ks0713 SPI library. This library includes a small 5x5 ascii font and line function.
Embed:
(wiki syntax)
Show/hide line numbers
ks0713_spi.cpp
00001 #include "mbed.h" 00002 #include "font5x5.h" 00003 #include "ks0713_spi.h" 00004 00005 KS0713_SPI::KS0713_SPI(PinName mosi, PinName sclk, PinName cs1b, PinName rs, PinName rstb) 00006 : _spi(mosi,NC,sclk), _cs1b(cs1b), _rs(rs), _rstb(rstb) { 00007 init(); 00008 } 00009 00010 00011 void KS0713_SPI::init() { 00012 _cs1b=0; 00013 _rs =0; 00014 _rstb=0; 00015 00016 _spi.format(8,0); 00017 _spi.frequency(5000000); 00018 00019 00020 wait(0.015); // wait for power up (reset low term must be greater than 900[ns]) 00021 00022 _rstb=1; // de-assert resetb 00023 00024 00025 writeCommand(0xA2); // LCD BIAS selection (1/65duty, 1/9bias) 00026 writeCommand(0xA1); // ADC selection (SEG128->SEG0) 00027 writeCommand(0xC0); // SHL selection (COM0->COM64) 00028 wait_ms(1); 00029 00030 /*Power control 00031 Displaytech screen uses built-in power circuits 00032 Switch on in order as specified in data sheet 00033 wait 1ms between each command 00034 1st - Voltage converter ON = 0x2C 00035 2nd - Voltage regulator ON = 0x2E 00036 3rd - Voltage follower ON = 0x2F */ 00037 writeCommand(0x2C); //0x2C = Voltage converter ON 00038 wait_ms(1); 00039 writeCommand(0x2E); //0x2E = Voltage regulator ON 00040 wait_ms(1); 00041 writeCommand(0x2F); //0x2F = Voltage follower ON 00042 00043 00044 // Regulator Resistor Selection 00045 /*Regulator resistor select 00046 Sets the internal resistance ratio used in the internal voltage regulator 00047 Refer to datasheet p.42 00048 This works as a corse contrast control 00049 0x20 = 1.9 00050 0x21 = 2.19 00051 0x22 = 2.55 00052 0x23 = 3.02 00053 0x24 = 3.61 00054 0x25 = 4.35 00055 0x26 = 5.29 00056 0x27 = 6.48 */ 00057 writeCommand(0x23); 00058 00059 /*Set reference voltage register 00060 Used as a fine contrast control 00061 0x81 = Enter voltage register set mode 00062 0x00 to 0x3F = 0 to 63 */ 00063 writeCommand(0x81); //0x81 = Enter voltage register set mode 00064 writeCommand(0x20); //0x20 = Set ref voltage to 20 00065 00066 /*Initial display line 00067 Specify DDRAM line for COM1 00068 0x40 + display line */ 00069 writeCommand(0x40); //Set initial line to 0 00070 00071 /*Set page address 00072 Sets the initial page address to write to 00073 0xB0 + page address 0 to 8 */ 00074 writeCommand(0xB0); //Initial page set to 0 00075 00076 /*Set column address 00077 Sets the initial column to write to 00078 for LSB (b3-b0) 0x00 + first nibble 00079 for MSB (b7-b4) 0x10 + second nibble 00080 0x00 to 0x83 = column 0 to 131 */ 00081 writeCommand(0x00); //Sets LSB to 0 00082 writeCommand(0x10); //Sets MSB to 0 - column is now set to 0 00083 00084 /*Reverse display 00085 Selects either a normal display or a reverse display 00086 0xA6 = normal 00087 0xA7 = reverse */ 00088 writeCommand(0xA6); //Sets display to normal 00089 00090 /*Set static indicator 00091 Sets up a static indicator on the display 00092 See datasheet p.42 00093 This is a 2 instruction cycle 00094 0xAC = static indicator ON 00095 0xAD = static indicator OFF 00096 Next instruction to set indicator type: 00097 0x00 = OFF 00098 0x01 = ON - 1 second blinking 00099 0x02 = ON - 0.5 second blinking 00100 0x03 = ON - always ON */ 00101 writeCommand(0xAD); //Static indicator OFF 00102 writeCommand(0x00); //OFF - 0.5 second blinking 00103 00104 /*Display ON/OFF 00105 Switched the display to on or off 00106 0xAE = Display OFF 00107 0xAF = Display ON */ 00108 writeCommand(0xAF); 00109 00110 clear(); 00111 update(); 00112 00113 _x=0; 00114 _y=0; 00115 } 00116 00117 void