mbed compatible API for the VL53L0X Time-of-Flight sensor
Dependents: VL53L0X_SingleRanging_Example robot_sm VL53L0X_SingleRanging_HighAccuracy_HANSL ENGR6002_P001unk
vl53l0x_platform.cpp
00001 /******************************************************************************* 00002 Copyright � 2015, STMicroelectronics International N.V. 00003 All rights reserved. 00004 00005 Redistribution and use in source and binary forms, with or without 00006 modification, are permitted provided that the following conditions are met: 00007 * Redistributions of source code must retain the above copyright 00008 notice, this list of conditions and the following disclaimer. 00009 * Redistributions in binary form must reproduce the above copyright 00010 notice, this list of conditions and the following disclaimer in the 00011 documentation and/or other materials provided with the distribution. 00012 * Neither the name of STMicroelectronics nor the 00013 names of its contributors may be used to endorse or promote products 00014 derived from this software without specific prior written permission. 00015 00016 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00017 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, AND 00019 NON-INFRINGEMENT OF INTELLECTUAL PROPERTY RIGHTS ARE DISCLAIMED. 00020 IN NO EVENT SHALL STMICROELECTRONICS INTERNATIONAL N.V. BE LIABLE FOR ANY 00021 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00022 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00023 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00024 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00025 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00026 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00027 ********************************************************************************/ 00028 00029 /** 00030 * @file VL53L0X_i2c.c 00031 * 00032 * Copyright (C) 2014 ST MicroElectronics 00033 * 00034 * provide variable word size byte/Word/dword VL6180x register access via i2c 00035 * 00036 */ 00037 00038 #include "vl53l0x_platform.h" 00039 #include "vl53l0x_i2c_platform.h" 00040 #include "vl53l0x_api.h" 00041 00042 #define LOG_FUNCTION_START(fmt, ... ) _LOG_FUNCTION_START(TRACE_MODULE_PLATFORM, fmt, ##__VA_ARGS__) 00043 #define LOG_FUNCTION_END(status, ... ) _LOG_FUNCTION_END(TRACE_MODULE_PLATFORM, status, ##__VA_ARGS__) 00044 #define LOG_FUNCTION_END_FMT(status, fmt, ... ) _LOG_FUNCTION_END_FMT(TRACE_MODULE_PLATFORM, status, fmt, ##__VA_ARGS__) 00045 00046 /** 00047 * @def I2C_BUFFER_CONFIG 00048 * 00049 * @brief Configure Device register I2C access 00050 * 00051 * @li 0 : one GLOBAL buffer \n 00052 * Use one global buffer of MAX_I2C_XFER_SIZE byte in data space \n 00053 * This solution is not multi-Device compliant nor multi-thread cpu safe \n 00054 * It can be the best option for small 8/16 bit MCU without stack and limited ram (STM8s, 80C51 ...) 00055 * 00056 * @li 1 : ON_STACK/local \n 00057 * Use local variable (on stack) buffer \n 00058 * This solution is multi-thread with use of i2c resource lock or mutex see VL6180x_GetI2CAccess() \n 00059 * 00060 * @li 2 : User defined \n 00061 * Per Device potentially dynamic allocated. Requires VL6180x_GetI2cBuffer() to be implemented. 00062 * @ingroup Configuration 00063 */ 00064 #define I2C_BUFFER_CONFIG 1 00065 /** Maximum buffer size to be used in i2c */ 00066 #define VL53L0X_MAX_I2C_XFER_SIZE 64 /* Maximum buffer size to be used in i2c */ 00067 00068 #if I2C_BUFFER_CONFIG == 0 00069 /* GLOBAL config buffer */ 00070 uint8_t i2c_global_buffer[VL53L0X_MAX_I2C_XFER_SIZE]; 00071 00072 #define DECL_I2C_BUFFER 00073 #define VL53L0X_GetLocalBuffer(Dev, n_byte) i2c_global_buffer 00074 00075 #elif I2C_BUFFER_CONFIG == 1 00076 /* ON STACK */ 00077 #define DECL_I2C_BUFFER uint8_t LocBuffer[VL53L0X_MAX_I2C_XFER_SIZE]; 00078 #define VL53L0X_GetLocalBuffer(Dev, n_byte) LocBuffer 00079 #elif I2C_BUFFER_CONFIG == 2 00080 /* user define buffer type declare DECL_I2C_BUFFER as access via VL53L0X_GetLocalBuffer */ 00081 #define DECL_I2C_BUFFER 00082 #else 00083 #error "invalid I2C_BUFFER_CONFIG " 00084 #endif 00085 00086 00087 #define VL53L0X_I2C_USER_VAR /* none but could be for a flag var to get/pass to mutex interruptible return flags and try again */ 00088 #define VL53L0X_GetI2CAccess(Dev) /* todo mutex acquire */ 00089 #define VL53L0X_DoneI2CAcces(Dev) /* todo mutex release */ 00090 00091 VL53L0X_Error VL53L0X_LockSequenceAccess(VL53L0X_DEV Dev){ 00092 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00093 00094 return Status; 00095 } 00096 00097 VL53L0X_Error VL53L0X_UnlockSequenceAccess(VL53L0X_DEV Dev){ 00098 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00099 00100 return Status; 00101 } 00102 00103 // the ranging_sensor_comms.dll will take care of the page selection 00104 VL53L0X_Error VL53L0X_WriteMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count){ 00105 00106 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00107 int32_t