
This program enables the use of the Semtech "SX1276 Starter Kit A" application to be used with the FRDM-KL25Z board. At the time of writing this, this app was available on Semtech's SX1276 page, in the "Docs & Resources"* tab, via the link "PC GUI (1.0.0Beta5)". This program was tested with the FRDM-KL25Z board, with a Modtronix inAir9 SX1276 board mounted in a Modtronix SHD3I shield. The inAir9 module is mounted in iMod port 3 of the SHD3I module. The SHD3I shield is mounted on the FRDM-KL25Z board.
Dependencies: SX127x_modtronix USBDevice_Semtech_GUI mbed
Fork of hid_test by
main.cpp
00001 #include "mbed.h" 00002 #include "USBHID.h" 00003 #include "sx127x.h" 00004 00005 //#define _DEBUG_ 00006 00007 /* 00008 * This program is a modified version of the "hid_test" application by Wayne Roberts. All credits go 00009 * to Wayne for writing it, I just did some minor modiciations! 00010 * 00011 * The program was tested with the FRDM-KL25Z board, with a Modtronix inAir9 SX1276 board mounted in 00012 * a Modtronix SHD3I shield. The inAir9 module is mounted in iMod port 3 of the SHD3I module. The 00013 * SHD3I shield is mounted on the FRDM-KL25Z board. 00014 * It enables the use of the Semtech "SX1276 Starter Kit A" application to be used with this board. 00015 * At the time of writing this, this app was available on Semtech's SX1276 page, in the "Docs & Resources" 00016 * tab, via the link "PC GUI (1.0.0Beta5)". 00017 * This app can be used for sending and receiving data via the SX1276. To use it: 00018 * - Plug the FRDM-KL25Z into the PC via the USB marked "USB KL25Z" 00019 * - Start Semtech "SX1276 Starter Kit A" app on PC 00020 * - App should connect to board, enabling it to control board. 00021 * 00022 * ========== Firmware for KL25Z ========== 00023 * The default firmware listed on mbed.org puts a clock pulse on pin A5. To fix this, use alternative 00024 * firmware (or unsolder resistor R24). 00025 * - Download "OpenSDA Firmware" from http://www.pemicro.com/opensda/ 00026 * - Put KL25Z into bootloader mode (hold down button while plugging in SDA USB). 00027 * - Drag "MSD-DEBUG-FRDM-KL25Z_Pemicro_v114.SDA" file onto it. 00028 * - Reset KL25Z. 00029 * - If the USB drivers do not automatically install, download and run "Windows USB Drivers" from 00030 * www.pemicro.com/opensda. After this, when you plug in the KL25Z next time, all drivers should 00031 * install correctly. 00032 */ 00033 00034 #define TARGET_KL25Z_SHD3I_INAIR8 /* FRDM-KL25Z + Modtronix inAir9 SX1276 board + SHD3I daughter board */ 00035 00036 //Defines for the FRDM-KL25Z board, with Modtronix SHD3I with an inAir9 SX1276 module mounted in it. 00037 #ifdef TARGET_KL25Z_SHD3I_INAIR8 00038 //SCLK=D13, MISO=D12, MOSI=D11 00039 //CS=D7, Reset=A5 00040 //DIO0=D2, DIO1=D8, DIO2=D4, DIO3=A4, DIO4=N.C., DIO5=D3 00041 // mosi, miso, sclk, cs, rst, dio0, dio1 00042 SX127x radio(D11, D12, D13, D7, A5, D2, D8); 00043 #else 00044 // pin: 3 8 1 7 10 12 5 00045 // mosi, miso, sclk, cs, rst, dio0, dio1 00046 // D11 D12 D13 D10 D9 D8 D2 00047 SX127x