KS0713_SPI::writeCommand(unsigned char data) { 00118 _cs1b=0; 00119 _rs =0; 00120 wait_us(1); // setup 55[ns] 00121 _spi.write(data); 00122 wait_us(1); // hold 180[ns] 00123 _cs1b=1; 00124 } 00125 00126 void KS0713_SPI::writeData(unsigned char data) { 00127 _cs1b=0; 00128 _rs =1; 00129 wait_us(1); // setup 55[ns] 00130 _spi.write(data); 00131 wait_us(1); // hold 180[ns] 00132 _cs1b=1; 00133 } 00134 00135 void KS0713_SPI::clear() { 00136 for (int page=0; page<MBED_KS0713_SPI_HEIGHT/8; page++) { 00137 for (int column=0; column<MBED_KS0713_SPI_WIDTH; column++) { 00138 vram[column][page]=0x00; 00139 } 00140 } 00141 } 00142 void KS0713_SPI::fill() { 00143 for (int page=0; page<MBED_KS0713_SPI_HEIGHT/8; page++) { 00144 for (int column=0; column<MBED_KS0713_SPI_WIDTH; column++) { 00145 vram[column][page]=0xFF; 00146 } 00147 } 00148 } 00149 void KS0713_SPI::update() { 00150 for (int page=0; page<MBED_KS0713_SPI_HEIGHT/8; page++) { 00151 writeCommand(0xB0+page); 00152 writeCommand(0x10); 00153 writeCommand(0x00); 00154 for (int column=0; column<100; column++) { 00155 writeData(vram[column][page]); 00156 } 00157 } 00158 } 00159 00160 void KS0713_SPI::locate(int x,int y) { 00161 _x=x; 00162 _y=y; 00163 } 00164 00165 void KS0713_SPI::line(int x1, int y1, int x2, int y2) { 00166 int dist_x; 00167 int dist_y; 00168 int calc_point; 00169 00170 if (x2>x1) { 00171 dist_x = x2-x1; 00172 } else { 00173 dist_x = x1-x2; 00174 } 00175 if (y2>y1) { 00176 dist_y = y2-y1; 00177 } else { 00178 dist_y = y1-y2; 00179 } 00180 00181 00182 00183 if (dist_x>dist_y) { 00184 // x direction 00185 if (x1>x2) 00186 // step -1 00187 { 00188 for (int x=x1; x>=x2; x--) { 00189 if (x<0) 00190 break; 00191 calc_point = y1+ ( (y2-y1) * (x-x1) / (x2-x1) ); 00192 if (calc_point>=0 && calc_point<MBED_KS0713_SPI_HEIGHT) 00193 vram[x][(calc_point>>3)] |= ( 0x1 <<(calc_point&0x7)); 00194 } 00195 } else 00196 // step ++ 00197 { 00198 // point 1 -> point 2 00199 for (int x=x1; x<=x2; x++) { 00200 if (x>=MBED_KS0713_SPI_WIDTH) 00201 break; 00202 calc_point = y1+( (y2-y1) * (x-x1) / (x2-x1) ); 00203 if (calc_point>=0 && calc_point<MBED_KS0713_SPI_HEIGHT) 00204 vram[x][(calc_point>>3)] |= ( 0x1 <<(calc_point&0x7)); 00205 } 00206 00207 } 00208 } else { 00209 // y direction 00210 if (y1>y2) 00211 // step -1 00212 { 00213 for (int y=y1; y>=y2; y--) { 00214 if (y<0) 00215 break; 00216 calc_point = x1+( (x2-x1) * (y-y1) / (y2-y1) ); 00217 if (calc_point>=0 && calc_point<MBED_KS0713_SPI_WIDTH) 00218 vram[calc_point][y>>3] |= ( 0x1 <<(y&0x7)); 00219 } 00220 } else 00221 // step ++ 00222 { 00223 // point 1 -> point 2 00224 for (int y=y1; y<=y2; y++) { 00225 if (y>=MBED_KS0713_SPI_HEIGHT) 00226 break; 00227 calc_point = x1+( (x2-x1) * (y-y1) / (y2-y1) ); 00228 if (calc_point>=0 && calc_point<MBED_KS0713_SPI_WIDTH) 00229 vram[calc_point][y>>3] |= ( 0x1 <<(y&0x7)); 00230 } 00231 } 00232 } 00233 } 00234 00235 00236 int KS0713_SPI::_putc(int value) { 00237 unsigned char fontdata; 00238 for (int cx=0; cx<5; cx++) { 00239 if ( _x+cx>=MBED_KS0713_SPI_WIDTH ) { 00240 break; 00241 } 00242 00243 fontdata = font5x5[value-' '][cx]; 00244 for (int cy=0; cy<5; cy++) { 00245 if (_y+cy>=MBED_KS0713_SPI_HEIGHT) { 00246 break ; 00247 } 00248 00249 if (fontdata & 0x1) { 00250 vram[_x+cx][(_y+cy)>>3] |= ( 0x1 <<((_y+cy)&0x7)); 00251 } else { 00252 vram[_x+cx][(_y+cy)>>3] &= ~( 0x1 <<((_y+cy)&0x7)); 00253 00254 } 00255 fontdata=fontdata>>1; 00256 } 00257 } 00258 00259 _x+=6; 00260 return value; 00261 } 00262 00263 int KS0713_SPI::_getc() { 00264 return -1; 00265 }
Generated on Wed Aug 10 2022 09:30:48 by
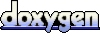