
An I/O controller for virtual pinball machines: accelerometer nudge sensing, analog plunger input, button input encoding, LedWiz compatible output controls, and more.
Dependencies: mbed FastIO FastPWM USBDevice
Fork of Pinscape_Controller by
IRProtocolList.h
00001 // IR Protocol List 00002 // 00003 // This file provides a list of the protocol singletons. It's 00004 // designed to be included multiple times in the compilation. 00005 // On each inclusion, we insert a desired bit of code for each 00006 // of the singletons. We use this to declare all of the singleton 00007 // instances, then to call various methods in all of them. 00008 // 00009 // By convention, the singletons are named s_ClassName. 00010 // 00011 // To use this file, #define ONE of the following macros: 00012 // 00013 // * IR_PROTOCOL_RXTX(className) - define this if you want to include 00014 // expansions for all of the protocol classes, send or receive. This 00015 // includes the dual use classes, the send-only classes, and the 00016 // receive-only classes. 00017 // 00018 // * IR_PROTOCOL_RX(className) - define this if you only want to include 00019 // expansions for RECEIVER protocol classes. This includes dual-purpose 00020 // classes with both sender and receiver, plus the receive-only classes. 00021 // 00022 // * IR_PROTOCOL_TX(className) - define this if you only want to include 00023 // expansions for TRANSMITTER protocol classes. This includes the 00024 // dual-purpose classes with both sender and receiver, plus the 00025 // transmit-only classes. 00026 // 00027 // After #define'ing the desired macro, #include this file. This file 00028 // can be #include'd multiple times in one file for different expansions 00029 // of the list. 00030 00031 00032 // Internally, we use the same three macros: 00033 // 00034 // IR_PROTOCOL_RXTX(className) - define a send/receive class 00035 // IR_PROTOCOL_RX(className) - define a send-only class 00036 // IR_PROTOCOL_TX(className) - define a receive-only class 00037 // 00038 // To set things up, see which one the caller defined, and implicitly 00039 // create expansions for the other two. If the caller wants all classes, 00040 // define _RX and _TX to expand to the same thing as _RXTX. If the caller 00041 // only wants senders, define _RXTX to expand to _TX, and define _RX to 00042 // empty. Vice versa with receive-only. 00043 // 00044 #if defined(IR_PROTOCOL_RXTX) 00045 # define IR_PROTOCOL_RX(cls) IR_PROTOCOL_RXTX(cls) 00046 # define IR_PROTOCOL_TX(cls) IR_PROTOCOL_RXTX(cls) 00047 #elif defined(IR_PROTOCOL_RX) 00048 # define IR_PROTOCOL_RXTX(cls) IR_PROTOCOL_RX(cls) 00049 # define IR_PROTOCOL_TX(cls) 00050 #elif defined(IR_PROTOCOL_TX) 00051 # define IR_PROTOCOL_RXTX(cls) IR_PROTOCOL_TX(cls) 00052 # define IR_PROTOCOL_RX(cls) 00053 #endif 00054 00055 // define the protocol handlers 00056 IR_PROTOCOL_RXTX(IRPNEC_32_48) 00057 IR_PROTOCOL_RXTX(IRPNEC_32x) 00058 IR_PROTOCOL_RXTX(IRPRC5) 00059 IR_PROTOCOL_RXTX(IRPRC6) 00060 IR_PROTOCOL_RXTX(IRPSony) 00061 IR_PROTOCOL_RXTX(IRPDenon) 00062 IR_PROTOCOL_RXTX(IRPKaseikyo) 00063 IR_PROTOCOL_RXTX(IRPSamsung20) 00064 IR_PROTOCOL_RXTX(IRPSamsung36) 00065 IR_PROTOCOL_RXTX(IRPLutron) 00066 IR_PROTOCOL_RXTX(IRPOrtekMCE) 00067 00068 // clear the macros to make way for future definitions 00069 #undef IR_PROTOCOL_RXTX 00070 #undef IR_PROTOCOL_RX 00071 #undef IR_PROTOCOL_TX
Generated on Wed Jul 13 2022 03:30:10 by
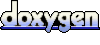