
Eurobot2012_Beacons
Embed:
(wiki syntax)
Show/hide line numbers
RF12B.h
00001 #ifndef _RF12B_H 00002 #define _RF12B_H 00003 00004 #include "mbed.h" 00005 #include <queue> 00006 00007 enum rfmode_t{RX, TX}; 00008 00009 class RF12B { 00010 public: 00011 /* Constructor */ 00012 RF12B(PinName SDI, 00013 PinName SDO, 00014 PinName SCK, 00015 PinName NCS, 00016 PinName NIRQ, 00017 PinName TRIG); 00018 00019 00020 00021 /* Reads a packet of data. Returns false if read failed. Use available() to check how much space to allocate for buffer */ 00022 bool read(unsigned char* data, unsigned int size); 00023 00024 /* Reads a byte of data from the receive buffer 00025 Returns 0xFF if there is no data */ 00026 unsigned char read(); 00027 00028 /* Transmits a packet of data */ 00029 void write(unsigned char* data, unsigned char length); 00030 void write(unsigned char data); /* 1-byte packet */ 00031 void write(queue<char> &data, int length = -1); /* sends a whole queue */ 00032 00033 /* Returns the packet length if data is available in the receive buffer, 0 otherwise*/ 00034 unsigned int available(); 00035 00036 /* RF code setting */ 00037 void setCode(unsigned char code); 00038 00039 protected: 00040 /* Receive FIFO buffer */ 00041 queue<unsigned char> fifo; 00042 00043 /* SPI module */ 00044 SPI spi; 00045 00046 /* Other digital pins */ 00047 DigitalOut NCS; 00048 InterruptIn NIRQ; 00049 DigitalIn NIRQ_in; 00050 DigitalOut rfled; 00051 DigitalOut trigLED; 00052 DigitalOut TRIG; 00053 00054 rfmode_t mode; 00055 00056 /* Initialises the RF12B module */ 00057 void init(); 00058 00059 /* Write a command to the RF Module */ 00060 unsigned int writeCmd(unsigned int cmd); 00061 00062 /* Sends a byte of data across RF */ 00063 void send(unsigned char data); 00064 00065 /* Switch module between receive and transmit modes */ 00066 void changeMode(rfmode_t mode); 00067 00068 /* Interrupt routine for data reception */ 00069 void rxISR(); 00070 00071 /* Tell the RF Module this packet is received and wait for the next */ 00072 void resetRX(); 00073 00074 /* Return the RF Module Status word */ 00075 unsigned int status(); 00076 00077 /* Calculate CRC8 */ 00078 unsigned char crc8(unsigned char crc, unsigned char data); 00079 00080 00081 unsigned char rfCode; 00082 }; 00083 00084 #endif /* _RF12B_H */
Generated on Thu Jul 14 2022 07:33:28 by
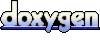