A library with drivers for different peripherals on the LPC4088 QuickStart Board or related add-on boards.
Fork of EALib by
TouchPanel.h
00001 /* 00002 * Copyright 2013 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef TOUCHPANEL_H 00018 #define TOUCHPANEL_H 00019 00020 00021 /** 00022 * An abstract class that represents touch panels. 00023 */ 00024 class TouchPanel { 00025 public: 00026 00027 typedef struct { 00028 int32_t x; 00029 int32_t y; 00030 int32_t z; 00031 } touchCoordinate_t; 00032 00033 00034 /** 00035 * Initialize the touch controller. This method must be called before 00036 * calibrating or reading data from the controller 00037 * 00038 * @param width the width of the touch panel. This is usually the same as 00039 * the width of the display 00040 * @param height the height of the touch panel. This is usually the same 00041 * as the height of the display. 00042 * 00043 * @return true if the request was successful; otherwise false 00044 */ 00045 virtual bool init(uint16_t width, uint16_t height) = 0; 00046 00047 /** 00048 * Read coordinates from the touch panel. 00049 * 00050 * @param coord pointer to coordinate object. The read coordinates will be 00051 * written to this object. 00052 */ 00053 virtual bool read(touchCoordinate_t &coord) = 0; 00054 00055 00056 /** 00057 * Start to calibrate the display 00058 * 00059 * @return true if the request was successful; otherwise false 00060 */ 00061 virtual bool calibrateStart() = 0; 00062 00063 /** 00064 * Get the next calibration point. Draw an indicator on the screen 00065 * at the coordinates and ask the user to press/click on the indicator. 00066 * Please note that waitForCalibratePoint() must be called after this 00067 * method. 00068 * 00069 * @param x the x coordinate is written to this argument 00070 * @param y the y coordinate is written to this argument 00071 * 00072 * @return true if the request was successful; otherwise false 00073 */ 00074 virtual bool getNextCalibratePoint(uint16_t* x, uint16_t* y) = 0; 00075 00076 /** 00077 * Wait for a calibration point to have been pressed and recored. 00078 * This method must be called just after getNextCalibratePoint(). 00079 * 00080 * @param morePoints true is written to this argument if there 00081 * are more calibrations points available; otherwise it will be false 00082 * @param timeout maximum number of milliseconds to wait for 00083 * a calibration point. Set this argument to 0 to wait indefinite. 00084 * 00085 * @return true if the request was successful; otherwise false 00086 */ 00087 virtual bool waitForCalibratePoint(bool* morePoints, uint32_t timeout) = 0; 00088 00089 00090 }; 00091 00092 #endif
Generated on Wed Jul 13 2022 02:29:31 by
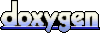