
aaa
Dependencies: mbed BNO055_fusion Adafruit_GFX ros_lib_kinetic
myOled.h
00001 #ifndef _MY_OLED_H_ 00002 #define _MY_OLED_H_ 00003 00004 #include "mbed.h" 00005 #include "Adafruit_SSD1306.h" 00006 #include "odom.h" 00007 #include "type.h" 00008 00009 class My_Oled : public Odom_Abstract, Adafruit_SSD1306_I2c 00010 { 00011 private: 00012 bool display_flag_; 00013 00014 public: 00015 My_Oled(Odom *odom, I2C &i2c) : Odom_Abstract(odom), Adafruit_SSD1306_I2c(i2c, D10){ 00016 clearDisplay(); 00017 display_flag_ = true; 00018 } 00019 00020 private: 00021 //Overlap function 00022 virtual void loop(){ 00023 static uint32_t counter = 0; 00024 if(display_flag_ == true && get_enable_oled() == false){ 00025 setTextCursor(0,0); 00026 clearDisplay(); 00027 display(); 00028 display_flag_ = false; 00029 } 00030 00031 if(get_enable_oled() && (++counter % 20) == 0){ 00032 display_flag_ = true; 00033 00034 setTextCursor(0,0); 00035 printf("Court color -> %s\n\n", (get_court_color() ? "BLUE" : "RED")); 00036 printf("odom x -> %8.4f\n", get_pose().x()); 00037 printf(" y -> %8.4f\n", get_pose().y()); 00038 printf(" a -> %8.4f\n", get_pose().z()); 00039 printf("drift x -> %8.4f\n", get_drift().x()); 00040 printf(" y -> %8.4f\n", get_drift().y()); 00041 printf(" a -> %8.4f\n", get_drift().z()); 00042 display(); 00043 } 00044 } 00045 00046 }; 00047 #endif
Generated on Mon Jul 18 2022 12:07:51 by
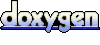