
The space invaders replica (SIR) is console game project written in C++ programming language. The SIR is targeting and works on Outrageous Circuits RETRO game console developer platform. The game is written in C++ programming language using MBED online IDE and compiler.
main.cpp
00001 #include "mbed.h" 00002 00003 00004 /* Display Resultion (128x160) */ 00005 #include "DisplayN18.h" 00006 DisplayN18 disp; 00007 char width = 160, height = 128; 00008 00009 00010 /* Graphic Units */ 00011 // Draw Blank Unit (13x10) 00012 void DrawBlankUnit(int Dx,int Dy) 00013 { 00014 for(int y=-5;y<16;y++) 00015 for(int x=0;x<8;x++) 00016 disp.setPixel(Dy+y,Dx+x,0x0000); 00017 } 00018 00019 // Enemies Visibility and Coordinates 00020 bool EnemyVisible[3][5]; 00021 /* { 00022 {true,true,true,true,true}, 00023 {true,true,true,true,true}, 00024 {true,true,true,true,true} 00025 }; */ 00026 bool FireBullet = false; // Fire Bullet 00027 00028 int EnemyCol[3][5] = { 00029 {10,30,50,70,90}, 00030 {10,30,50,70,90}, 00031 {10,30,50,70,90} 00032 }; 00033 int EnemyRow[3][5] = { 00034 {10,10,10,10,10}, 00035 {20,20,20,20,20}, 00036 {30,30,30,30,30} 00037 }; 00038 // Enemy Unit Part 1 00039 unsigned short Enemy1[8][11] = 00040 { 00041 {0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000}, 00042 {0xffff,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0xffff}, 00043 {0xffff,0x0000,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0x0000,0xffff}, 00044 {0xffff,0xffff,0xffff,0x0000,0xffff,0xffff,0xffff,0x0000,0xffff,0xffff,0xffff}, 00045 {0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff}, 00046 {0x0000,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0x0000}, 00047 {0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000}, 00048 {0x0000,0xffff,0x0000,0x0000,0x0000,0x0000,0x0000,0x0000,0x0000,0xffff,0x0000}, 00049 }; 00050 // Enemy Unit Part 2 00051 unsigned short Enemy2[8][11] = 00052 { 00053 {0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000}, 00054 {0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000}, 00055 {0x0000,0x0000,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0x0000,0x0000}, 00056 {0x0000,0xffff,0xffff,0x0000,0xffff,0xffff,0xffff,0x0000,0xffff,0xffff,0x0000}, 00057 {0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff}, 00058 {0xffff,0x0000,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0x0000,0xffff}, 00059 {0xffff,0x0000,0xffff,0x0000,0x0000,0x0000,0x0000,0x0000,0xffff,0x0000,0xffff}, 00060 {0x0000,0x0000,0x0000,0xffff,0xffff,0x0000,0xffff,0xffff,0x0000,0x0000,0x0000} 00061 }; 00062 // Draw Enemy Unit Part 1 00063 void DrawEnemyUnitPart1(int Dx,int Dy) 00064 { 00065 DrawBlankUnit(Dx,Dy); 00066 for(int y=0;y<11;y++) 00067 for(int x=0;x<8;x++) 00068 if(Enemy1[x][y] != 0x0000) 00069 disp.setPixel(Dy+y,Dx+x,Enemy1[x][y]); 00070 } 00071 // Draw Enemy Unit Part 2 00072 void DrawEnemyUnitPart2(int Dx,int Dy) 00073 { 00074 DrawBlankUnit(Dx,Dy); 00075 for(int y=0;y<11;y++) 00076 for(int x=0;x<8;x++) 00077 if(Enemy2[x][y] != 0x0000) 00078 disp.setPixel(Dy+y,Dx+x,Enemy2[x][y]); 00079 } 00080 // Draw All Visible Enemies 00081 void DrawEnemies(int y,int x) 00082 { 00083 // part 1 00084 if(EnemyVisible[0][0]) DrawEnemyUnitPart1(EnemyRow[0][0]+y,EnemyCol[0][0]+x); 00085 if(EnemyVisible[0][1]) DrawEnemyUnitPart1(EnemyRow[0][1]+y,EnemyCol[0][1]+x); 00086 if(EnemyVisible[0][2]) DrawEnemyUnitPart1(EnemyRow[0][2]+y,EnemyCol[0][2]+x); 00087 if(EnemyVisible[0][3]) DrawEnemyUnitPart1(EnemyRow[0][3]+y,EnemyCol[0][3]+x); 00088 if(EnemyVisible[0][4]) DrawEnemyUnitPart1(EnemyRow[0][4]+y,EnemyCol[0][4]+x); 