
Embedded WebSockets Experiment
Embed:
(wiki syntax)
Show/hide line numbers
HTTPRestHandler.cpp
00001 #include "HTTPRestHandler.h" 00002 #include "stdio.h" 00003 #include "TemperatureSensor.h" 00004 #include "RGBLed.h" 00005 00006 extern TemperatureSensor sensor; 00007 extern RGBLed rgb; 00008 00009 HTTPStatus HTTPRestHandler::doGet(char *resource, HTTPConnection *conn) const { 00010 printf("HTTPRestHandler GET: %s\n", resource); 00011 00012 HTTPRestData *d= new HTTPRestData(); 00013 char *host; 00014 char buffer[20]; 00015 00016 // The host field string can have junk at the end... 00017 host= (char*) conn->getField("Host"); 00018 for (char *p= host; *p != 0; p++) { 00019 if(!isprint(*p)) { 00020 *p= 0; 00021 } 00022 } 00023 00024 if (strcmp(resource, "/") == 0) { 00025 strcat(d->response, "{\"temperature\":\"http://"); 00026 strcat(d->response, host); 00027 strcat(d->response, conn->getURL()); 00028 strcat(d->response, "temperature\",\"rgbled\":\"http://"); 00029 strcat(d->response, host); 00030 strcat(d->response, conn->getURL()); 00031 strcat(d->response, "rgbled\"}"); 00032 } else if (strcmp(resource, "/temperature") == 0) { 00033 sensor.measure(); 00034 sprintf(buffer, "%f", sensor.getKelvin()); 00035 00036 strcat(d->response, "{\"realtime-uri\":\"ws://"); 00037 strcat(d->response, host); 00038 strcat(d->response, "/ws/\",\"format\":\"text/csv\",\"fields\":\"ADC,resistence,temperature\",\"calibration\":\"http:/"); 00039 strcat(d->response, conn->getURL()); 00040 strcat(d->response, "/calibration\","); 00041 strcat(d->response, "\"units\":\"kelvin\",\"value\":"); 00042 strcat(d->response, buffer); 00043 strcat(d->response, "}"); 00044 } else if (strcmp(resource, "/temperature/calibration") == 0) { 00045 strcat(d->response, "{TODO}"); 00046 } else if (strcmp(resource, "/rgbled") == 0) { 00047 strcat(d->response, "{\"web-socket-uri\":\"ws://"); 00048 strcat(d->response, host); 00049 strcat(d->response, "/ws/\",\"format\":\"text/csv\",\"fields\":\"red,green,blue\"}"); 00050 } else return HTTP_NotFound; 00051 00052 //conn->setHeaderFields("Content-Type: application/json"); 00053 conn->setLength(strlen(d->response)); 00054 conn->data= d; 00055 00056 //printf("json: [ %s ]\n", static_cast<HTTPRestData*>(conn->data)->response); 00057 return HTTP_OK; 00058 } 00059 00060 HTTPStatus HTTPRestHandler::doPost(char *resource, HTTPConnection *conn) const { 00061 printf("HTTPRestHandler POST: %s\n", resource); 00062 return HTTP_NotFound; 00063 } 00064 00065 void HTTPRestHandler::reg(HTTPServer *server) { 00066 server->registerField("Host"); 00067 } 00068 00069 HTTPStatus HTTPRestHandler::init(HTTPConnection *conn) const { 00070 char *resource= conn->getURL() + prefixLength; 00071 switch (conn->getType()) { 00072 case GET: 00073 return doGet(resource, conn); 00074 00075 case POST: 00076 return doPost(resource, conn); 00077 } 00078 return HTTP_BadRequest; 00079 } 00080 00081 00082 HTTPHandle HTTPRestHandler::data(HTTPConnection *conn, void *data, int len) const { 00083 //printf("REST data()\n"); 00084 return HTTP_SuccessEnded; 00085 } 00086 00087 HTTPHandle HTTPRestHandler::send(HTTPConnection *conn, int maxData) const { 00088 HTTPRestData *d= static_cast<HTTPRestData*>(conn->data); 00089 const char *str= d->response; 00090 int len= strlen(str); 00091 printf("REST send: %d [ %s ]\n", len, str); 00092 conn->write((void*)str, len); 00093 return HTTP_SuccessEnded; 00094 }
Generated on Wed Jul 13 2022 23:42:33 by
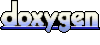