mbed I/F binding for mruby
Dependents: mruby_mbed_web mirb_mbed
object.c
00001 #include "mruby.h" 00002 #include "mruby/array.h" 00003 #include "mruby/class.h" 00004 00005 /* 00006 * call-seq: 00007 * nil.to_a -> [] 00008 * 00009 * Always returns an empty array. 00010 */ 00011 00012 static mrb_value 00013 nil_to_a(mrb_state *mrb, mrb_value obj) 00014 { 00015 return mrb_ary_new(mrb); 00016 } 00017 00018 /* 00019 * call-seq: 00020 * nil.to_f -> 0.0 00021 * 00022 * Always returns zero. 00023 */ 00024 00025 static mrb_value 00026 nil_to_f(mrb_state *mrb, mrb_value obj) 00027 { 00028 return mrb_float_value(mrb, 0.0); 00029 } 00030 00031 /* 00032 * call-seq: 00033 * nil.to_i -> 0 00034 * 00035 * Always returns zero. 00036 */ 00037 00038 static mrb_value 00039 nil_to_i(mrb_state *mrb, mrb_value obj) 00040 { 00041 return mrb_fixnum_value(0); 00042 } 00043 00044 /* 00045 * call-seq: 00046 * obj.instance_exec(arg...) {|var...| block } -> obj 00047 * 00048 * Executes the given block within the context of the receiver 00049 * (_obj_). In order to set the context, the variable +self+ is set 00050 * to _obj_ while the code is executing, giving the code access to 00051 * _obj_'s instance variables. Arguments are passed as block parameters. 00052 * 00053 * class KlassWithSecret 00054 * def initialize 00055 * @secret = 99 00056 * end 00057 * end 00058 * k = KlassWithSecret.new 00059 * k.instance_exec(5) {|x| @secret+x } #=> 104 00060 */ 00061 00062 static mrb_value 00063 mrb_obj_instance_exec(mrb_state *mrb, mrb_value self) 00064 { 00065 mrb_value *argv; 00066 mrb_int argc; 00067 mrb_value blk; 00068 struct RClass *c; 00069 00070 mrb_get_args(mrb, "*&", &argv, &argc, &blk); 00071 00072 if (mrb_nil_p(blk)) { 00073 mrb_raise(mrb, E_ARGUMENT_ERROR, "no block given"); 00074 } 00075 00076 switch (mrb_type(self)) { 00077 case MRB_TT_SYMBOL: 00078 case MRB_TT_FIXNUM: 00079 case MRB_TT_FLOAT: 00080 c = NULL; 00081 break; 00082 default: 00083 c = mrb_class_ptr(mrb_singleton_class(mrb, self)); 00084 break; 00085 } 00086 00087 return mrb_yield_with_class(mrb, blk, argc, argv, self, c); 00088 } 00089 00090 void 00091 mrb_mruby_object_ext_gem_init(mrb_state* mrb) 00092 { 00093 struct RClass * n = mrb->nil_class; 00094 00095 mrb_define_method(mrb, n, "to_a", nil_to_a, MRB_ARGS_NONE()); 00096 mrb_define_method(mrb, n, "to_f", nil_to_f, MRB_ARGS_NONE()); 00097 mrb_define_method(mrb, n, "to_i", nil_to_i, MRB_ARGS_NONE()); 00098 00099 mrb_define_method(mrb, mrb->object_class, "instance_exec", mrb_obj_instance_exec, MRB_ARGS_ANY() | MRB_ARGS_BLOCK()); 00100 } 00101 00102 void 00103 mrb_mruby_object_ext_gem_final(mrb_state* mrb) 00104 { 00105 } 00106
Generated on Tue Jul 12 2022 18:00:34 by
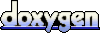