radio(PTD2, PTD3, PTD1, PTD0, PTD5, PTA13, PTD4); 00048 #endif 00049 00050 #ifdef _DEBUG_ 00051 #include "sx127x_lora.h" 00052 SX127x_lora lora(radio); 00053 #endif /* _DEBUG_ */ 00054 00055 //We declare a USBHID device. By default input and output reports are 64 bytes long. 00056 USBHID hid(64, 64, 0x47a, 0x0b); 00057 //USBHID (uint8_t output_report_length=64, uint8_t input_report_length=64, uint16_t vendor_id=0x1234, uint16_t product_id=0x0006, uint16_t product_release=0x0001, bool connect=true) 00058 00059 Serial pc(USBTX, USBRX); 00060 00061 //This report will contain data to be sent 00062 HID_REPORT send_report; 00063 HID_REPORT recv_report; 00064 00065 DigitalOut l1(LED1); 00066 00067 #define FW_VERSION "3.1.0" 00068 #define SK_NAME "mbed" 00069 00070 #define HID_SK_RESET 0x00 00071 #define HID_SK_GET_VERSION 0x01 00072 #define HID_SK_GET_NAME 0x02 00073 #define HID_SK_GET_PIN 0x10 00074 #define HID_SK_SET_PIN 0x11 00075 #define HID_SK_GET_PINS 0x14 00076 #define HID_DEVICE_READ 0x80 00077 #define HID_DEVICE_WRITE 0x81 00078 #define HID_DEVICE_INIT 0x88 00079 #define HID_DEVICE_RESET 0x89 00080 #define HID_SK_CMD_NONE 0xFF 00081 00082 typedef struct sHidCommand 00083 { 00084 uint8_t Cmd; 00085 uint8_t CmdOpt; 00086 uint8_t CmdDataSize; 00087 uint8_t *CmdData; 00088 } tHidCommand; 00089 00090 typedef enum 00091 { 00092 SX_OK, 00093 SX_ERROR, 00094 SX_BUSY, 00095 SX_EMPTY, 00096 SX_DONE, 00097 SX_TIMEOUT, 00098 SX_UNSUPPORTED, 00099 SX_WAIT, 00100 SX_CLOSE, 00101 SX_YES, 00102 SX_NO, 00103 } tReturnCodes; 00104 00105 #ifdef _DEBUG_ 00106 char verbose = 0; 00107 #endif /* _DEBUG_ */ 00108 00109 //Following code is from V7 00110 //DigitalOut rfsw1(PTC8); 00111 //DigitalOut rfsw2(PTC9); 00112 00113 void rfsw_callback() 00114 { 00115 /* 00116 if (radio.RegOpMode.bits.Mode == RF_OPMODE_TRANSMITTER) { // start of transmission 00117 if (radio.HF) { 00118 if (radio.RegPaConfig.bits.PaSelect) { // if PA_BOOST 00119 rfsw2 = 0; 00120 rfsw1 = 1; 00121 } else { // RFO to power amp 00122 rfsw2 = 1; 00123 rfsw1 = 0; 00124 } 00125 } else { 00126 // todo: sx1276 00127 } 00128 } else if (radio.RegOpMode.bits.Mode == RF_OPMODE_RECEIVER || radio.RegOpMode.bits.Mode == RF_OPMODE_CAD) { // start of reception 00129 if (radio.HF) { 00130 rfsw2 = 1; 00131 rfsw1 = 1; 00132 } else { 00133 // todo: sx1276 00134 } 00135 } else { // RF switch shutdown 00136 rfsw2 = 0; 00137 rfsw1 = 0; 00138 } 00139 */ 00140 } 00141 00142 00143 void HidDecodeCommand( uint8_t *hidReport, tHidCommand *cmd ) 00144 { 00145 cmd->Cmd = hidReport[0]; 00146 cmd->CmdOpt = hidReport[1]; 00147 cmd->CmdDataSize = hidReport[2]; 00148 cmd->CmdData = hidReport + 3; 00149 } 00150 00151 void HidEncodeCommandAns( uint8_t cmd, uint8_t stat, uint8_t dataSize, uint8_t *data ) 00152 { 00153 send_report.data[0] = cmd; 00154 send_report.data[1] = stat; 00155 00156 // TimeStamp 00157 memset( send_report.data + 2, 0, 8 ); 00158 00159 send_report.data[10] = dataSize; 00160 memcpy( send_report.data + 11, ( const void* )data, dataSize ); 00161 00162 //send_report.length = 11 + dataSize; 00163 send_report.length = 64; 00164 hid.send(&send_report); 00165 } 00166 00167 void HidCmdProcess(void) 00168 { 00169 uint8_t stat = SX_OK; 00170 uint8_t size = 0; 00171 uint8_t dataBuffer[64]; 00172 tHidCommand cmd = { HID_SK_CMD_NONE, 0, 0, NULL }; 00173 #ifdef _DEBUG_ 00174 int i; 00175 #endif /* _DEBUG_ */ 00176 00177 HidDecodeCommand(recv_report.data, &cmd); 00178 00179 switch (cmd.Cmd) { 00180 case HID_DEVICE_RESET: 00181 radio.hw_reset(); 00182 break; 00183 case HID_SK_RESET: 00184 case HID_DEVICE_INIT: 00185 radio.hw_reset(); 00186 #ifdef _DEBUG_ 00187 if (verbose) 00188 printf("reset-init\r\n"); 00189 #endif /* _DEBUG_ */ 00190 radio.init(); //SX1272Init( ); 00191 // Set FSK modem ON 00192 radio.set_opmode(RF_OPMODE_SLEEP); 00193 radio.RegOpMode.bits.LongRangeMode = 0; 00194 radio.write_reg(REG_OPMODE, radio.RegOpMode.octet); 00195 //radio.SetLoRaOn( false ); //SX1272SetLoRaOn( false ); // Default radio setting 00196 // Default answer settings 00197 break; 00198 case HID_SK_GET_VERSION: 00199 strcpy( ( char* )dataBuffer, FW_VERSION ); 00200 size = strlen( FW_VERSION ); 00201 break; 00202 case HID_DEVICE_READ: 00203 // cmd.CmdData[0] = size 00204 // cmd.CmdData[1] = address 00205 size = cmd.CmdData[0]; 00206 radio.ReadBuffer( cmd.CmdData[1], dataBuffer, size ); 00207 #ifdef _DEBUG_ 00208 if (verbose) { 00209 pc.printf("read %d bytes from %02x: ", size, cmd.CmdData[1]); 00210 for (i = 0; i < size; i++) 00211 pc.printf("%02x ", dataBuffer[i]); 00212 pc.printf("\r\n"); 00213 } 00214 #endif /* _DEBUG_ */ 00215 stat = SX_OK; 00216 break; 00217 case HID_SK_GET_PINS: 00218 dataBuffer[0] = 0; 00219 if (radio.dio0) 00220 dataBuffer[0] |= 0x01; 00221 if (radio.dio1) 00222 dataBuffer[0] |= 0x02; 00223 #ifdef _DEBUG_ 00224 if (verbose && dataBuffer[0] != 0) 00225 printf("HID_SK_GET_PINS:%02x\r\n", dataBuffer[0]); 00226 #endif /* _DEBUG_ */ 00227 /*dataBuffer[0] |= DIO1 << 1; 00228 dataBuffer[0] |= DIO2 << 2; 00229 dataBuffer[0] |= DIO3 << 3; 00230 dataBuffer[0] |= DIO4 << 4; 00231 dataBuffer[0] |= DIO5 << 5;*/ 00232 size = 1; 00233 break; 00234 case HID_SK_GET_PIN: 00235 // cmd.CmdData[0] = Pin id 00236 switch( cmd.CmdData[0] ) { 00237 case 11: // FEM_CPS_PIN 00238 //MODTRONIX modified, we do not have a femcps pin 00239 //dataBuffer[0] = radio.femcps; 00240 dataBuffer[0] = 0; 00241 #ifdef _DEBUG_ 00242 if (verbose) 00243 printf("HID_SK_GET_PIN femcps:%02x\r\n", dataBuffer[0]); 00244 #endif /* _DEBUG_ */ 00245 break; 00246 case 12: // FEM_CTX_PIN 00247 //MODTRONIX modified, we do not have a femctx pin 00248 //dataBuffer[0] = radio.femctx; 00249 dataBuffer[0] = 0; 00250 #ifdef _DEBUG_ 00251 if (verbose) 00252 printf("HID_SK_GET_PIN femctx:%02x\r\n", dataBuffer[0]); 00253 #endif /* _DEBUG_ */ 00254 break; 00255 default: 00256 dataBuffer[0] = 0xFF; // Signal ID error 00257 #ifdef _DEBUG_ 00258 printf("HID_SK_GET_PIN %d\r\n", cmd.CmdData[0]); 00259 #endif /* _DEBUG_ */ 00260 break; 00261 } // ...switch( cmd.CmdData[0] ) 00262 break; 00263 case HID_SK_SET_PIN: 00264 // cmd.CmdData[0] = Pin id 00265 // cmd.CmdData[1] = Pin state 00266 switch( cmd.CmdData[0] ) { 00267 case 6: 00268 case 7: 00269 case 8: 00270 // ignore LEDs 00271 break; 00272 case 11: // FEM_CPS_PIN 00273 //MODTRONIX modified, we do not have a femcps pin 00274 //radio.femcps = cmd.CmdData[1]; 00275 #ifdef _DEBUG_ 00276 if (verbose) 00277 printf("HID_SK_SET_PIN femcps:%d\r\n", (int)radio.femcps); 00278 #endif /* _DEBUG_ */ 00279 break; 00280 case 12: // FEM_CTX_PIN 00281 //MODTRONIX modified, we do not have a femctx pin 00282 //radio.femctx = cmd.CmdData[1]; 00283 #ifdef _DEBUG_ 00284 if (verbose) 00285 printf("HID_SK_SET_PIN femctx:%d\r\n", (int)radio.femctx); 00286 #endif /* _DEBUG_ */ 00287 break; 00288 default: 00289 stat = SX_UNSUPPORTED; 00290 #ifdef _DEBUG_ 00291 pc.printf("HID_SK_SET_PIN %d %d\r\n", cmd.CmdData[0], cmd.CmdData[1]); 00292 #endif /* _DEBUG_ */ 00293 break; 00294 } // ...switch( cmd.CmdData[0] ) 00295 00296 break; 00297 case HID_DEVICE_WRITE: 00298 // cmd.CmdData[0] = size 00299 // cmd.CmdData[1] = address 00300 // cmd.CmdData[2] = Buffer first byte 00301 // cmd.CmdData[2+(size-1)] = Buffer last byte 00302 radio.WriteBuffer( cmd.CmdData[1], cmd.CmdData + 2, cmd.CmdData[0] ); 00303 #ifdef _DEBUG_ 00304 if (verbose) { 00305 pc.printf("write %d bytes to %02x: ", cmd.CmdData[0], cmd.CmdData[1]); 00306 for (i = 0; i < cmd.CmdData[0]; i++) 00307 pc.printf("%02x ", cmd.CmdData[2+i]); 00308 pc.printf("\r\n"); 00309 } 00310 #endif /* _DEBUG_ */ 00311 stat = SX_OK; 00312 break; 00313 case HID_SK_GET_NAME: 00314 strcpy( ( char* )dataBuffer, SK_NAME ); 00315 size = strlen( SK_NAME ); 00316 break; 00317 default: 00318 pc.printf("%d: ", recv_report.length); 00319 for(int i = 0; i < recv_report.length; i++) { 00320 pc.printf("%02x ", recv_report.data[i]); 00321 } 00322 pc.printf("\r\n"); 00323 stat = SX_UNSUPPORTED; 00324 break; 00325 } // ...switch (cmd.Cmd) 00326 00327 HidEncodeCommandAns( cmd.Cmd, stat, size, dataBuffer); 00328 } 00329 00330 #ifdef _DEBUG_ 00331 void printOpMode() 00332 { 00333 radio.RegOpMode.octet = radio.read_reg(REG_OPMODE); 00334 switch (radio.RegOpMode.bits.Mode) { 00335 case RF_OPMODE_SLEEP: printf("[7msleep[0m"); break; 00336 case RF_OPMODE_STANDBY: printf("[7mstby[0m"); break; 00337 case RF_OPMODE_SYNTHESIZER_TX: printf("[33mfstx[0m"); break; 00338 case RF_OPMODE_TRANSMITTER: printf("[31mtx[0m"); break; 00339 case RF_OPMODE_SYNTHESIZER_RX: printf("[33mfsrx[0m"); break; 00340 case RF_OPMODE_RECEIVER: printf("[32mrx[0m"); break; 00341 case 6: 00342 if (radio.RegOpMode.bits.LongRangeMode) 00343 printf("[42mrxs[0m"); 00344 else 00345 printf("-6-"); 00346 break; // todo: different lora/fsk 00347 case 7: 00348 if (radio.RegOpMode.bits.LongRangeMode) 00349 printf("[45mcad[0m"); 00350 else 00351 printf("-7-"); 00352 break; // todo: different lora/fsk 00353 } 00354 } 00355 #endif /* _DEBUG_ */ 00356 00357 #ifdef _DEBUG_ 00358 void 00359 printPa() 00360 { 00361 radio.RegPaConfig.octet = radio.read_reg(REG_PACONFIG); 00362 if (radio.RegPaConfig.bits.PaSelect) { 00363 float output_dBm = 17 - (15-radio.RegPaConfig.bits.OutputPower); 