status_int = 0; 00108 uint8_t deviceAddress; 00109 00110 if (count>=VL53L0X_MAX_I2C_XFER_SIZE){ 00111 Status = VL53L0X_ERROR_INVALID_PARAMS; 00112 } 00113 00114 deviceAddress = Dev->I2cDevAddr ; 00115 00116 status_int = VL53L0X_write_multi(deviceAddress, index, pdata, count); 00117 00118 if (status_int != 0) 00119 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00120 00121 return Status; 00122 } 00123 00124 // the ranging_sensor_comms.dll will take care of the page selection 00125 VL53L0X_Error VL53L0X_ReadMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count){ 00126 VL53L0X_I2C_USER_VAR 00127 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00128 int32_t status_int; 00129 uint8_t deviceAddress; 00130 00131 if (count>=VL53L0X_MAX_I2C_XFER_SIZE){ 00132 Status = VL53L0X_ERROR_INVALID_PARAMS; 00133 } 00134 00135 deviceAddress = Dev->I2cDevAddr ; 00136 00137 status_int = VL53L0X_read_multi(deviceAddress, index, pdata, count); 00138 00139 if (status_int != 0) 00140 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00141 00142 return Status; 00143 } 00144 00145 00146 VL53L0X_Error VL53L0X_WrByte(VL53L0X_DEV Dev, uint8_t index, uint8_t data){ 00147 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00148 int32_t status_int; 00149 uint8_t deviceAddress; 00150 00151 deviceAddress = Dev->I2cDevAddr ; 00152 00153 status_int = VL53L0X_write_byte(deviceAddress, index, data); 00154 00155 if (status_int != 0) 00156 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00157 00158 return Status; 00159 } 00160 00161 VL53L0X_Error VL53L0X_WrWord(VL53L0X_DEV Dev, uint8_t index, uint16_t data){ 00162 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00163 int32_t status_int; 00164 uint8_t deviceAddress; 00165 00166 deviceAddress = Dev->I2cDevAddr ; 00167 00168 status_int = VL53L0X_write_word(deviceAddress, index, data); 00169 00170 if (status_int != 0) 00171 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00172 00173 return Status; 00174 } 00175 00176 VL53L0X_Error VL53L0X_WrDWord(VL53L0X_DEV Dev, uint8_t index, uint32_t data){ 00177 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00178 int32_t status_int; 00179 uint8_t deviceAddress; 00180 00181 deviceAddress = Dev->I2cDevAddr ; 00182 00183 status_int = VL53L0X_write_dword(deviceAddress, index, data); 00184 00185 if (status_int != 0) 00186 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00187 00188 return Status; 00189 } 00190 00191 VL53L0X_Error VL53L0X_UpdateByte(VL53L0X_DEV Dev, uint8_t index, uint8_t AndData, uint8_t OrData){ 00192 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00193 int32_t status_int; 00194 uint8_t deviceAddress; 00195 uint8_t data; 00196 00197 deviceAddress = Dev->I2cDevAddr ; 00198 00199 status_int = VL53L0X_read_byte(deviceAddress, index, &data); 00200 00201 if (status_int != 0) 00202 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00203 00204 if (Status == VL53L0X_ERROR_NONE) { 00205 data = (data & AndData) | OrData; 00206 status_int = VL53L0X_write_byte(deviceAddress, index, data); 00207 00208 if (status_int != 0) 00209 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00210 } 00211 00212 return Status; 00213 } 00214 00215 VL53L0X_Error VL53L0X_RdByte(VL53L0X_DEV Dev, uint8_t index, uint8_t *data){ 00216 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00217 int32_t status_int; 00218 uint8_t deviceAddress; 00219 00220 deviceAddress = Dev->I2cDevAddr ; 00221 00222 status_int = VL53L0X_read_byte(deviceAddress, index, data); 00223 00224 if (status_int != 0) 00225 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00226 00227 return Status; 00228 } 00229 00230 VL53L0X_Error VL53L0X_RdWord(VL53L0X_DEV Dev, uint8_t index, uint16_t *data){ 00231 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00232 int32_t status_int; 00233 uint8_t deviceAddress; 00234 00235 deviceAddress = Dev->I2cDevAddr ; 00236 00237 status_int = VL53L0X_read_word(deviceAddress, index, data); 00238 00239 if (status_int != 0) 00240 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00241 00242 return Status; 00243 } 00244 00245 VL53L0X_Error VL53L0X_RdDWord(VL53L0X_DEV Dev, uint8_t index, uint32_t *data){ 00246 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00247 int32_t status_int; 00248 uint8_t deviceAddress; 00249 00250 deviceAddress = Dev->I2cDevAddr ; 00251 00252 status_int = VL53L0X_read_dword(deviceAddress, index, data); 00253 00254 if (status_int != 0) 00255 Status = VL53L0X_ERROR_CONTROL_INTERFACE; 00256 00257 return Status; 00258 } 00259 00260 #define VL53L0X_POLLINGDELAY_LOOPNB 250 00261 VL53L0X_Error VL53L0X_PollingDelay(VL53L0X_DEV Dev){ 00262 VL53L0X_Error status = VL53L0X_ERROR_NONE; 00263 volatile uint32_t i; 00264 LOG_FUNCTION_START(""); 00265 00266 for(i=0;i<VL53L0X_POLLINGDELAY_LOOPNB;i++){ 00267 //Do nothing 00268 asm("nop"); 00269 } 00270 00271 LOG_FUNCTION_END(status); 00272 return status; 00273 }
Generated on Wed Jul 13 2022 02:54:36 by
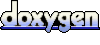