
An I/O controller for virtual pinball machines: accelerometer nudge sensing, analog plunger input, button input encoding, LedWiz compatible output controls, and more.
Dependencies: mbed FastIO FastPWM USBDevice
Fork of Pinscape_Controller by
ticker_api_fix.c
00001 #include <stddef.h> 00002 #include "ticker_api.h" 00003 00004 void $Sub$$ticker_insert_event(const ticker_data_t *const data, ticker_event_t *obj, timestamp_t timestamp, uint32_t id) { 00005 /* disable interrupts for the duration of the function */ 00006 __disable_irq(); 00007 00008 // initialise our data 00009 obj->timestamp = timestamp; 00010 obj->id = id; 00011 00012 /* Go through the list until we either reach the end, or find 00013 an element this should come before (which is possibly the 00014 head). */ 00015 ticker_event_t *prev = NULL, *p = data->queue->head; 00016 while (p != NULL) { 00017 /* check if we come before p */ 00018 if ((int)(timestamp - p->timestamp) < 0) { 00019 break; 00020 } 00021 /* go to the next element */ 00022 prev = p; 00023 p = p->next; 00024 } 00025 00026 /* if we're at the end p will be NULL, which is correct */ 00027 // BUG FIX: do this BEFORE calling set_interrupt(), to ensure 00028 // that the list is in a consistent state if set_interrupt() 00029 // happens to call the event handler, and the event handler 00030 // happens to call back here to re-queue the event. Such 00031 // things aren't hypothetical: this exact thing will happen 00032 // if a Ticker object gets more than one cycle behind. The 00033 // inconsistent state of the list caused crashes. 00034 obj->next = p; 00035 00036 /* if prev is NULL we're at the head */ 00037 if (prev == NULL) { 00038 data->queue->head = obj; 00039 data->interface->set_interrupt(timestamp); 00040 } else { 00041 prev->next = obj; 00042 } 00043 00044 __enable_irq(); 00045 } 00046
Generated on Wed Jul 13 2022 03:30:11 by
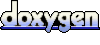