
2 Player Tron
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed-rtos mbed wave_player
Fork of 2PlayerTron by
main.cpp
00001 00002 // uLCD-144-G2 demo program for uLCD-4GL LCD driver library 00003 // 00004 #include "mbed.h" 00005 #include "uLCD_4DGL.h" 00006 #include "rtos.h" 00007 #include "SDFileSystem.h" 00008 #include "wave_player.h" 00009 00010 uLCD_4DGL uLCD(p9,p10,p8); // serial tx, serial rx, reset pin; 00011 BusIn joy1(p11,p12,p13,p14,p15); 00012 BusIn joy2(p16,p17,p19,p20,p21); 00013 00014 int player1_direction = 1; 00015 int player2_direction = 2; 00016 bool hitClick; 00017 bool play1 = false; 00018 int choice = 1; 00019 FILE *wave_file; 00020 00021 SDFileSystem sd(p5, p6, p7, p27, "sd"); //SD card 00022 AnalogOut DACout(p18); 00023 wave_player waver(&DACout); 00024 00025 // Thread 1 00026 // Joystick 1 00027 void joy1_thread(void const *args) { 00028 while(true) { 00029 switch(joy1) { 00030 case 0x1e: 00031 if (player1_direction != 4) { 00032 player1_direction = 3; 00033 } 00034 break; 00035 case 0x1d: hitClick = true; break; 00036 case 0x1b: 00037 if (player1_direction != 1) { 00038 player1_direction = 2; 00039 } 00040 break; 00041 case 0x17: 00042 if (player1_direction != 3) { 00043 player1_direction = 4; 00044 } 00045 break; 00046 case 0xf: 00047 if (player1_direction != 2) { 00048 player1_direction = 1; 00049 } 00050 break; 00051 } 00052 00053 Thread::wait(50); 00054 } 00055 } 00056 00057 // Thread 2 00058 // Joystick 2 00059 void joy2_thread(void const *args) { 00060 while(true) { 00061 switch(joy2) { 00062 case 0x1e: 00063 if (player2_direction != 4) { 00064 player2_direction = 3; 00065 } 00066 break; 00067 case 0x1d: hitClick = true; break; 00068 case 0x1b: 00069 if (player2_direction != 1) { 00070 player2_direction = 2; 00071 } 00072 break; 00073 case 0x17: 00074 if (player2_direction != 3) { 00075 player2_direction = 4; 00076 } 00077 break; 00078 case 0xf: 00079 if (player2_direction != 2) { 00080 player2_direction = 1; 00081 } 00082 break; 00083 } 00084 00085 Thread::wait(50); 00086 } 00087 } 00088 00089 int main() 00090 { 00091 Thread thread(joy1_thread); 00092 Thread thread2(joy2_thread); 00093 00094 00095 while (true) { 00096 uLCD.filled_rectangle(0, 0, 128, 128, 0x000000); 00097 uLCD.text_width(4); //4X size text 00098 uLCD.text_height(4); 00099 uLCD.color(RED); 00100 00101 00102 wave_file=fopen("/sd/wavfiles/dp_tron_start.wav","r"); 00103 waver.play(wave_file); 00104 fclose(wave_file); 00105 00106 for (int i=4; i>=0; --i) { 00107 uLCD.locate(1.9,1.9); 00108 uLCD.printf("%d",i); 00109 Thread::wait(400); 00110 } 00111 uLCD.cls(); 00112 00113 player1_direction = 1; 00114 player2_direction = 2; 00115 int velocity = 2; 00116 int p1x = 50; 00117 int p1y = 50; 00118 int p2x = 100; 00119 int p2y = 100; 00120 int size = 2; 00121 int vel = 2; 00122 int count = 0; 00123 int p1xH[1000]; 00124 int p1yH[1000]; 00125 int p2xH[1000]; 00126 int p2yH[1000]; 00127 int lost_player = 0; 00128 00129 00130 00131 bool game = true; 00132 uLCD.filled_rectangle(0, 0, 128, 128, 0x000000); 00133 //draw walls 00134 uLCD.line(0, 0, 127, 0, WHITE); 00135 uLCD.line(127, 0, 127, 127, WHITE); 00136 uLCD.line(127, 127, 0, 127, WHITE); 00137 uLCD.line(0, 127, 0, 0, WHITE); 00138 00139 uLCD.filled_rectangle(p1x, p1y, p1x + size, p1y + size, 0xFF0000); 00140 uLCD.filled_rectangle(p2x, p2y, p2x + size, p2y + size, 0x0000FF); 00141 00142 while(game) { 00143 // check the previous paths 00144 p1xH[count] = p1x; 00145 p1yH[count] = p1y; 00146 p2xH[count] = p2x; 00147 p2yH[count] = p2y; 00148 00149 // Movement for Player 1 00150 