Library for Modtronix NZ32 STM32 boards, like the NZ32-SC151, NZ32-SB072, NZ32-SE411 and others
Embed:
(wiki syntax)
Show/hide line numbers
nz32s.cpp
00001 /** 00002 * File: nz32s.cpp 00003 * 00004 * Author: Modtronix Engineering - www.modtronix.com 00005 * 00006 * Description: 00007 * 00008 * Software License Agreement: 00009 * This software has been written or modified by Modtronix Engineering. The code 00010 * may be modified and can be used free of charge for commercial and non commercial 00011 * applications. If this is modified software, any license conditions from original 00012 * software also apply. Any redistribution must include reference to 'Modtronix 00013 * Engineering' and web link(www.modtronix.com) in the file header. 00014 * 00015 * THIS SOFTWARE IS PROVIDED IN AN 'AS IS' CONDITION. NO WARRANTIES, WHETHER EXPRESS, 00016 * IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00017 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE 00018 * COMPANY SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR 00019 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00020 */ 00021 #define THIS_IS_NZ32S_CPP 00022 00023 #include "mbed.h" 00024 #include <stdarg.h> 00025 #include <stdio.h> 00026 00027 #define DEBUG_ENABLE 1 00028 #include "mx_default_debug.h" 00029 00030 //Includes that use debugging - Include AFTER debug defines/includes above! It uses them for debugging! 00031 #define DEBUG_ENABLE_NZ32S 0 00032 #define DEBUG_ENABLE_INFO_NZ32S 0 00033 #include "nz32s.h" 00034 00035 00036 // DEFINES //////////////////////////////////////////////////////////////////// 00037 00038 00039 // GLOBAL VARIABLES /////////////////////////////////////////////////////////// 00040 00041 00042 // STATIC OBJECTS ///////////////////////////////////////////////////////////// 00043 DigitalOut NZ32S::led1(LED1); 00044 DigitalIn NZ32S::btn1(USER_BUTTON, PullDown); 00045 #if (NZ32S_USE_WWDG==1) 00046 WWDG_HandleTypeDef NZ32S::hwwdg; //Windowed Watchdog Timer, is stopped during low power mode 00047 #else 00048 IWDG_HandleTypeDef NZ32S::hiwdg; //Independent Watchdog Timer, is NOT stopped during low power mode! 00049 #endif 00050 #if (NZ32S_USE_A13_A14 == 1) 00051 DigitalInOut NZ32S::enableFastCharge(PA_14, PIN_INPUT, PullNone, 0); 00052 #endif 00053 00054 00055 // Function Prototypes //////////////////////////////////////////////////////// 00056 00057 00058 /** Constructor 00059 */ 00060 NZ32S::NZ32S() { 00061 led1 = 0; //User LED off 00062 } 00063 00064 00065 /** Get the battery voltage. 00066 */ 00067 uint16_t NZ32S::get_batt_mv() { 00068 MX_DEBUG("\r\nnz32s_getBattMV()"); 00069 00070 //uint16_t getBattMV(void) { 00071 // float fval = 0; 00072 // 00073 //#if (NZ32S_USE_A13_A14 == 1) 00074 //// uint16_t meas; 00075 //// 00076 //// //Measure Vbatt. It doesn't seem to make lots of a difference if we disable the DC/DC converter! 00077 //// ctrVBatt = 0; 00078 //// ctrVBatt.output(); 00079 //// wait_ms(150); 00080 //// meas = ainVBatt.read_u16(); // Converts and read the analog input value 00081 //// ctrVBatt.input(); 00082 //// //fval = ((float)meas / (float)0xffff) * 6600; //6600 = 3300*2, because resistor divider = 2 00083 //// fval = meas * ((float)6600.0 / (float)65535.0); 00084 //#endif 00085 // 00086 // return (uint16_t) fval; 00087 return 0; 00088 } 00089 00090 00091 /** 00092 * Get Vusb or 5V supply voltage in millivolts. 