Library for Modtronix NZ32 STM32 boards, like the NZ32-SC151, NZ32-SB072, NZ32-SE411 and others
Embed:
(wiki syntax)
Show/hide line numbers
mx_helpers.cpp
00001 /** 00002 * File: mx_helpers.cpp 00003 * 00004 * Author: Modtronix Engineering - www.modtronix.com 00005 * 00006 * Description: 00007 * 00008 * Software License Agreement: 00009 * This software has been written or modified by Modtronix Engineering. The code 00010 * may be modified and can be used free of charge for commercial and non commercial 00011 * applications. If this is modified software, any license conditions from original 00012 * software also apply. Any redistribution must include reference to 'Modtronix 00013 * Engineering' and web link(www.modtronix.com) in the file header. 00014 * 00015 * THIS SOFTWARE IS PROVIDED IN AN 'AS IS' CONDITION. NO WARRANTIES, WHETHER EXPRESS, 00016 * IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00017 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE 00018 * COMPANY SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR 00019 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00020 */ 00021 #include "mbed.h" 00022 00023 #define DEBUG_ENABLE 1 00024 #include "mx_default_debug.h" 00025 00026 //Includes that use debugging - Include AFTER debug defines/includes above! It uses them for debugging! 00027 #include "mx_helpers.h" 00028 00029 uint8_t MxHelpers::ascii_hex_to_byte(WORD_VAL asciiChars) { 00030 // Convert lowercase to uppercase 00031 if (asciiChars.v[1] > 'F') 00032 asciiChars.v[1] -= 'a' - 'A'; 00033 if (asciiChars.v[0] > 'F') 00034 asciiChars.v[0] -= 'a' - 'A'; 00035 00036 // Convert 0-9, A-F to 0x0-0xF 00037 if (asciiChars.v[1] > '9') 00038 asciiChars.v[1] -= 'A' - 10; 00039 else 00040 asciiChars.v[1] -= '0'; 00041 00042 if (asciiChars.v[0] > '9') 00043 asciiChars.v[0] -= 'A' - 10; 00044 else 00045 asciiChars.v[0] -= '0'; 00046 00047 // Concatenate 00048 return (asciiChars.v[1] << 4) | asciiChars.v[0]; 00049 } 00050 00051 uint8_t MxHelpers::ascii_hex_nibble_to_byte(uint8_t c) { 00052 if ((c <= '9') && (c >= '0')) 00053 return (c - '0'); 00054 else if ((c <= 'F') && (c >= 'A')) 00055 return (c - 55); 00056 else if ((c <= 'f') && (c >= 'a')) 00057 return (c - 87); 00058 00059 return 0xff; //Indicate given byte was NOT alpha numerical 00060 } 00061 00062 00063 uint16_t MxHelpers::cvt_ascii_dec_to_uint16(const char* str, uint8_t* retFlags) { 00064 uint16_t val = 0; //Returned value 00065 uint8_t ret = 0; //Return retFlags value 00066 bool isHex = 0; 00067 const char* savedStrPtr; 00068 00069 savedStrPtr = str; 00070 00071 //MX_DEBUG("\nparseHexDecWord()"); 00072 00073 //Does the string contain a Upper Case Hex value 00074 if (*str == 'x') { 00075 str++; 00076 isHex = true; 00077 //MX_DEBUG(" -d"); 00078 } 00079 00080 //Parse all '0'-'9', and 'A'-'F' bytes 00081 while ((((*str >= '0') && (*str <= '9')) 00082 || ((*str >= 'A') && (*str <= 'F')))) { 00083 if (isHex) { 00084 val = (val << 4) 00085 + ((*str <= '9') ? (*str - '0') : (*str - 'A' + 10)); 00086 } else { 00087 val = (val * 10) + ((*str) - '0'); 00088 } 00089 str++; 00090 } 00091 00092 //Get the number of bytes parsed 00093 ret = str - savedStrPtr; 00094 if (retFlags != NULL) { 00095 *retFlags = ret; 00096 } 00097 00098 return val; 00099 } 00100 00101 00102 uint16_t MxHelpers::cvt_ascii_hex_to_uint16(const char* str, uint8_t* retFlags) { 00103 uint16_t retVal = 0; //Returned value 00104 uint8_t ret = 0; //Return retFlags value 00105 bool isDecimal = 0; 00106 const char* savedStrPtr; 00107 00108 savedStrPtr = str; 00109 00110 //MX_DEBUG("\nparseHexDecWord()"); 00111 00112 //Does the string contain a decimal value 00113 if (*str == 'd') { 00114 str++; 00115 isDecimal = true; 00116 //MX_DEBUG(" -d"); 00117 } 00118 00119 //Parse all '0'-'9', and 'A'-'F' bytes 00120 while ((((*str >= '0') && (*str <= '9')) 00121 || ((*str >= 'A') && (*str <= 'F')))) { 00122 if (isDecimal) { 00123 retVal = (retVal * 10) + ((*str) - '0'); 00124 } else { 00125 retVal = (retVal << 4) 00126 + ((*str <= '9') ? (*str - '0') : (*str - 'A' + 10)); 00127 } 00128 str++; 00129 } 00130 00131 //Get the number of bytes parsed 00132 ret = str - savedStrPtr; 00133 if (retFlags != NULL) { 00134 *retFlags = ret; 00135 } 00136 00137 return retVal; 00138 } 00139 00140 00141 uint8_t MxHelpers::cvt_uint16_to_ascii_str(uint16_t val, uint8_t* buf) { 00142 uint8_t i; 00143 uint8_t retVal = 0; 00144 uint16_t digit; 00145 uint16_t divisor; 00146 bool printed = false; 00147 //const uint16_t NZ_UITOA_DIV[4] = { 1, 10, 100, 1000 }; 00148 //static const uint16_t NZ_UITOA_DIV[4] = { 1, 10, 100, 1000 }; 00149 00150 if (val) { 00151 divisor = 10000; 00152 for (i = 5; i != 0; i--) { 00153 digit = val / divisor; 00154 if ((digit!=0) || printed) { 00155 *buf++ = '0' + digit; 00156 val -= digit * divisor; 00157 printed = true; 00158 retVal++; 00159 } 00160 divisor = divisor / 10; 00161 00162 //Alternative method 00163 //divisor = NZ_UITOA_DIV[i - 2]; 00164 00165 //Alternative method 00166 //if (i==5) 00167 // divisor = 1000; 00168 //else if (i==4) 00169 // divisor = 100; 00170 //else if (i==3) 00171 // divisor = 10; 00172 //else 00173 // divisor = 1; 00174 } 00175 } else { 00176 *buf++ = '0'; 00177 retVal++; 00178 } 00179 00180 *buf = '\0'; 00181 00182 return retVal; 00183 } 00184 00185 00186 00187
Generated on Tue Jul 12 2022 15:45:25 by
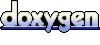