This is a library for the JY-LKM1638 Display
Embed:
(wiki syntax)
Show/hide line numbers
LKM1638.cpp
00001 00002 #include "mbed.h" 00003 #include "LKM1638.h" 00004 00005 00006 const char READMODE = 0x42; 00007 const char INTENSITY = 0x00; //Intensity of LED and Seven Segment Displays (0-7) 00008 const int WAIT = 1; 00009 00010 00011 //MPL3115A2 Class Constructor 00012 LKM1636::LKM1636(PinName pin1, PinName pin2, PinName pin3) :_Strobe(pin1),_CLK(pin2),_Data(pin3) 00013 { 00014 _Data.mode(PullUp); 00015 00016 } 00017 00018 00019 //Driver Function that is used to write any printable char to the seven segment displays 0-7 00020 void LKM1636::displaychar (unsigned char value, unsigned char Seg_num, bool decimal) //Seg_num 0-7 ____,____ 00021 { 00022 00023 unsigned char address; 00024 unsigned char Seg_value=0; 00025 address = 0xC0 + Seg_num * 2; //Base Address for Segments is 0xC0 Each segment uses to Bytes of Mem. 00026 Seg_value = getchar(value); 00027 if(decimal) 00028 Seg_value = Seg_value | 0x80; 00029 if(Seg_value<0xff) // If Seg_value == 0xFF then character was not recognized 00030 { 00031 _Strobe = 0; 00032 SendChar(address); 00033 SendChar(Seg_value); 00034 _Strobe = 1; 00035 Delay(WAIT); 00036 _Strobe = 0; 00037 SendChar(INTENSITY | 0x88); 00038 _Strobe = 1; 00039 } 00040 00041 00042 00043 } 00044 00045 00046 //Prints a Long int to the 8 Seven Segment displays 00047 void LKM1636::Clear() //bank is either Seven Segs 0-3 Bank==0 or Segs 4-7 Bank==1 00048 { 00049 unsigned long temp1=0; 00050 00051 for(temp1=0;temp1<8;temp1++) 00052 { 00053 displaychar (' ',temp1,0); 00054 } 00055 00056 } 00057 00058 00059 00060 ///Writes an 8bool number to either the first or second bank of 4 seven segment displays 00061 00062 void LKM1636::Write_Short(unsigned char value, bool bank) //bank is either Seven Segs 0-3 Bank==0 or Segs 4-7 Bank==1 00063 { 00064 unsigned char temp=0; 00065 unsigned char Seg_number = 0; 00066 if(bank) 00067 Seg_number = 5; 00068 temp = value/100; 00069 temp = temp%10; 00070 displaychar (temp, Seg_number,0); 00071 temp = value/10; 00072 temp = temp%10; 00073 displaychar (temp, Seg_number+1,0); 00074 temp = value%10; 00075 displaychar (temp, Seg_number+2,0); 00076 } 00077 //Prints a Long int to the 8 Seven Segment displays 00078 void LKM1636::Write_Long(unsigned long int value) //bank is either Seven Segs 0-3 Bank==0 or Segs 4-7 Bank==1 00079 { 00080 unsigned long temp1=0; 00081 00082 temp1 = (value/10000000); 00083 temp1 = temp1%10; 00084 displaychar ((unsigned char)temp1,0,0); 00085 00086 temp1 = (value/1000000); 00087 temp1 = temp1%10; 00088 displaychar ((unsigned char)temp1,1,0); 00089 00090 temp1 = (value/100000); 00091 temp1 = temp1%10; 00092 displaychar ((unsigned char)temp1,2,0); 00093 00094 temp1 = (value/10000); 00095 temp1 = temp1%10; 00096 displaychar ((unsigned char)temp1,3,0); 00097 00098 temp1 = (value/1000); 00099 temp1 = temp1%10; 00100 displaychar ((unsigned char)temp1,4,0); 00101 00102 temp1 =(value/100); 00103 temp1 = temp1%10; 00104 displaychar ((unsigned char)temp1,5,0); 00105 00106 temp1 = (value/10); 00107 temp1 = temp1%10; 00108 displaychar ((unsigned char)temp1,6,0); 00109 00110 temp1 = (value%10); 00111 displaychar ((unsigned char)temp1,7,0); 00112 } 00113 00114 // Serializes the Value passed into the character variable and send out using the data pin 00115 void LKM1636::SendChar(unsigned char Character) 00116 { 00117 unsigned char t; 00118 _CLK =1; 00119 _Data.output(); 00120 Delay(WAIT); 00121 for (t=0;t<8;t++) //Serialize Address and put out on Data Pin 00122 { 00123 00124 _Data = (Character & 0x01); 00125 Character = Character>>1; 00126 ToggleClock (); 00127 } 00128 _Data.input(); 00129 00130 } 00131 /// Toggles the Clock pin low to high with a minimal delay between the toggle 00132 void LKM1636::ToggleClock () 00133 { 00134 _CLK = 0; 00135 Delay(WAIT); 00136 _CLK = 1; 00137 Delay(WAIT); 00138 } 00139 /// Delay for Tick * Tocks of system clock 00140 /// 00141 void LKM1636::Delay(unsigned int Tick) 00142 { 00143 unsigned int tock=0,t=0; 00144 for(t=0;t<Tick;t++) 00145 { 00146 for(tock=0;tock<10;tock++); 00147 } 00148 00149 } 00150 00151 /// Getchar takes a integer value 0-15 or ascii value '0' - 'F' 00152 /// and returns