A library with drivers for different peripherals on the LPC4088 QuickStart Board or related add-on boards.
Fork of EALib by
EaLcdBoard.h
00001 /* 00002 * Copyright 2013 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef EALCDBOARD_H 00018 #define EALCDBOARD_H 00019 00020 #include "LcdController.h" 00021 00022 /** An interface to Embedded Artists LCD Boards 00023 * 00024 */ 00025 class EaLcdBoard { 00026 public: 00027 00028 enum Result { 00029 Ok = 0, 00030 InvalidCommandString, 00031 InvalidArgument, 00032 InvalidStorage, 00033 BufferTooSmall, 00034 VersionNotSupported, 00035 LcdAccessError 00036 }; 00037 00038 enum Constants { 00039 NameBufferSize = 30 00040 }; 00041 00042 enum TouchPanelId { 00043 // must be first 00044 TouchPanelInvalidFirst = 0x80, 00045 00046 // Texas Instruments Touch Screen Controller (TSC2046). 00047 TouchPanel_TSC2046, 00048 // Microchip Touch Screen Controller (AR1021). 00049 TouchPanel_AR1021, 00050 00051 // Must be after last panel ID 00052 TouchPanelInvalidLast, 00053 // unknown touch panel 00054 TouchPanelUnknown = 0xFF 00055 00056 }; 00057 00058 typedef struct { 00059 uint8_t swap; // set to 1 if x and y should be swapped 00060 uint32_t xres; // x resistance 00061 uint32_t yres; // y resistance 00062 TouchPanelId panelId; // identifies touch panel 00063 00064 } TouchParams_t; 00065 00066 /** Create an interface to an Embedded Artists LCD Board 00067 * 00068 * @param sda I2C data line pin 00069 * @param scl I2C clock line pin 00070 */ 00071 EaLcdBoard(PinName sda, PinName scl); 00072 00073 /** Open the interface and start initialization. 00074 * 00075 * @param cfg initialize with a given LCD configuration. If this argument is 00076 * NULL the LCD configuration will be retrieved from persistent 00077 * storage on the LCD Board. 00078 * @param initSeq the initialization string. If this argument is NULL the 00079 * initialization string will be retrieved from persistent 00080 * storage on the LCD Board. 00081 * 00082 * @returns the result of the operation 00083 */ 00084 Result open(LcdController::Config* cfg, char* initSeq); 00085 00086 /** Close the interface 00087 * 00088 * @returns the result of the operation 00089 */ 00090 Result close(); 00091 00092 /** Set and activate the address of the frame buffer to use. 00093 * 00094 * It is the content of the frame buffer that is shown on the 00095 * display. All the drawing on the frame buffer can be done 00096 * 'offline' and whenever it should be shown this function 00097 * can be called with the address of the offline frame buffer. 00098 * 00099 * @param address Memory address of the frame buffer 00100 * 00101 * @returns the result of the operation 00102 */ 00103 Result setFrameBuffer(uint32_t address); 00104 00105 /** Get the LCD configuration stored in persistent storage on the LCD Board 00106 * 00107 * @param cfg pointer to a configuration object. Parameters are copied to 00108 * this object. 00109 * 00110 * @returns the result of the operation 00111 */ 00112 Result getLcdConfig(LcdController::Config* cfg); 00113 00114 /** Get the display name stored in persistent storage on the LCD Board 00115 * 00116 * @param buf buffer to which the name will be copied 00117 * @param len size of the buffer in bytes 00118 * 00119 * @returns the result of the operation 00120 */ 00121 Result getDisplayName(char* buf, int len); 00122 00123 /** Get the display manufacturer stored in persistent storage on the 00124 * LCD Board 00125 * 00126 * @param buf buffer to which the name will be copied 00127 * @param len size of the buffer in bytes 00128 * 00129 * @returns the result of