
Second revision of test code for Polyathalon sensor board.
Embed:
(wiki syntax)
Show/hide line numbers
Quaternion.h
Go to the documentation of this file.
00001 /** 00002 * @file Quaternion.h 00003 * @author Mike Panetta 00004 * 00005 * Created Oct 29th 2011 00006 * 00007 * Based on tutorial here: http://www.cprogramming.com/tutorial/3d/quaternions.html 00008 * 00009 */ 00010 00011 #ifndef _QUATERNION_H_ 00012 #define _QUATERNION_H_ 00013 00014 #include <math.h> 00015 00016 class Quaternion 00017 { 00018 public: 00019 Quaternion(float const & a, 00020 float const & b, 00021 float const & c, 00022 float const & d) : w(a), x(b), y(c), z(d) {} 00023 00024 float norm(void) 00025 { 00026 return sqrtf(w*w+x*x+y*y+z*z); 00027 } 00028 00029 float real(void) 00030 { 00031 return w; 00032 } 00033 00034 Quaternion unreal(void) 00035 { 00036 return Quaternion(0, x, y, z); 00037 } 00038 00039 private: 00040 00041 float w, x, y, z; 00042 }; 00043 00044 00045 #endif //_QUATERNION_H)_
Generated on Sat Jul 16 2022 02:43:59 by
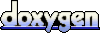