PING Library
Embed:
(wiki syntax)
Show/hide line numbers
PING.h
00001 #ifndef MBED_PING_H 00002 #define MBED_PING_H 00003 #include "mbed.h" 00004 00005 /* Class: PING 00006 * Abstraction for the PING Ultrasonic range finder 00007 * 00008 * Example: 00009 * > // Print measured distance 00010 * > 00011 * > #include "mbed.h" 00012 * > #include "PING.h" 00013 * > 00014 * > PING ping(p9); 00015 * > 00016 * > int main() { 00017 * > while(1) { 00018 * > printf("Measured : %.1f\n", ping.read()); 00019 * > wait(0.2); 00020 * > } 00021 * > } 00022 */ 00023 class PING 00024 { 00025 // Public functions 00026 public: 00027 /* Constructor: PING 00028 * Create a PING object, connected to the specified pins 00029 * 00030 * Variables: 00031 * trigger - Output to trigger the PING 00032 */ 00033 PING(PinName trigger); 00034 00035 /* Function: read 00036 * A non-blocking function that will return the last measurement 00037 * 00038 * Variables: 00039 * returns - floating point representation in cm. 00040 */ 00041 float read(void); 00042 00043 /* Function: read_in 00044 * A non-blocking function that will return the last measurement 00045 * 00046 * Variables: 00047 * returns - floating point representation in in. 00048 */ 00049 float read_in(void); 00050 00051 /* Function: operator float 00052 * A short hand way of using the read function 00053 * 00054 * Example: 00055 * > float range = ping.read(); 00056 * > float range = ping; 00057 * > 00058 * > if(ping.read() > 0.25) { ... } 00059 * > if(ping > 0.25) { ... } 00060 */ 00061 operator float(); 00062 00063 private : 00064 DigitalInOut _trigger; 00065 Timer _timer; 00066 Ticker _ticker; 00067 void _startRange(void); 00068 float _dist; 00069 }; 00070 00071 #endif
Generated on Fri Jul 15 2022 02:34:19 by
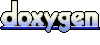