Initial version for Modtronix LCD2S I2C and SPI serial LCD displays. For details, see http://modtronix.com/products-serial-lcd-board/
lcd2s.cpp
00001 /** 00002 * File: lcd2s.cpp 00003 * 00004 * Author: Modtronix Engineering - www.modtronix.com 00005 * 00006 * Description: 00007 * 00008 * Software License Agreement: 00009 * This software has been written or modified by Modtronix Engineering. The code 00010 * may be modified and can be used free of charge for commercial and non commercial 00011 * applications. If this is modified software, any license conditions from original 00012 * software also apply. Any redistribution must include reference to 'Modtronix 00013 * Engineering' and web link(www.modtronix.com) in the file header. 00014 * 00015 * THIS SOFTWARE IS PROVIDED IN AN 'AS IS' CONDITION. NO WARRANTIES, WHETHER EXPRESS, 00016 * IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00017 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE 00018 * COMPANY SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR 00019 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00020 */ 00021 #include "mbed.h" 00022 #include "lcd2s.h" 00023 00024 00025 // DEFINES //////////////////////////////////////////////////////////////////// 00026 00027 00028 // GLOBAL VARIABLES /////////////////////////////////////////////////////////// 00029 #if (LCD2S_USE_MALLOC == 0) 00030 uint8_t buf[80]; 00031 uint32_t dirtyRows[4]; 00032 #endif 00033 00034 // Function Prototypes //////////////////////////////////////////////////////// 00035 00036 00037 // DEBUG //////////////////////////////////////////////////////////////////// 00038 //IMPORTANT, when enabling debugging, it is very important to note the following: 00039 //- If (MX_DEBUG_IS_POINTER==1), ensure there is global Stream pointer defined somewhere in code to override "pMxDebug = NULL" below! 00040 //- If (MX_DEBUG_IS_POINTER==0), define a mxDebug() function somewhere in code to handel debug output 00041 #define DEBUG_ENABLE 1 00042 #if (DEBUG_ENABLE==1) && !defined(NDEBUG) 00043 //Alternative method in stead of using NULL below. This requires to create derived Stream class in each file we want to use debugging 00044 // class modtronixDebugStream : public Stream {int _putc(int value) {return 0;}int _getc() {return 0;}}; 00045 // static modtronixDebugStream modtronixDebug; 00046 // WEAK Stream* pMxDebug = &modtronixDebug; 00047 #if (MX_DEBUG_IS_POINTER==1) //More efficient, but only works if pMxDebug is defined in code. Disabled by default! 00048 WEAK Stream* pMxDebug = NULL; 00049 #define MX_DEBUG pMxDebug->printf 00050 #else 00051 WEAK void mxDebug(const char *format, ...) {} 00052 #define MX_DEBUG mxDebug 00053 #endif 00054 #else 00055 //GCC's CPP has extensions; it allows for macros with a variable number of arguments. We use this extension here to preprocess pmesg away. 00056 #define MX_DEBUG(format, args...) ((void)0) 00057 #endif 00058 00059 00060 00061 LCD2S::LCD2S(uint8_t rows/* = 4*/, uint8_t columns/* = 20*/) { 00062 contrast = 200; 00063 brightness = 200; 00064 this->rows = rows; 00065 this->columns = columns; 00066 bufSize = rows * columns; 00067 cursor_row = cursor_col = 0; 00068 00069 //MX_DEBUG("\r\nLCD2S()"); 00070 00071 #if (LCD2S_USE_MALLOC == 1) 00072 buf = new uint8_t[bufSize)]; 00073 dirtyRows = new uint32_t(rows); 00074 #endif 00075 00076 pBuf = buf; 00077 pDirtyRows = dirtyRows; 00078 memset(pBuf, ' ', sizeof(buf)); 00079 memset(pDirtyRows, 0, 4 * rows); 00080 } 00081 00082 void LCD2S::clearDisplay() { 00083 memset(pBuf, ' ', sizeof(buf)); 00084 memset(pDirtyRows, 0xff, 4 * rows); 00085 } 00086 00087 void LCD2S::setCursor(int8_t row, int8_t col) { 00088 cursor_row = row-1; 00089 if(cursor_row > rows) 00090 cursor_row = rows-1; 00091 00092 cursor_col = col-1; 00093 if(cursor_col > columns) 00094 cursor_col = columns-1; 00095 }; 00096
Generated on Fri Jul 15 2022 08:00:12 by
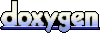