "GET /create_board?playerNum=2&gameNum=144&board=1111000000000000000000000000000000000000000000000000000000000000 HTTP/1.0\n\n"); 00059 // pc.printf("Command is %s", http_cmd); 00060 // sock.send_all(http_cmd, sizeof(http_cmd)-1); 00061 // 00062 // while (true) { 00063 // ret = sock.receive(buffer, sizeof(buffer)-1); 00064 // if (ret <= 0) 00065 // break; 00066 // buffer[ret] = '\0'; 00067 // pc.printf("Received %d chars from server:\n%s\n", ret, buffer); 00068 // } 00069 // 00070 // sock.close(); 00071 // wait(0.2); 00072 // sock.connect("192.184.82.3", 5000); 00073 // wait(0.2); 00074 // strcpy(http_cmd, "GET /polling?gameNum=144&playerNum=1 HTTP/1.0\n\n"); 00075 // pc.printf("Command is %s", http_cmd); 00076 // sock.send_all(http_cmd, sizeof(http_cmd)-1); 00077 // 00078 // while (true) { 00079 // ret = sock.receive(buffer, sizeof(buffer)-1); 00080 // if (ret <= 0) 00081 // break; 00082 // buffer[ret] = '\0'; 00083 // pc.printf("Received %d chars from server:\n%s\n", ret, buffer); 00084 // } 00085 // 00086 // sock.close(); 00087 // wait(0.2); 00088 // sock.connect("192.184.82.3", 5000); 00089 // wait(0.2); 00090 // strcpy(http_cmd, "GET /fire?playerNum=1&gameNum=144&x=1&y=0 HTTP/1.0\n\n"); 00091 // pc.printf("Command is %s", http_cmd); 00092 // sock.send_all(http_cmd, sizeof(http_cmd)-1); 00093 // 00094 // while (true) { 00095 // ret = sock.receive(buffer, sizeof(buffer)-1); 00096 // if (ret <= 0) 00097 // break; 00098 // buffer[ret] = '\0'; 00099 // pc.printf("Received %d chars from server:\n%s\n", ret, buffer); 00100 // } 00101 // 00102 // sock.close(); 00103 // eth.disconnect(); 00104 // 00105 // while(1) {} 00106 //} 00107 00108 uLCD_4DGL lcd(p28,p27,p29); 00109 DigitalOut myled(LED1); 00110 00111 AnalogIn sliderh(p17); 00112 AnalogIn sliderv(p19); 00113 DigitalIn pb1(p21); 00114 bool down = false; 00115 string gameID = ""; 00116 string myBoard = ""; 00117 string targetBoard = ""; 00118 int size=7;//board square size in pixels 00119 int cx=0,cy=0;//cursor position 00120 int playerNum; 00121 00122 //If button is pressed, print only once 00123 bool check_button() { 00124 if(pb1 == 0 && !down) { 00125 return true; 00126 } 00127 if(pb1 == 1) { 00128 down = false; 00129 } 00130 return false; 00131 } 00132 00133 void emptyBoards() { 00134 myBoard=""; 00135 targetBoard=""; 00136 for (int i=0;i<64;i++) { 00137 myBoard+="0"; 00138 targetBoard+="0"; 00139 } 00140 } 00141 00142 //0: nothing 00143 //1: down 00144 //2: up 00145 //3: right 00146 //4: down 00147 //5: button press 00148 int analogStick() { 00149 if(sliderh < 0.2) { 00150 return 1; 00151 } 00152 else if(sliderh > 0.8) { 00153 return 2; 00154 } 00155 else if(sliderv < 0.2) { 00156 return 3; 00157 } 00158 else if(sliderv > 0.8) { 00159 return 4; 00160 } 00161 else if (check_button()){ 00162 return 5; 00163 } 00164 else 00165 return 0; 00166 } 00167 00168 string playerMenu(){ 00169 lcd.cls(); 00170 wait(0.2); 00171 //lcd.printf("Player Select:"); 00172 lcd.locate(3,0); 00173 lcd.printf("Host a Game"); 00174 lcd.locate(3, 14); 00175 lcd.printf("Join a Game"); 00176 while (1){ 00177 if (analogStick()==2){ 00178 return "1"; 00179 } 00180 else if (analogStick()==1){ 00181 return "2"; 00182 } 00183 } 00184 00185 } 00186 00187 void selectMenu(){ 00188 lcd.cls(); 00189 wait(0.2); 00190 lcd.locate(1,0); 00191 lcd.printf("Current GameID:"); 00192 int input; 00193 while (1){ 00194 input = analogStick(); 00195 if (input==5) { 00196 break; 00197 } 00198 else if (input==0) { 00199 lcd.locate(1,0); 00200 lcd.printf("Current GameID: %s",gameID); 00201 continue; 00202 } 00203 char buf[10]; 00204 sprintf(buf,"%d",input); 00205 gameID.append(buf); 00206 wait(0.3); 00207 } 00208 } 00209 00210 void drawTargetBoard(){ 00211 int x=0; 00212 int y=0; 00213 string s; 00214 for (int i=0;i<64;i++) { 00215 x=7*(i%8); 00216 if (i%8==0 && i!=0) { 00217 y+=size; 00218 } 00219 s = targetBoard.at(i); 00220 if (s.compare("0")==0){ //water 00221 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0x0000FF); 00222 } 00223 else if (s.compare("0")==1){ //unknown opponent ship 00224 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0x0000FF); 00225 } 00226 else if (s.compare("0")==2){ //hit 00227 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0xFF0000); 00228 } 00229 else if (s.compare("0")==3){ //miss 00230 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0xFFFFFF); 00231 } 00232 } 00233 } 00234 00235 void drawMyBoard(){ 00236 int x=0; 00237 int y=56+16; 00238 string s; 00239 for (int i=0;i<64;i++) { 00240 x=7*(i%8); 00241 if (i%8==0 && i!=0) { 00242 y+=size; 00243 } 00244 s = myBoard.at(i); 00245 if (s.compare("0")==0){ //water 00246 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0x0000FF); 00247 } 00248 else if (s.compare("0")==1){ //my ship 00249 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0x2F4F4F); 00250 } 00251 else if (s.compare("0")==2){ //hit 00252 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0xFF0000); 00253 } 00254 else if (s.compare("0")==3){ //miss 00255 lcd.filled_rectangle(x,y,x+size-1,y+size-1,0xFFFFFF); 00256 } 00257 } 00258 } 00259 00260 void drawLegend(){ 00261 int startX=64; 00262 int startY=16; 00263 int lSize=5; 00264 int dY=24; 00265 lcd.locate(11,2); 00266 lcd.printf("Water"); 00267 lcd.filled_rectangle(startX,startY,startX+lSize,startY+lSize,0x0000FF); 00268 lcd.locate(11,5); 00269 lcd.printf("Ship"); 00270 lcd.filled_rectangle(startX,startY+dY,startX+lSize,startY+dY+lSize,0x2F4F4F); 00271 lcd.locate(11,8); 00272 lcd.printf("Hit"); 00273 lcd.filled_rectangle(startX,startY+2*dY,startX+lSize,startY+2*dY+lSize,0xFF0000); 00274 lcd.locate(11,11); 00275 lcd.printf("Miss"); 00276 lcd.filled_rectangle(startX,startY+3*dY,startX+lSize,startY+3*dY+lSize,0xFFFFFF); 00277 } 00278 00279 void drawBoards() { 00280 lcd.cls(); 00281 //wait(0.2); 00282 drawTargetBoard(); 00283 drawMyBoard(); 00284 drawLegend(); 00285 } 00286 00287 void selectTarget(){//selected x,y coords are stored in global cx/cy coords 00288 drawBoards(); 00289 lcd.filled_rectangle(cx*size,cy*size,cx*size+size-1,cy*size+size-1,0xFFFF00); 00290 int input; 00291 while (1) { 00292 input=analogStick(); 00293 if (input==5) { 00294 return; 00295 } 00296 if (input==1) {//down 00297 if (cy<7) { 00298 cy++; 00299 } 00300 } 00301 if (input==2) {//up 00302 if (cy>0) { 00303 cy--; 00304 } 00305 } 00306 if (input==3) {//right 00307 if (cx<7) { 00308 cx++; 00309 } 00310 } 00311 if (input==4) {//left 00312 if (cx>0) { 00313 cx--; 00314 } 00315 } 00316 if (input!