fork of smartwav interface
Fork of SMARTWAV by
Embed:
(wiki syntax)
Show/hide line numbers
SMARTWAV.h
00001 /********************************************************* 00002 VIZIC TECHNOLOGIES. COPYRIGHT 2012. 00003 THE DATASHEETS, SOFTWARE AND LIBRARIES ARE PROVIDED "AS IS." 00004 VIZIC EXPRESSLY DISCLAIM ANY WARRANTY OF ANY KIND, WHETHER 00005 EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO, THE IMPLIED 00006 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, 00007 OR NONINFRINGEMENT. IN NO EVENT SHALL VIZIC BE LIABLE FOR 00008 ANY INCIDENTAL, SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES, 00009 LOST PROFITS OR LOST DATA, HARM TO YOUR EQUIPMENT, COST OF 00010 PROCUREMENT OF SUBSTITUTE GOODS, TECHNOLOGY OR SERVICES, 00011 ANY CLAIMS BY THIRD PARTIES (INCLUDING BUT NOT LIMITED TO 00012 ANY DEFENCE THEREOF), ANY CLAIMS FOR INDEMNITY OR CONTRIBUTION, 00013 OR OTHER SIMILAR COSTS. 00014 *********************************************************/ 00015 00016 /******************************************************** 00017 IMPORTANT : This library is created for the mbed Microcontroller Software IDE 00018 ********************************************************/ 00019 00020 #ifndef SMARTWAV_H 00021 #define SMARTWAV_H 00022 00023 #include <mbed.h> 00024 00025 00026 //******************LIBRARY DEFINED PINS (don't modify)*******************// 00027 //General definitions 00028 #define audioOFF 0 00029 #define audioON 1 00030 #define RESET 13 00031 #define TX 1 00032 #define RX 0 00033 #define DISABLE 0 00034 #define ENABLE 1 00035 #define HALFX 0 00036 #define ONEX 1 00037 #define ONEPOINTFIVEX 2 00038 #define TWOX 3 00039 #define MAX 0xFF 00040 #define MED 0xE0 00041 #define MIN 0x00 00042 00043 00044 //************************************************************************** 00045 // class SMARTWAV SMARTWAV.h 00046 // This is the main class. It shoud be used like this : SMARTWAV audio(p13,p14,p15); 00047 00048 class SMARTWAV{ 00049 00050 public: 00051 00052 SMARTWAV(PinName TXPin, PinName RXPin, PinName resetPin); 00053 00054 void init(); 00055 00056 void reset(); 00057 00058 unsigned char sleep(); 00059 00060 unsigned char getStatus(); 00061 00062 unsigned char playTracks(); 00063 00064 unsigned char pausePlay(); 00065 00066 unsigned char rewindTrack(); 00067 00068 unsigned char nextTrack(); 00069 00070 unsigned char playTrackName(char[]); 00071 00072 unsigned char stopTrack(); 00073 00074 unsigned char continuousPlay(unsigned char); 00075 00076 unsigned char volume(unsigned char); 00077 00078 unsigned char setFolder(char[]); 00079 00080 unsigned char getFileName(char[]); 00081 00082 unsigned char getFolderName(char[]); 00083 00084 unsigned char getFileList(char[]); 00085 00086 unsigned char getFolderList(char[]); 00087 00088 unsigned char playSpeed(unsigned char); 00089 00090 protected : 00091 00092 Serial _serialSMARTWAV; 00093 DigitalOut _resetPin; 00094 00095 }; 00096 typedef unsigned char BYTE; 00097 00098 #endif
Generated on Thu Jul 14 2022 01:13:04 by
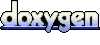