SDFileSystem, slightly modified for the ExoController
Dependents: Data-Management-Honka
Fork of SDFileSystem_HelloWorld by
SDFile.h
00001 /** 00002 * @file SDFile.h 00003 * @brief This header file contains read/write functions for the SDFile object 00004 * @author Michael Ling 00005 * @date 2/2/2015 00006 */ 00007 00008 #include <string> 00009 00010 #include "mbed.h" 00011 #include "SDFileSystem.h" 00012 00013 #ifndef SDFILE_H 00014 #define SDFILE_H 00015 class SDFile 00016 { 00017 public: 00018 SDFile(std::string path, std::string filename, bool readOnly); 00019 int *read(int length, int *array); 00020 int *read_from_start(int length, int *array); 00021 void write(int length, int *array); 00022 void write_to_index(int index, int value); 00023 void append(int length, int *array); 00024 // void changeAccess(std::string path, std::string filename); 00025 void open_for_read(std::string path, std::string filename); 00026 void open_for_write(std::string path, std::string filename); 00027 void close(); 00028 void debug_print(); 00029 00030 private: 00031 FILE *_fp; 00032 }; 00033 #endif
Generated on Tue Jul 19 2022 21:06:39 by
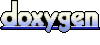