00089 if(EnemyVisible[1][0]) DrawEnemyUnitPart1(EnemyRow[1][0]+y,EnemyCol[1][0]+x); 00090 if(EnemyVisible[1][1]) DrawEnemyUnitPart1(EnemyRow[1][1]+y,EnemyCol[1][1]+x); 00091 if(EnemyVisible[1][2]) DrawEnemyUnitPart1(EnemyRow[1][2]+y,EnemyCol[1][2]+x); 00092 if(EnemyVisible[1][3]) DrawEnemyUnitPart1(EnemyRow[1][3]+y,EnemyCol[1][3]+x); 00093 if(EnemyVisible[1][4]) DrawEnemyUnitPart1(EnemyRow[1][4]+y,EnemyCol[1][4]+x); 00094 if(EnemyVisible[2][0]) DrawEnemyUnitPart1(EnemyRow[2][0]+y,EnemyCol[2][0]+x); 00095 if(EnemyVisible[2][1]) DrawEnemyUnitPart1(EnemyRow[2][1]+y,EnemyCol[2][1]+x); 00096 if(EnemyVisible[2][2]) DrawEnemyUnitPart1(EnemyRow[2][2]+y,EnemyCol[2][2]+x); 00097 if(EnemyVisible[2][3]) DrawEnemyUnitPart1(EnemyRow[2][3]+y,EnemyCol[2][3]+x); 00098 if(EnemyVisible[2][4]) DrawEnemyUnitPart1(EnemyRow[2][4]+y,EnemyCol[2][4]+x); 00099 // part 2 00100 if(EnemyVisible[0][0]) DrawEnemyUnitPart2(EnemyRow[0][0]+y,EnemyCol[0][0]+x); 00101 if(EnemyVisible[0][1]) DrawEnemyUnitPart2(EnemyRow[0][1]+y,EnemyCol[0][1]+x); 00102 if(EnemyVisible[0][2]) DrawEnemyUnitPart2(EnemyRow[0][2]+y,EnemyCol[0][2]+x); 00103 if(EnemyVisible[0][3]) DrawEnemyUnitPart2(EnemyRow[0][3]+y,EnemyCol[0][3]+x); 00104 if(EnemyVisible[0][4]) DrawEnemyUnitPart2(EnemyRow[0][4]+y,EnemyCol[0][4]+x); 00105 if(EnemyVisible[1][0]) DrawEnemyUnitPart2(EnemyRow[1][0]+y,EnemyCol[1][0]+x); 00106 if(EnemyVisible[1][1]) DrawEnemyUnitPart2(EnemyRow[1][1]+y,EnemyCol[1][1]+x); 00107 if(EnemyVisible[1][2]) DrawEnemyUnitPart2(EnemyRow[1][2]+y,EnemyCol[1][2]+x); 00108 if(EnemyVisible[1][3]) DrawEnemyUnitPart2(EnemyRow[1][3]+y,EnemyCol[1][3]+x); 00109 if(EnemyVisible[1][4]) DrawEnemyUnitPart2(EnemyRow[1][4]+y,EnemyCol[1][4]+x); 00110 if(EnemyVisible[2][0]) DrawEnemyUnitPart2(EnemyRow[2][0]+y,EnemyCol[2][0]+x); 00111 if(EnemyVisible[2][1]) DrawEnemyUnitPart2(EnemyRow[2][1]+y,EnemyCol[2][1]+x); 00112 if(EnemyVisible[2][2]) DrawEnemyUnitPart2(EnemyRow[2][2]+y,EnemyCol[2][2]+x); 00113 if(EnemyVisible[2][3]) DrawEnemyUnitPart2(EnemyRow[2][3]+y,EnemyCol[2][3]+x); 00114 if(EnemyVisible[2][4]) DrawEnemyUnitPart2(EnemyRow[2][4]+y,EnemyCol[2][4]+x); 00115 // wait(0.01); // timeout 00116 } 00117 // Check Enemy Kill 00118 void EnemyKill(int By,int Bx,int Ey,int Ex) 00119 { 00120 for(int row=0;row<3;row++) 00121 for(int col=0;col<5;col++) 00122 if( EnemyVisible[row][col] == true and 00123 By >= EnemyRow[row][col]+Ey and By <= EnemyRow[row][col]+Ey+8 and 00124 Bx >= EnemyCol[row][col]+Ex and Bx <= EnemyCol[row][col]+Ex+11 00125 ) 00126 { 00127 disp.setPixel(Bx,By,0x0000); // hide current bullet 00128 FireBullet = false; // enable next bullet 00129 EnemyVisible[row][col] = false; // disable killed enemy 00130 DrawBlankUnit(EnemyRow[row][col]+Ey,EnemyCol[row][col]+Ex); // hide killed enemy 00131 } 00132 } 00133 00134 // Player Graphics 00135 unsigned short Player[8][11] = 00136 { 00137 {0x0000,0x0000,0x0000,0x0000,0x0000,0xffff,0x0000,0x0000,0x0000,0x0000,0x0000}, 00138 {0x0000,0x0000,0x0000,0x0000,0xffff,0xffff,0xffff,0x0000,0x0000,0x0000,0x0000}, 00139 {0x0000,0x0000,0x0000,0x0000,0xffff,0xffff,0xffff,0x0000,0x0000,0x0000,0x0000}, 00140 {0x0000,0x0000,0x0000,0x0000,0xffff,0xffff,0xffff,0x0000,0x0000,0x0000,0x0000}, 00141 {0x0000,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0x0000}, 00142 {0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff}, 00143 {0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff}, 00144 {0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff,0xffff}, 00145 00146 }; 00147 // Draw Player 