=0) { 00317 drawBoards(); 00318 lcd.filled_rectangle(cx*size,cy*size,cx*size+size-1,cy*size+size-1,0xFFFF00); 00319 } 00320 } 00321 } 00322 00323 void placeShip(int s){ 00324 for (int i=0;i<s;i++) { 00325 drawBoards(); 00326 lcd.filled_rectangle(cx*size,cy*size+72,cx*size+(size-1)*2,cy*size+72+size-1,0xFFFF00); 00327 int input; 00328 while (1) { 00329 input=analogStick(); 00330 if (input==5) { 00331 break; 00332 } 00333 if (input==1) {//down 00334 if (cy<7) { 00335 cy++; 00336 } 00337 } 00338 if (input==2) {//up 00339 if (cy>0) { 00340 cy--; 00341 } 00342 } 00343 if (input==3) {//right 00344 if (cx<6) { 00345 cx++; 00346 } 00347 } 00348 if (input==4) {//left 00349 if (cx>0) { 00350 cx--; 00351 } 00352 } 00353 if (input!=0) { 00354 drawBoards(); 00355 lcd.filled_rectangle(cx*size,cy*size+72,cx*size+(size-1)*2,cy*size+72+size-1,0xFFFF00); 00356 } 00357 } 00358 myBoard.replace(cy*8+cx,2,"11"); 00359 } 00360 } 00361 00362 int main() { 00363 pc.baud(9600); 00364 pc.printf("Running\n"); 00365 EthernetInterface eth; 00366 eth.init(); //Use DHCP 00367 wait(15); 00368 eth.connect(); 00369 wait(15); 00370 pc.printf("MAC is %s\n", eth.getMACAddress()); 00371 pc.printf("IP Address is %s\n", eth.getIPAddress()); 00372 TCPSocketConnection sock; 00373 char http_cmd[300]; 00374 string cmdStr = ""; 00375 string resultStr = ""; 00376 char buffer[600]; 00377 int ret; 00378 std::size_t found; 00379 00380 emptyBoards(); 00381 pb1.mode(PullUp); 00382 lcd.baudrate(3000000); 00383 lcd.cls(); 00384 //lcd.printf("%d",analogStick()); 00385 //lcd.printf("%d",playerMenu()); 00386 string pm = playerMenu(); 00387 wait(0.5); 00388 selectMenu(); 00389 lcd.cls(); 00390 placeShip(2); 00391 sock.connect("192.184.82.3", 5000); 00392 cmdStr = "GET /create_board?playerNum="; 00393 cmdStr += pm; 00394 cmdStr += "&gameNum="; 00395 cmdStr += gameID; 00396 cmdStr += "&board="; 00397 cmdStr += myBoard; 00398 cmdStr += " HTTP/1.0\n\n"; 00399 strcpy(http_cmd, cmdStr.c_str()); 00400 pc.printf(http_cmd); 00401 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00402 00403 //Instead of printing here, do what you did before to convert the string, and just append buffer to a string (below the break), 00404 // replacing pc.printf 00405 //Then we can do string find to snipe the values we want (have to do math to grab the right amount) 00406 //Implemented example here, testStr now holds the received text (so you can search it) 00407 while (true) { 00408 ret = sock.receive(buffer, sizeof(buffer)-1); 00409 if (ret <= 0) 00410 break; 00411 buffer[ret] = '\0'; 00412 string conv(buffer); 00413 resultStr = resultStr + conv; 00414 } 00415 sock.close(); 00416 resultStr=""; 00417 wait(0.2);//we have now setup and sent the board 00418 00419 while (1){ 00420 while (1){ 00421 sock.connect("192.184.82.3", 5000);//start polling to wait for turn 00422 wait(0.2); 00423 cmdStr = "GET /polling?playerNum="; 00424 cmdStr += pm; 00425 cmdStr += "&gameNum="; 00426 cmdStr += gameID; 00427 cmdStr += " HTTP/1.0\n\n"; 00428 strcpy(http_cmd, cmdStr.c_str()); 00429 pc.printf("Command is %s", http_cmd); 00430 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00431 resultStr = ""; 00432 while (true) { 00433 ret = sock.receive(buffer, sizeof(buffer)-1); 00434 if (ret <= 0) 00435 break; 00436 buffer[ret] = '\0'; 00437 string conv(buffer); 00438 resultStr = resultStr + conv; 00439 } 00440 found = resultStr.find("You lose"); 00441 pc.printf("%s",resultStr); 00442 if(found!