
An input/output controller for virtual pinball machines, with plunger position tracking, accelerometer-based nudge sensing, button input encoding, and feedback device control.
Dependencies: USBDevice mbed FastAnalogIn FastIO FastPWM SimpleDMA
FreescaleIAP.h
00001 /* 00002 * Freescale FTFA Flash Memory programmer 00003 * 00004 * Sample usage: 00005 00006 #include "mbed.h" 00007 #include "FreescaleIAP.h" 00008 00009 int main() { 00010 int address = flash_size() - SECTOR_SIZE; //Write in last sector 00011 00012 int *data = (int*)address; 00013 printf("Starting\r\n"); 00014 erase_sector(address); 00015 int numbers[10] = {0, 1, 10, 100, 1000, 10000, 1000000, 10000000, 100000000, 1000000000}; 00016 program_flash(address, (char*)&numbers, 40); //10 integers of 4 bytes each: 40 bytes length 00017 printf("Resulting flash: \r\n"); 00018 for (int i = 0; i<10; i++) 00019 printf("%d\r\n", data[i]); 00020 00021 printf("Done\r\n\n"); 00022 00023 00024 while (true) { 00025 } 00026 } 00027 00028 */ 00029 00030 #ifndef FREESCALEIAP_H 00031 #define FREESCALEIAP_H 00032 00033 #include "mbed.h" 00034 00035 #ifdef TARGET_KLXX 00036 #define SECTOR_SIZE 1024 00037 #elif TARGET_K20D5M 00038 #define SECTOR_SIZE 2048 00039 #elif TARGET_K64F 00040 #define SECTOR_SIZE 4096 00041 #else 00042 #define SECTOR_SIZE 1024 00043 #endif 00044 00045 enum IAPCode { 00046 BoundaryError = -99, //Commands may not span several sectors 00047 AlignError, //Data must be aligned on longword (two LSBs zero) 00048 ProtectionError, //Flash sector is protected 00049 AccessError, //Something went wrong 00050 CollisionError, //During writing something tried to flash which was written to 00051 LengthError, //The length must be multiples of 4 00052 RuntimeError, 00053 EraseError, //The flash was not erased before writing to it 00054 Success = 0 00055 }; 00056 00057 00058 class FreescaleIAP 00059 { 00060 public: 00061 FreescaleIAP(); 00062 ~FreescaleIAP(); 00063 00064 /** Erase a flash sector 00065 * 00066 * The size erased depends on the used device 00067 * 00068 * @param address address in the sector which needs to be erased 00069 * @param return Success if no errors were encountered, otherwise one of the error states 00070 */ 00071 IAPCode erase_sector(int address); 00072 00073 /** Program flash 00074 * 00075 * Before programming the used area needs to be erased. The erase state is checked 00076 * before programming, and will return an error if not erased. 00077 * 00078 * @param address starting address where the data needs to be programmed (must be longword alligned: two LSBs must be zero) 00079 * @param data pointer to array with the data to program 00080 * @param length number of bytes to program (must be a multiple of 4) 00081 * @param return Success if no errors were encountered, otherwise one of the error states 00082 */ 00083 IAPCode program_flash(int address, const void *data, unsigned int length); 00084 00085 /** 00086 * Returns size of flash memory 00087 * 00088 * This is the first address which is not flash 00089 * 00090 * @param return length of flash memory in bytes 00091 */ 00092 uint32_t flash_size(void); 00093 00094 private: 00095 // program a word of flash 00096 IAPCode program_word(int address, const char *data); 00097 00098 // verify that a flash area has been erased 00099 IAPCode verify_erased(int address, unsigned int length); 00100 }; 00101 00102 #endif
Generated on Fri Jul 15 2022 08:43:32 by
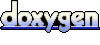