the operation 00130 */ 00131 Result getDisplayMfg(char* buf, int len); 00132 00133 /** Get the initialization sequence stored in persistent storage on the 00134 * LCD Board 00135 * 00136 * @param buf buffer to which the string will be copied 00137 * @param len size of the buffer in bytes 00138 * 00139 * @returns the result of the operation 00140 */ 00141 Result getInitSeq(char* buf, int len); 00142 00143 /** Get the power down sequence stored in persistent storage on the 00144 * LCD Board 00145 * 00146 * @param buf buffer to which the string will be copied 00147 * @param len size of the buffer in bytes 00148 * 00149 * @returns the result of the operation 00150 */ 00151 Result getPowerDownSeq(char* buf, int len); 00152 00153 /** 00154 * Get the touch panel parameters stored in persistent storage 00155 * 00156 * @param params pointer to a configuration object. Parameters are copied to 00157 * this object. 00158 * 00159 * @returns the result of the operation 00160 */ 00161 Result getTouchParameters(TouchParams_t* params); 00162 00163 /** 00164 * Write display parameters to the EEPROM. Please use this function with 00165 * care since original parameters will be overwritten and cannot be restored. 00166 * 00167 * @param lcdName the name of the display 00168 * @param lcdMfg the display manufacturer 00169 * @param cfg the display configuration parameters 00170 * @param initSeqStr the initialization sequence string 00171 * @param pdSeqStr the power down sequence string 00172 * @param touch touch panel parameters 00173 */ 00174 Result storeParameters( 00175 const char* lcdName, 00176 const char* lcdMfg, 00177 LcdController::Config* cfg, 00178 const char* initSeqStr, 00179 const char* pdSeqStr, 00180 TouchParams_t* touch, 00181 bool controlWp = false); 00182 00183 00184 private: 00185 00186 typedef struct { 00187 uint32_t magic; // magic number 00188 uint8_t lcd_name[NameBufferSize]; // LCD name 00189 uint8_t lcd_mfg[NameBufferSize]; // manufacturer name 00190 uint16_t lcdParamOff; // offset to LCD parameters 00191 uint16_t initOff; // offset to init sequence string 00192 uint16_t pdOff; // offset to power down sequence string 00193 uint16_t tsOff; // offset to touch parameters 00194 uint16_t end; // end offset 00195 } store_t; 00196 00197 Result getStore(store_t* store); 00198 00199 int eepromRead(uint8_t* buf, uint16_t offset, uint16_t len); 00200 int eepromWrite(uint8_t* buf, uint16_t offset, uint16_t len); 00201 00202 Result parseInitString(char* str, LcdController::Config* cfg); 00203 Result checkVersion(char* v, uint32_t len); 00204 Result execDelay(char* del, uint32_t len); 00205 Result execSeqCtrl(char* cmd, uint32_t len); 00206 Result execPinSet(char* cmd, uint32_t len); 00207 00208 void setLsStates(uint16_t states, uint8_t* ls, uint8_t mode); 00209 void setLeds(void); 00210 void pca9532_setLeds (uint16_t ledOnMask, uint16_t ledOffMask); 00211 void pca9532_setBlink0Period(uint8_t period); 00212 void pca9532_setBlink0Duty(uint8_t duty); 00213 void pca9532_setBlink0Leds(uint16_t ledMask); 00214 00215 virtual void setWriteProtect(bool enable); 00216 virtual void set3V3Signal(bool enabled); 00217 virtual void set5VSignal(bool enabled); 00218 virtual void setDisplayEnableSignal(bool enabled); 00219 virtual void setBacklightContrast(uint32_t value); 00220 00221 I2C _i2c; 00222 LcdController::Config _cfg; 00223 LcdController lcdCtrl; 00224 uint16_t _blink0Shadow; 00225 uint16_t _blink1Shadow; 00226 uint16_t _ledStateShadow; 00227 bool _lcdPwrOn; 00228 }; 00229 00230 #endif
Generated on Wed Jul 13 2022 02:29:31 by
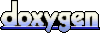