
Read the Bosch BME680 sensor on Nuvoton NuMaker board
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "mbed_bme680.h" 00003 00004 #if TARGET_NUMAKER_IOT_M263A 00005 I2C i2c(PD_4, PD_5); 00006 #else 00007 I2C i2c(I2C_SDA, I2C_SCL); // Used inside the BME680 Mbed Lib. 00008 #endif 00009 00010 BME680 bme680(0x76 << 1); 00011 00012 int main() 00013 { 00014 int count = 10; 00015 00016 if (!bme680.begin()) { 00017 printf("BME680 Begin failed \r\n"); 00018 return 1; 00019 } 00020 00021 while (true) { 00022 if (++count >= 10) 00023 { 00024 count = 0; 00025 printf("\r\nTemperature Humidity Pressure VOC\r\n" 00026 " degC %% hPa KOhms\r\n" 00027 "------------------------------------------\r\n"); 00028 } 00029 00030 if (bme680.performReading()) 00031 { 00032 printf(" %.2f ", bme680.getTemperature()); 00033 printf("%.2f ", bme680.getHumidity()); 00034 printf("%.2f ", bme680.getPressure() / 100.0); 00035 printf("%0.2f\r\n", bme680.getGasResistance() / 1000.0); 00036 } 00037 00038 thread_sleep_for(1000); 00039 } 00040 }
Generated on Tue Jul 12 2022 20:58:28 by
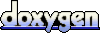