
An I/O controller for virtual pinball machines: accelerometer nudge sensing, analog plunger input, button input encoding, LedWiz compatible output controls, and more.
Dependencies: mbed FastIO FastPWM USBDevice
Fork of Pinscape_Controller by
FreescaleIAP.h
00001 /* 00002 * Freescale FTFA Flash Memory programmer 00003 * 00004 * Sample usage: 00005 00006 #include "mbed.h" 00007 #include "FreescaleIAP.h" 00008 00009 int main() 00010 { 00011 int address = flashSize() - SECTOR_SIZE; //Write in last sector 00012 00013 int *data = (int*)address; 00014 printf("Starting\r\n"); 00015 erase_sector(address); 00016 int numbers[10] = {0, 1, 10, 100, 1000, 10000, 1000000, 10000000, 100000000, 1000000000}; 00017 programFlash(address, (char*)&numbers, 40); //10 integers of 4 bytes each: 40 bytes length 00018 while (true) ; 00019 } 00020 00021 */ 00022 00023 #ifndef FREESCALEIAP_H 00024 #define FREESCALEIAP_H 00025 00026 #include "mbed.h" 00027 00028 #ifdef TARGET_KLXX 00029 #define SECTOR_SIZE 1024 00030 #elif TARGET_K20D5M 00031 #define SECTOR_SIZE 2048 00032 #elif TARGET_K64F 00033 #define SECTOR_SIZE 4096 00034 #else 00035 #define SECTOR_SIZE 1024 00036 #endif 00037 00038 class FreescaleIAP 00039 { 00040 public: 00041 enum IAPCode { 00042 BoundaryError = -99, // Commands may not span several sectors 00043 AlignError, // Data must be aligned on longword (two LSBs zero) 00044 ProtectionError, // Flash sector is protected 00045 AccessError, // Something went wrong 00046 CollisionError, // During writing something tried to flash which was written to 00047 LengthError, // The length must be multiples of 4 00048 RuntimeError, // FTFA runtime error reports 00049 EraseError, // The flash was not erased before writing to it 00050 VerifyError, // The data read back from flash didn't match what we wrote 00051 Success = 0 00052 }; 00053 00054 FreescaleIAP() { } 00055 ~FreescaleIAP() { } 00056 00057 /** Program flash. This erases the area to be written, then writes the data. 00058 * 00059 * @param address starting address where the data needs to be programmed (must be longword alligned: two LSBs must be zero) 00060 * @param data pointer to array with the data to program 00061 * @param length number of bytes to program (must be a multiple of 4) 00062 * @param return Success if no errors were encountered, otherwise one of the error states 00063 */ 00064 IAPCode programFlash(int address, const void *data, unsigned int length); 00065 00066 /** 00067 * Returns size of flash memory 00068 * 00069 * This is the first address which is not flash 00070 * 00071 * @param return length of flash memory in bytes 00072 */ 00073 uint32_t flashSize(void); 00074 00075 private: 00076 // program a word of flash 00077 IAPCode program_word(int address, const char *data); 00078 00079 // verify that a flash area has been erased 00080 IAPCode verify_erased(int address, unsigned int length); 00081 }; 00082 00083 #endif
Generated on Wed Jul 13 2022 03:30:10 by
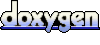