00364 printf(" PABOOST OutputPower=%.1fdBm", output_dBm); 00365 } else { 00366 float pmax = (0.6*radio.RegPaConfig.bits.MaxPower) + 10.8; 00367 float output_dBm = pmax - (15-radio.RegPaConfig.bits.OutputPower); 00368 printf(" RFO pmax=%.1fdBm OutputPower=%.1fdBm", pmax, output_dBm); 00369 } 00370 } 00371 #endif /* _DEBUG_ */ 00372 00373 #ifdef _DEBUG_ 00374 void /* things always present, whether lora or fsk */ 00375 common_print_status() 00376 { 00377 printf("version:0x%02x %.3fMHz ", radio.read_reg(REG_VERSION), radio.get_frf_MHz()); 00378 printOpMode(); 00379 00380 printPa(); 00381 00382 radio.RegOcp.octet = radio.read_reg(REG_OCP); 00383 if (radio.RegOcp.bits.OcpOn) { 00384 int imax = 0; 00385 if (radio.RegOcp.bits.OcpTrim < 16) 00386 imax = 45 + (5 * radio.RegOcp.bits.OcpTrim); 00387 else if (radio.RegOcp.bits.OcpTrim < 28) 00388 imax = -30 + (10 * radio.RegOcp.bits.OcpTrim); 00389 else 00390 imax = 240; 00391 printf(" OcpOn %dmA ", imax); 00392 } else 00393 printf(" OcpOFF "); 00394 00395 printf("\r\n"); 00396 00397 } 00398 #endif /* _DEBUG_ */ 00399 00400 #ifdef _DEBUG_ 00401 void lora_print_dio() 00402 { 00403 radio.RegDioMapping2.octet = radio.read_reg(REG_DIOMAPPING2); 00404 printf("DIO5:"); 00405 switch (radio.RegDioMapping2.bits.Dio5Mapping) { 00406 case 0: printf("ModeReady"); break; 00407 case 1: printf("ClkOut"); break; 00408 case 2: printf("ClkOut"); break; 00409 } 00410 printf(" DIO4:"); 00411 switch (radio.RegDioMapping2.bits.Dio4Mapping) { 00412 case 0: printf("CadDetected"); break; 00413 case 1: printf("PllLock"); break; 00414 case 2: printf("PllLock"); break; 00415 } 00416 radio.RegDioMapping1.octet = radio.read_reg(REG_DIOMAPPING1); 00417 printf(" DIO3:"); 00418 switch (radio.RegDioMapping1.bits.Dio3Mapping) { 00419 case 0: printf("CadDone"); break; 00420 case 1: printf("ValidHeader"); break; 00421 case 2: printf("PayloadCrcError"); break; 00422 } 00423 printf(" DIO2:"); 00424 switch (radio.RegDioMapping1.bits.Dio2Mapping) { 00425 case 0: 00426 case 1: 00427 case 2: 00428 printf("FhssChangeChannel"); 00429 break; 00430 } 00431 printf(" DIO1:"); 00432 switch (radio.RegDioMapping1.bits.Dio1Mapping) { 00433 case 0: printf("RxTimeout"); break; 00434 case 1: printf("FhssChangeChannel"); break; 00435 case 2: printf("CadDetected"); break; 00436 } 00437 printf(" DIO0:"); 00438 switch (radio.RegDioMapping1.bits.Dio0Mapping) { 00439 case 0: printf("RxDone"); break; 00440 case 1: printf("TxDone"); break; 00441 case 2: printf("CadDone"); break; 00442 } 00443 00444 printf("\r\n"); 00445 } 00446 #endif /* _DEBUG_ */ 00447 00448 #ifdef _DEBUG_ 00449 void 00450 printCodingRate(bool from_rx) 00451 { 00452 uint8_t d = lora.getCodingRate(from_rx); 00453 printf("CodingRate:"); 00454 switch (d) { 00455 case 1: printf("4/5 "); break; 00456 case 2: printf("4/6 "); break; 00457 case 3: printf("4/7 "); break; 00458 case 4: printf("4/8 "); break; 00459 default: 00460 printf("%d ", d); 00461 break; 00462 } 00463 } 00464 #endif /* _DEBUG_ */ 00465 00466 #ifdef _DEBUG_ 00467 void printHeaderMode() 