if (player1_direction == 1) { // moving right 00151 if (p1x >= 126 - size) { 00152 lost_player = 1; 00153 game = false; 00154 } 00155 p1x = p1x + vel; 00156 00157 } else if (player1_direction == 2) { // moving left 00158 if (p1x <= size + 1) { 00159 lost_player = 1; 00160 game = false; 00161 } 00162 p1x = p1x - vel; 00163 00164 } else if (player1_direction == 3) { // moving up 00165 if (p1y <= size + 1) { 00166 lost_player = 1; 00167 game = false; 00168 } 00169 p1y = p1y - vel; 00170 00171 } else if (player1_direction == 4) { // moving down 00172 if (p1y >= 126 - size) { 00173 lost_player = 1; 00174 game = false; 00175 } 00176 p1y = p1y + vel; 00177 } 00178 00179 00180 // Movement for Player 2 00181 if (player2_direction == 1) { // moving right 00182 if (p2x >= 126 - size) { 00183 lost_player = 2; 00184 game = false; 00185 } 00186 p2x = p2x + vel; 00187 } else if (player2_direction == 2) { // moving left 00188 if (p2x <= size + 1) { 00189 lost_player = 2; 00190 game = false; 00191 } 00192 p2x = p2x - vel; 00193 } else if (player2_direction == 3) { // moving up 00194 if (p2y <= size + 1) { 00195 lost_player = 2; 00196 game = false; 00197 } 00198 p2y = p2y - vel; 00199 00200 } else if (player2_direction == 4) { // moving down 00201 if (p2y >= 126 - size) { 00202 lost_player = 2; 00203 game = false; 00204 } 00205 p2y = p2y + vel; 00206 } 00207 00208 //collision check 00209 bool found = false; 00210 for (int i=0; (i < count+1 && !found); i++) { 00211 // for player 1 00212 if ((p2xH[i] > (p1x + size)) || ((p2xH[i] + size) < p1x) || ((p2yH[i] + size) < p1y) || (p2yH[i] > (p1y + size))) { 00213 00214 } else { 00215 found = true; 00216 game = false; 00217 lost_player = 1; 00218 } 00219 00220 if (i > 0) { 00221 int j = i - 1; 00222 if ((p1xH[j] >= (p1x + size)) || ((p1xH[j] + size) <= p1x) || ((p1yH[j] + size) <= p1y) || (p1yH[j] >= (p1y + size))) { 00223 00224 } else { 00225 found = true; 00226 game = false; 00227 lost_player = 1; 00228 } 00229 } 00230 00231 // for player 2 00232 if ((p1xH[i] > (p2x + size)) || ((p1xH[i] + size) < p2x) || ((p1yH[i] + size) < p2y) || (p1yH[i] > (p2y + size))) { 00233 //test passed 00234 } else { 00235 found = true; 00236 game = false; 00237 lost_player = 2; 00238 } 00239 00240 if (i > 0) { 00241 int j = i - 1; 00242 if ((p2xH[j] >= (p2x + size)) || ((p2xH[j] + size) <= p2x) || ((p2yH[j] + size) <= p2y) || (p2yH[j] >= (p2y + size))) { 00243 00244 } else { 00245 found = true; 00246 game = false; 00247 lost_player = 2; 00248 } 00249 } 00250 } 00251 00252 00253 //Drawing the path. 00254 uLCD.filled_rectangle(p1x, p1y, p1x + size, p1y + size, 0xFF0000); 00255 uLCD.filled_rectangle(p2x, p2y, p2x + size, p2y + size, 0x0000FF); 00256 00257 00258 00259 if (!game) { 00260 uLCD.filled_rectangle(0, 0, 128, 128, 0x000000); 00261 choice = 2; 00262 play1 = true; 00263 uLCD.locate(5,2); 00264 uLCD.printf("Game over"); 00265 uLCD.locate(3,4); 00266 uLCD.printf("Player %d LOST", lost_player); 00267 uLCD.locate(2,9); 00268 uLCD.color(GREEN); 00269 uLCD.printf("Click to start!"); 00270 wave_file=fopen("/sd/wavfiles/dp_tron_end.wav","r"); 00271 waver.play(wave_file); 00272 fclose(wave_file); 00273 hitClick = false; 00274 while (!hitClick) { 00275 Thread::wait(300); 00276 } 00277 } 00278 count = count + 1; 00279 Thread::wait(30); 00280 } 00281 00282 } 00283 /*if ((x<=radius+1) || (x>=126-radius)) vx = -.90*vx; 00284 if ((y<=radius+1) || (y>=126-radius)) vy = -.90*vy;*/ 00285 //......more code demos can be found in main.cpp 00286 }
Generated on Wed Jul 20 2022 02:01:06 by
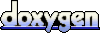