
Eurobot_2012_Secondary
Embed:
(wiki syntax)
Show/hide line numbers
RFSRF05.h
00001 00002 #ifndef MBED_RFSRF05_H 00003 #define MBED_RFSRF05_H 00004 00005 #include "mbed.h" 00006 #include "RF12B.h" 00007 00008 #define CODE0 0x22 00009 #define CODE1 0x44 00010 #define CODE2 0x88 00011 00012 /* SAMPLE IMPLEMENTATION! 00013 RFSRF05 my_srf(p13,p21,p22,p23,p24,p25,p26,p5,p6,p7,p8,p9); 00014 00015 00016 void callbinmain(int num, float dist) { 00017 //Here is where you deal with your brand new reading ;D 00018 } 00019 00020 int main() { 00021 pc.printf("Hello World of RobotSonar!\r\n"); 00022 my_srf.callbackfunc = callbinmain; 00023 00024 while (1); 00025 } 00026 00027 */ 00028 00029 class DummyCT; 00030 00031 class RFSRF05 { 00032 public: 00033 00034 RFSRF05( 00035 PinName trigger, 00036 PinName echo0, 00037 PinName echo1, 00038 PinName echo2, 00039 PinName echo3, 00040 PinName echo4, 00041 PinName echo5, 00042 PinName SDI, 00043 PinName SDO, 00044 PinName SCK, 00045 PinName NCS, 00046 PinName NIRQ); 00047 00048 /** A non-blocking function that will return the last measurement 00049 * 00050 * @returns floating point representation of distance in mm 00051 */ 00052 float read0(); 00053 float read1(); 00054 float read2(); 00055 float read(unsigned int beaconnum); 00056 00057 00058 /** A assigns a callback function when a new reading is available **/ 00059 void (*callbackfunc)(int beaconnum, float distance); 00060 DummyCT* callbackobj; 00061 void (DummyCT::*mcallbackfunc)(int beaconnum, float distance, float variance); 00062 00063 //triggers a read 00064 00065 00066 /** A short hand way of using the read function */ 00067 //operator float(); 00068 00069 private : 00070 RF12B _rf; 00071 DigitalOut _trigger; 00072 InterruptIn _echo0; 00073 InterruptIn _echo1; 00074 InterruptIn _echo2; 00075 InterruptIn _echo3; 00076 InterruptIn _echo4; 00077 InterruptIn _echo5; 00078 Timer _timer; 00079 Ticker _ticker; 00080 void _startRange(); 00081 void _rising (void); 00082 void _falling (void); 00083 float _dist[3]; 00084 char _code[3]; 00085 int _beacon_counter; 00086 bool ValidPulse; 00087 bool expValidPulse; 00088 00089 }; 00090 00091 #endif
Generated on Sat Jul 16 2022 01:26:17 by
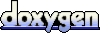