00468 { 00469 if (lora.getHeaderMode()) 00470 printf("implicit "); 00471 else 00472 printf("explicit "); 00473 } 00474 #endif /* _DEBUG_ */ 00475 00476 #ifdef _DEBUG_ 00477 void printBw() 00478 { 00479 uint8_t bw = lora.getBw(); 00480 00481 printf("Bw:"); 00482 if (radio.type == SX1276) { 00483 switch (lora.RegModemConfig.sx1276bits.Bw) { 00484 case 0: printf("7.8KHz "); break; 00485 case 1: printf("10.4KHz "); break; 00486 case 2: printf("15.6KHz "); break; 00487 case 3: printf("20.8KHz "); break; 00488 case 4: printf("31.25KHz "); break; 00489 case 5: printf("41.7KHz "); break; 00490 case 6: printf("62.5KHz "); break; 00491 case 7: printf("125KHz "); break; 00492 case 8: printf("250KHz "); break; 00493 case 9: printf("500KHz "); break; 00494 default: printf("%x ", lora.RegModemConfig.sx1276bits.Bw); break; 00495 } 00496 } else if (radio.type == SX1272) { 00497 switch (lora.RegModemConfig.sx1272bits.Bw) { 00498 case 0: printf("125KHz "); break; 00499 case 1: printf("250KHz "); break; 00500 case 2: printf("500KHz "); break; 00501 case 3: printf("11b "); break; 00502 } 00503 } 00504 } 00505 #endif /* _DEBUG_ */ 00506 00507 #ifdef _DEBUG_ 00508 void printSf() 00509 { 00510 // spreading factor same between sx127[26] 00511 printf("sf:%d ", lora.getSf()); 00512 } 00513 #endif /* _DEBUG_ */ 00514 00515 #ifdef _DEBUG_ 00516 void printTxContinuousMode() 00517 { 00518 printf("TxContinuousMode:%d ", lora.RegModemConfig2.sx1276bits.TxContinuousMode); // same for sx1272 and sx1276 00519 } 00520 #endif /* _DEBUG_ */ 00521 00522 #ifdef _DEBUG_ 00523 void printAgcAutoOn() 00524 { 00525 printf("AgcAutoOn:%d", lora.getAgcAutoOn()); 00526 } 00527 #endif /* _DEBUG_ */ 00528 00529 #ifdef _DEBUG_ 00530 void printRxPayloadCrcOn() 00531 { 00532 bool on = lora.getRxPayloadCrcOn(); 00533 //printf("RxPayloadCrcOn:%s ", on ? "on" : "off"); 00534 if (on) 00535 printf("RxPayloadCrcOn:1 = Tx CRC Enabled\r\n"); 00536 else 00537 printf("RxPayloadCrcOn:1 = no Tx CRC\r\n"); 00538 } 00539 #endif /* _DEBUG_ */ 00540 00541 #ifdef _DEBUG_ 00542 void printLoraIrqs_(bool clear) 00543 { 00544 //in radio class -- RegIrqFlags_t RegIrqFlags; 00545 00546 //already read RegIrqFlags.octet = radio.read_reg(REG_LR_IRQFLAGS); 00547 printf("\r\nIrqFlags:"); 00548 if (lora.RegIrqFlags.bits.CadDetected) 00549 printf("CadDetected "); 00550 if (lora.RegIrqFlags.bits.FhssChangeChannel) { 00551 //radio.RegHopChannel.octet = radio.read_reg(REG_LR_HOPCHANNEL); 00552 printf("FhssChangeChannel:%d ", lora.RegHopChannel.bits.FhssPresentChannel); 00553 } 00554 if (lora.RegIrqFlags.bits.CadDone) 00555 printf("CadDone "); 00556 if (lora.RegIrqFlags.bits.TxDone) 00557 printf("TxDone "); 00558 if (lora.RegIrqFlags.bits.ValidHeader) 00559 printf("[42mValidHeader[0m "); 00560 if (lora.RegIrqFlags.bits.PayloadCrcError) 00561 printf("[41mPayloadCrcError[0m "); 00562 if (lora.RegIrqFlags.bits.RxDone) 00563 printf("[42mRxDone[0m "); 00564 if (lora.RegIrqFlags.bits.RxTimeout) 00565 printf("RxTimeout "); 00566 00567 printf("\r\n"); 00568 00569 if (clear) 00570 