the Seven Segment value needed to display the value 00153 00154 unsigned char LKM1636::getchar(unsigned char value) 00155 { 00156 int seg_value = 0; 00157 00158 switch(value) 00159 { 00160 case ('0'): 00161 case (0): 00162 seg_value = 0x3f; 00163 break; 00164 case ('1'): 00165 case (1): 00166 seg_value = 0x6; 00167 break; 00168 case ('2'): 00169 case (2): 00170 seg_value = 0x5b; 00171 break; 00172 case ('3'): 00173 case (3): 00174 seg_value = 0x4f; 00175 break; 00176 case ('4'): 00177 case (4): 00178 seg_value = 0x66; 00179 break; 00180 case ('5'): 00181 case (5): 00182 seg_value = 0x6d; 00183 break; 00184 case ('6'): 00185 case (6): 00186 seg_value = 0x7d; 00187 break; 00188 case ('7'): 00189 case (7): 00190 seg_value = 0x7; 00191 break; 00192 case ('8'): 00193 case (8): 00194 seg_value = 0x7f; 00195 break; 00196 case ('9'): 00197 case (9): 00198 seg_value = 0x6f; 00199 break; 00200 case ('A'): 00201 case ('a'): 00202 case (10): 00203 seg_value = 0x77; 00204 break; 00205 case ('B'): 00206 case ('b'): 00207 case (11): 00208 seg_value = 0x7c; 00209 break; 00210 case ('C'): 00211 case ('c'): 00212 case (12): 00213 seg_value = 0x39; 00214 break; 00215 case ('D'): 00216 case ('d'): 00217 case (13): 00218 seg_value = 0x5e; 00219 break; 00220 case ('E'): 00221 case ('e'): 00222 case (14): 00223 seg_value = 0x79; 00224 break; 00225 case ('F'): 00226 case ('f'): 00227 case (15): 00228 seg_value = 0x71; 00229 break; 00230 case ('.'): 00231 seg_value = 0x80; 00232 break; 00233 case ('-'): 00234 seg_value = 0x40; 00235 break; 00236 case (' '): 00237 seg_value =0x0; 00238 break; 00239 default : 00240 seg_value = 0xff; 00241 break; 00242 00243 } 00244 return (seg_value); 00245 } 00246 00247 //Reads the current state of all 8 input buttons. The state of each button is returned as an 8bool value 00248 //the bool to Sw map: 00249 //Switch bool order bool# 7 6 5 4 3 2 1 0 //0x80 0x20 0x08 0x02 || 0x40 0x10 0x04 0x01 00250 // Sw# 1 5 2 6 3 7 4 8 00251 00252 unsigned char LKM1636::read_buttons () 00253 { 00254 unsigned char temp=0; 00255 char d=0; 00256 _Strobe = 0; 00257 SendChar(READMODE); 00258 //_Data = 1; 00259 _CLK = 1; 00260 Delay(WAIT); 00261 for(d=0;d<32;d++) 00262 { 00263 if(!((d-1)%4)) 00264 { 00265 temp=temp<<1; 00266 if(_Data) 00267 temp+=1; 00268 } 00269 ToggleClock (); 00270 } 00271 _Strobe = 1; 00272 return temp; 00273 } 00274 00275 //Takes the 8bool number returned from the read_buttons function and lights the corsponing LED to current switch posistion 00276 void LKM1636::Switch_To_LED(unsigned char Switch_Values) 00277 { 00278 unsigned char t=0; 00279 bool temp_state=0; 00280 for(t=0; t<8; t++) 00281 { 00282 temp_state = (Switch_Values & 0x01); 00283 Switch_Values= Switch_Values>>1; 00284 switch (t) 00285 { 00286 case (0): 00287 LED (7, temp_state); 00288 break; 00289 case (1): 00290 LED (3, temp_state); 00291 break; 00292 case (2): 00293 LED (6, temp_state); 00294 break; 00295 case (3): 00296 LED (2, temp_state); 00297 break; 00298 case (4): 00299 LED (5, temp_state); 00300 break; 00301 case (5): 00302 LED (1, temp_state); 00303 break; 00304 case (6): 00305 LED (4, temp_state); 00306 break; 00307 case (7): 00308 LED (0, temp_state); 00309 break; 00310 default: 00311 break; 00312 } 00313 00314 } 00315 } 00316 00317 //LED_Num (0-7)) corsponds to the LED that is to be written to state(1=led on, 0 = led off) 00318 void LKM1636::LED (unsigned char LED_Num, bool state)//intensity can range from 0-7 00319 { 00320 unsigned char LED_Address; 00321 LED_Address = 0xC0 + (LED_Num*2) + 1; //LED are at odd address 1 to 15; 00322 _Strobe = 0; 00323 SendChar(LED_Address); 00324 if(state) 00325 SendChar(0x01); 00326 else 00327 SendChar(0x00); 00328 _Strobe = 1; 00329 Delay(WAIT); 00330 _Strobe = 0; 00331 SendChar(INTENSITY | 0x88); 00332 _Strobe = 1; 00333 } 00334 //Takes a 8bool number and writes out the value to the 8 leds (0-7) 00335 void LKM1636::LEDS (unsigned char LED_Values) 00336 { 00337 unsigned char temp; 00338 bool temp_state = 0 ; 00339 for(temp=0; temp<8; temp++) 00340 { 00341 temp_state = (LED_Values & 0x01); 00342 LED (temp, temp_state); 00343 LED_Values= LED_Values>>1; 00344 } 00345 }
Generated on Sun Jul 24 2022 14:46:23 by
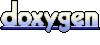