00148 void DrawPlayer(int Dx,int Dy) 00149 { 00150 DrawBlankUnit(Dx,Dy); 00151 for(int y=0;y<11;y++) 00152 for(int x=0;x<8;x++) 00153 if(Player[x][y] != 0x0000) 00154 disp.setPixel(Dy+y,Dx+x,Player[x][y]); 00155 } 00156 00157 /* Keyboard */ 00158 DigitalIn LeftButton(P0_14, PullUp); 00159 DigitalIn RightButton(P0_11, PullUp); 00160 DigitalIn FireButton(P0_1, PullUp); 00161 // DigitalIn FireButton(P0_16, PullUp); 00162 char LEFT = 0, RIGHT = 1, UP = 2, DOWN = 3; 00163 00164 /* Dispplay Splash Screen */ 00165 void DisplaySplashScreen() 00166 { 00167 char* info[5] = 00168 { 00169 "Burgas Game Jam 2015", 00170 "Space Inviders Replica", 00171 "by Dimitar Minchev", 00172 "PhD of Informatics", 00173 "Press Fire to Start ..." 00174 }; 00175 // info 00176 disp.drawString(10, 10, info[0], 0xffff, 0x0000, 1); 00177 disp.drawString(10, 30, info[1], 0xffff, 0x0000, 1); 00178 disp.drawString(10, 50, info[2], 0xffff, 0x0000, 1); 00179 disp.drawString(10, 70, info[3], 0xffff, 0x0000, 1); 00180 disp.drawString(10, 90, info[4], 0xffff, 0x0000, 1); 00181 // wait keypress 00182 while (FireButton) wait_ms(1); 00183 disp.clear(); 00184 } 00185 // YouWin 00186 void DisplayYouWin() 00187 { 00188 disp.clear(); 00189 disp.drawString(10, 50, "You Win", 0xffff, 0x0000, 2); 00190 while(true) wait_ms(1); // forever until restart 00191 } 00192 // GameOver 00193 void DisplayGameOver() 00194 { 00195 disp.clear(); 00196 disp.drawString(10, 50, "Game Over", 0xffff, 0x0000, 2); 00197 while(true) wait_ms(1); // forever until restart 00198 } 00199 00200 00201 // Main function 00202 main() 00203 { 00204 // Start 00205 DisplaySplashScreen(); 00206 00207 // vars 00208 bool MoveRight = true; // Movement Direction 00209 int Ex = 0, Ey = 0; // Enemies Coordinates 00210 int Px = 75, Py = 120; // Player Coordinates 00211 int Bx = 80, By = 120; // Bullet Coordinates 00212 00213 // Initialize Emeny Visibility 00214 for(int row=0;row<3;row++) for(int col=0;col<5;col++) EnemyVisible[row][col] = true; 00215 00216 // the loop 00217 while (true) 00218 { 00219 // Buttons 00220 int LeftButtonState = LeftButton.read(); 00221 if( LeftButtonState == 0 ) Px -= 5; 00222 int RightButtonState = RightButton.read(); 00223 if( RightButtonState == 0 ) Px += 5; 00224 int FireButtonState = FireButton.read(); 00225 if(FireButtonState == 0 && FireBullet == false) 00226 { 00227 Bx = Px + 5; 00228 By = Py; 00229 FireBullet = true; 00230 } 00231 00232 // Draw Enemies 00233 DrawEnemies(Ey,Ex); 00234 00235 // Draw Player 00236 DrawPlayer(Py,Px); 00237 00238 // Bullet Movement 00239 if(FireBullet) 00240 { 00241 disp.setPixel(Bx,By,0x0000); 00242 By = By - 5; 00243 disp.setPixel(Bx,By,0xffff); 00244 } 00245 if(By <= 10) // Remove bulet if it is outside 00246 { 00247 disp.setPixel(Bx,By,0x0000); 00248 By = Py; 00249 FireBullet = false; 00250 } 00251 00252 // Check if bullet hit enemy 00253 if(FireBullet) EnemyKill(By,Bx,Ey,Ex); 00254 00255 // Enemies Movement 00256 if(Ex>=60 || Ex<=-6) 00257 { 00258 for(int row=10;row<=30;row+=10) 00259 for(int col=10;col<=90;col+=20) 00260 DrawBlankUnit(row+Ey,col+Ex); 00261 Ey += 10; 00262 } 00263 if(Ex>=60) MoveRight = false; 00264 if(Ex<=-6) MoveRight = true; 00265 if(MoveRight) Ex++; 00266 else Ex--; 00267 00268 // Check Win or Lose 00269 bool win = true; 00270 for(int row=0;row<3;row++) for(int col=0;col<5;col++) if(EnemyVisible[row][col] == true) win = false; 00271 if(win) DisplayYouWin(); 00272 if(Ey >= 120) DisplayGameOver(); 00273 } 00274 }
Generated on Mon Jul 25 2022 04:13:13 by
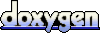