radio.write_reg(REG_LR_IRQFLAGS, lora.RegIrqFlags.octet); 00571 00572 } 00573 #endif /* _DEBUG_ */ 00574 00575 #ifdef _DEBUG_ 00576 void lora_print_status() 00577 { 00578 uint8_t d; 00579 00580 if (radio.type == SX1276) 00581 printf("\r\nSX1276 "); 00582 else if (radio.type == SX1272) 00583 printf("\r\nSX1272 "); 00584 00585 radio.RegOpMode.octet = radio.read_reg(REG_OPMODE); 00586 if (!radio.RegOpMode.bits.LongRangeMode) { 00587 printf("FSK\r\n"); 00588 return; 00589 } 00590 00591 lora_print_dio(); 00592 printf("LoRa "); 00593 00594 // printing LoRa registers at 0x0d -> 0x3f 00595 00596 lora.RegModemConfig.octet = radio.read_reg(REG_LR_MODEMCONFIG); 00597 lora.RegModemConfig2.octet = radio.read_reg(REG_LR_MODEMCONFIG2); 00598 00599 printCodingRate(false); // false: transmitted coding rate 00600 printHeaderMode(); 00601 printBw(); 00602 printSf(); 00603 printRxPayloadCrcOn(); 00604 // RegModemStat 00605 printf("ModemStat:0x%02x\r\n", radio.read_reg(REG_LR_MODEMSTAT)); 00606 00607 // fifo ptrs: 00608 lora.RegPayloadLength = radio.read_reg(REG_LR_PAYLOADLENGTH); 00609 lora.RegRxMaxPayloadLength = radio.read_reg(REG_LR_RX_MAX_PAYLOADLENGTH); 00610 printf("fifoptr=0x%02x txbase=0x%02x rxbase=0x%02x payloadLength=0x%02x maxlen=0x%02x", 00611 radio.read_reg(REG_LR_FIFOADDRPTR), 00612 radio.read_reg(REG_LR_FIFOTXBASEADDR), 00613 radio.read_reg(REG_LR_FIFORXBASEADDR), 00614 lora.RegPayloadLength, 00615 lora.RegRxMaxPayloadLength 00616 ); 00617 00618 lora.RegIrqFlags.octet = radio.read_reg(REG_LR_IRQFLAGS); 00619 printLoraIrqs_(false); 00620 00621 lora.RegHopPeriod = radio.read_reg(REG_LR_HOPPERIOD); 00622 if (lora.RegHopPeriod != 0) { 00623 printf("\r\nHopPeriod:0x%02x\r\n", lora.RegHopPeriod); 00624 } 00625 00626 printf("SymbTimeout:0x%03x ", radio.read_u16(REG_LR_MODEMCONFIG2) & 0x3ff); 00627 00628 lora.RegPreamble = radio.read_u16(REG_LR_PREAMBLEMSB); 00629 printf("PreambleLength:0x%03x ", lora.RegPreamble); 00630 00631 00632 if (radio.RegOpMode.bits.Mode == RF_OPMODE_RECEIVER || radio.RegOpMode.bits.Mode == RF_OPMODE_RECEIVER_SINGLE) { 00633 d = radio.read_reg(REG_LR_RSSIVALUE); 00634 printf("rssi:%ddBm ", d-120); 00635 } 00636 00637 printTxContinuousMode(); 00638 00639 printf("\r\n"); 00640 printAgcAutoOn(); 00641 if (radio.type == SX1272) { 00642 printf(" LowDataRateOptimize:%d\r\n", lora.RegModemConfig.sx1272bits.LowDataRateOptimize); 00643 } 00644 00645 printf("\r\nHeaderCount:%d PacketCount:%d, ", 00646 radio.read_u16(REG_LR_RXHEADERCNTVALUE_MSB), radio.read_u16(REG_LR_RXPACKETCNTVALUE_MSB)); 00647 00648 printf("Lora detection threshold:%02x\r\n", radio.read_reg(REG_LR_DETECTION_THRESHOLD)); 00649 lora.RegTest31.octet = radio.read_reg(REG_LR_TEST31); 00650 printf("detect_trig_same_peaks_nb:%d\r\n", lora.RegTest31.bits.detect_trig_same_peaks_nb); 00651 00652 if (radio.type == SX1272) { 00653 lora.RegModemConfig.octet = radio.read_reg(REG_LR_MODEMCONFIG); 00654 printf("LowDataRateOptimize:%d\r\n", lora.RegModemConfig.sx1272bits.LowDataRateOptimize); 00655 } else if (radio.type == SX1276) { 00656 