00093 */ 00094 uint16_t NZ32S::get_supply_mv(void) { 00095 // float fval; 00096 // uint16_t meas; 00097 // 00098 // //Following voltages were measured at input of ADC: 00099 // // 00100 // //----- Vusb=0V & 5V supply=0V ----- 00101 // //Value is typically 4mV 00102 // // 00103 // //----- Vusb=5V & 5V supply=0V ----- 00104 // //When only VUSB is supplied, the value is typically 1.95V 00105 // // 00106 // //----- Vusb=0V & 5V supply=5V ----- 00107 // //When only VUSB is supplied, the value is typically 1.95V 00108 // // 00109 // //The reason this circuit does not work, is because of the reverse voltage of the diodes. It is given 00110 // //as about 40 to 80uA. With a resistor value of 470K, this will put 5V on other side of diode. 00111 // //Thus, no matter if 5V is at Vusb, Vsupply, or both, the read value will always be 1.95V. 00112 // //To solve problem, resistors must be lowered to value where 80uA will no longer give more than 5V 00113 // //voltage drop = 62k. Using two 47k resistors should work. 00114 // 00115 // //Measure Vusb/5V sense input 00116 // meas = ainVSense.read_u16(); // Converts and read the analog input value 00117 // fval = ((float) meas / (float) 0xffff) * 3300; 00118 // 00119 // return (uint16_t) fval; 00120 return 0; 00121 } 00122 00123 00124 /** 00125 * Reset I2C bus 00126 */ 00127 void NZ32S::i2c_reset(uint8_t busNumber, PinName sda, PinName scl) { 00128 #if defined(STM32) || defined(STM32L1) 00129 i2c_t objI2C; 00130 00131 if (busNumber == 1) { 00132 objI2C.i2c = I2C_1; 00133 } 00134 else if (busNumber == 2) { 00135 objI2C.i2c = I2C_2; 00136 } 00137 00138 objI2C.slave = 0; 00139 i2c_init(&objI2C, sda, scl); 00140 //i2c_reset(&objI2C); //i2c_init() above calls i2c_reset() 00141 //pc.printf("\r\nI2C1 Reset"); 00142 00143 MX_DEBUG("\r\nI2C1 Reset"); 00144 00145 #endif //#if defined(STM32) || defined(STM32L1) 00146 } 00147 00148 void NZ32S::print_reset_cause(bool clearFlags) { 00149 #if defined(STM32) || defined(STM32L1) 00150 if ( __HAL_RCC_GET_FLAG(PWR_FLAG_SB)) 00151 MX_DEBUG("\r\nSystem resumed from STANDBY mode"); 00152 00153 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_SFTRST)) 00154 MX_DEBUG("\r\nSoftware Reset"); 00155 00156 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_PORRST)) 00157 MX_DEBUG("\r\nPower-On-Reset"); 00158 00159 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_PINRST)) // Always set, test other cases first 00160 MX_DEBUG("\r\nExternal Pin Reset"); 00161 00162 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_IWDGRST) != RESET) 00163 MX_DEBUG("\r\nWatchdog Reset"); 00164 00165 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_WWDGRST) != RESET) 00166 MX_DEBUG("\r\nWindow Watchdog Reset"); 00167 00168 if ( __HAL_RCC_GET_FLAG(RCC_FLAG_LPWRRST) != RESET) 00169 MX_DEBUG("\r\nLow Power Reset"); 00170 00171 // if ( __HAL_RCC_GET_FLAG(RCC_FLAG_BORRST) != RESET) // F4 Usually set with POR 00172 // MX_DEBUG("\r\nBrown-Out Reset"); 00173 00174 if (clearFlags) { 00175 __HAL_RCC_CLEAR_RESET_FLAGS(); // The flags cleared after use 00176 } 00177 #endif //#if defined(STM32) || defined(STM32L1) 00178 } 00179
Generated on Tue Jul 12 2022 15:45:25 by
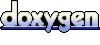