=std::string::npos){ 00443 wait(3); 00444 sock.close(); 00445 wait(0.2); 00446 lcd.cls(); 00447 drawTargetBoard(); 00448 drawMyBoard(); 00449 lcd.locate(12,5); 00450 lcd.printf("You"); 00451 lcd.locate(12,9); 00452 lcd.printf("Lose!"); 00453 return; 00454 } 00455 found = resultStr.find("Don't go"); 00456 pc.printf("%s",resultStr); 00457 if(found==std::string::npos){ 00458 wait(3); 00459 sock.close(); 00460 wait(0.2); 00461 break; 00462 } 00463 sock.close(); 00464 wait(2); 00465 } 00466 //Now your turn 00467 // if (myBoard.at(cy*8+cx)=='1') { 00468 // targetBoard.replace(cy*8+cx,1,"2"); 00469 // myBoard.replace(cy*8+cx,1,"2"); 00470 // } 00471 // else{ 00472 // targetBoard.replace(cy*8+cx,1,"3"); 00473 // myBoard.replace(cy*8+cx,1,"3"); 00474 // } 00475 string tmp = "player"; 00476 tmp += pm; 00477 tmp += "_board"; 00478 // pc.printf("\nwe printing shit"); 00479 // pc.printf("\ntmp: %s",tmp); 00480 found = resultStr.find(tmp); 00481 pc.printf("\nfound: %d",found); 00482 myBoard = resultStr.substr(found+17,64); 00483 wait(0.5); 00484 // pc.printf("\nmyBoard: %s",myBoard); 00485 // pc.printf("\nresultStr: %s",resultStr); 00486 tmp = "player"; 00487 tmp += pm; 00488 tmp += "_board"; 00489 if (pm.compare("1")==0) 00490 tmp += "_p2"; 00491 else 00492 tmp += "_p1"; 00493 found = resultStr.find(tmp); 00494 targetBoard = resultStr.substr(found+20,64); 00495 drawBoards(); 00496 cx=0,cy=0; 00497 selectTarget(); 00498 sock.connect("192.184.82.3", 5000);//start polling to wait for turn 00499 wait(0.2); 00500 cmdStr = "GET /fire?playerNum="; 00501 cmdStr += pm; 00502 cmdStr += "&gameNum="; 00503 cmdStr += gameID; 00504 cmdStr += "&x="; 00505 char buf[10]; 00506 sprintf(buf,"%d",cx); 00507 cmdStr.append(buf); 00508 cmdStr += "&y="; 00509 char buf2[10]; 00510 sprintf(buf2,"%d",cy); 00511 cmdStr.append(buf2); 00512 cmdStr += " HTTP/1.0\n\n"; 00513 //strcpy(http_cmd, "GET /fire?playerNum=1&gameNum=144&x=1&y=0 HTTP/1.0\n\n"); 00514 strcpy(http_cmd, cmdStr.c_str()); 00515 pc.printf("Command is %s", http_cmd); 00516 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00517 resultStr = ""; 00518 while (true) { 00519 ret = sock.receive(buffer, sizeof(buffer)-1); 00520 if (ret <= 0) 00521 break; 00522 buffer[ret] = '\0'; 00523 string conv(buffer); 00524 resultStr = resultStr + conv; 00525 } 00526 found = resultStr.find("You win"); 00527 pc.printf("%s",resultStr); 00528 if(found!=std::string::npos){ 00529 wait(1.5); 00530 sock.close(); 00531 wait(0.2); 00532 lcd.cls(); 00533 drawTargetBoard(); 00534 drawMyBoard(); 00535 lcd.locate(12,5); 00536 lcd.printf("You"); 00537 lcd.locate(12,9); 00538 lcd.printf("Win!"); 00539 return; 00540 } 00541 sock.close(); 00542 wait(0.5); 00543 } 00544 00545 }
Generated on Sat Jul 30 2022 22:14:40 by
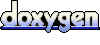