lora.RegModemConfig3.octet = radio.read_reg(REG_LR_MODEMCONFIG3); 00657 printf("LowDataRateOptimize:%d\r\n", lora.RegModemConfig3.sx1276bits.LowDataRateOptimize); 00658 } 00659 00660 printf("\r\n"); 00661 //printf("A %02x\r\n", radio.RegModemConfig2.octet); 00662 } 00663 #endif /* _DEBUG_ */ 00664 00665 /*void 00666 service_radio() 00667 { 00668 service_action_e act = radio.service(); 00669 00670 switch (act) { 00671 case SERVICE_READ_FIFO: 00672 printf("SERVICE_READ_FIFO\r\n"); 00673 // clear Irq flags 00674 radio.write_reg(REG_LR_IRQFLAGS, radio.RegIrqFlags.octet); 00675 break; 00676 case SERVICE_TX_DONE: 00677 printf("SERVICE_TX_DONE\r\n"); 00678 break; 00679 case SERVICE_ERROR: 00680 printf("error\r\n"); 00681 break; 00682 } // ...switch (act) 00683 }*/ 00684 00685 int 00686 main(void) 00687 { 00688 #ifdef _DEBUG_ 00689 pc.baud(57600); 00690 pc.printf("\r\nstart\r\n"); 00691 #endif /* _DEBUG_ */ 00692 00693 //Required for V7 of SX127x library, but V7 doesn't work for this program! 00694 radio.rf_switch.attach(rfsw_callback); 00695 00696 while (1) { 00697 //try to read a msg 00698 if (hid.readNB(&recv_report)) { 00699 HidCmdProcess(); 00700 } 00701 00702 #ifdef _DEBUG_ 00703 if (pc.readable()) { 00704 char c = pc.getc(); 00705 if (c == 'v') { 00706 pc.printf("verbose "); 00707 if (verbose) { 00708 verbose = 0; 00709 pc.printf("off"); 00710 } else { 00711 verbose = 1; 00712 pc.printf("on"); 00713 } 00714 pc.printf("\r\n"); 00715 } else if (c == '.') { 00716 common_print_status(); 00717 if (radio.RegOpMode.bits.LongRangeMode) 00718 lora_print_status(); 00719 else 00720 printf("FSK\r\n"); 00721 } else if (c == 't') { 00722 int i; 00723 printf("tx\r\n"); 00724 radio.set_opmode(RF_OPMODE_TRANSMITTER); 00725 for (i = 0; i < 20; i++) { 00726 radio.RegOpMode.octet = radio.read_reg(REG_OPMODE); 00727 printf("opmode:%02x\r\n", radio.RegOpMode.octet); 00728 } 00729 } else if (c == 'T') { 00730 printf("start_tx\r\n"); 00731 lora.RegPayloadLength = 8; 00732 radio.write_reg(REG_LR_PAYLOADLENGTH, lora.RegPayloadLength); 00733 lora.start_tx(8); 00734 } /*else if (c == 'e') { 00735 printf("service_radio\r\n"); 00736 service_radio(); 00737 }*/ else if (c == 's') { 00738 radio.set_opmode(RF_OPMODE_STANDBY); 00739 printf("standby\r\n"); 00740 } else if (c == 'h') { 00741 printf("hwreset\r\n"); 00742 radio.hw_reset(); 00743 radio.init(); //SX1272Init( ); 00744 } /*else if (c == 'l') { 00745 radio.SetLoRaOn(!radio.RegOpMode.bits.LongRangeMode); 00746 printf("LongRangeMode:%d\r\n", radio.RegOpMode.bits.LongRangeMode); 00747 }*/ else if (c == '?') { 00748 printf("s standby\r\n"); 00749 printf("T lora_start_tx(8)\r\n"); 00750 printf(". print status\r\n"); 00751 printf("v toggle verbose\r\n"); 00752 printf("t tx mode test\r\n"); 00753 printf("e manualy service radio once\r\n"); 00754 printf("h hwreset, init\r\n"); 00755 printf("l toggle lora mode\r\n"); 00756 } 00757 } // ...if (pc.readable()) 00758 #endif /* _DEBUG_ */ 00759 00760 } // ...while (1) 00761 }
Generated on Sun Jul 24 2022 03:26:06 by
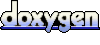