SX1276Lib
Fork of SX1276Lib by
Embed:
(wiki syntax)
Show/hide line numbers
sx1276-hal.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C) 2014 Semtech 00008 00009 Description: - 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: Miguel Luis, Gregory Cristian and Nicolas Huguenin 00014 */ 00015 #ifndef __SX1276_HAL_H__ 00016 #define __SX1276_HAL_H__ 00017 #include "sx1276.h" 00018 00019 /*! 00020 * \brief Radio hardware registers initialization definition 00021 * 00022 * \remark Can be automatically generated by the SX1276 GUI (not yet implemented) 00023 */ 00024 #define RADIO_INIT_REGISTERS_VALUE \ 00025 { \ 00026 { MODEM_FSK , REG_LNA , 0x23 },\ 00027 { MODEM_FSK , REG_RXCONFIG , 0x1E },\ 00028 { MODEM_FSK , REG_RSSICONFIG , 0xD2 },\ 00029 { MODEM_FSK , REG_PREAMBLEDETECT , 0xAA },\ 00030 { MODEM_FSK , REG_OSC , 0x07 },\ 00031 { MODEM_FSK , REG_SYNCCONFIG , 0x12 },\ 00032 { MODEM_FSK , REG_SYNCVALUE1 , 0xC1 },\ 00033 { MODEM_FSK , REG_SYNCVALUE2 , 0x94 },\ 00034 { MODEM_FSK , REG_SYNCVALUE3 , 0xC1 },\ 00035 { MODEM_FSK , REG_PACKETCONFIG1 , 0xD8 },\ 00036 { MODEM_FSK , REG_FIFOTHRESH , 0x8F },\ 00037 { MODEM_FSK , REG_IMAGECAL , 0x02 },\ 00038 { MODEM_FSK , REG_DIOMAPPING1 , 0x00 },\ 00039 { MODEM_FSK , REG_DIOMAPPING2 , 0x30 },\ 00040 { MODEM_LORA, REG_LR_PAYLOADMAXLENGTH, 0x40 },\ 00041 } \ 00042 00043 /*! 00044 * Actual implementation of a SX1276 radio, includes some modifications to make it compatible with the MB1 LAS board 00045 */ 00046 class SX1276MB1xAS : public SX1276 00047 { 00048 protected: 00049 /*! 00050 * Antenna switch GPIO pins objects 00051 */ 00052 DigitalInOut antSwitch ; 00053 00054 DigitalIn fake; 00055 00056 private: 00057 static const RadioRegisters_t RadioRegsInit[]; 00058 00059 public: 00060 SX1276MB1xAS ( RadioEvents_t *events, 00061 PinName mosi, PinName miso, PinName sclk, PinName nss, PinName reset , 00062 PinName dio0 , PinName dio1, PinName dio2, PinName dio3, PinName dio4, PinName dio5, 00063 PinName antSwitch ); 00064 00065 SX1276MB1xAS ( RadioEvents_t *events ); 00066 00067 virtual ~SX1276MB1xAS ( ) { }; 00068 00069 protected: 00070 /*! 00071 * @brief Initializes the radio I/Os pins interface 00072 */ 00073 virtual void IoInit( void ); 00074 00075 /*! 00076 * @brief Initializes the radio registers 00077 */ 00078 virtual void RadioRegistersInit( ); 00079 00080 /*! 00081 * @brief Initializes the radio SPI 00082 */ 00083 virtual void SpiInit( void ); 00084 00085 /*! 00086 * @brief Initializes DIO IRQ handlers 00087 * 00088 * @param [IN] irqHandlers Array containing the IRQ callback functions 00089 */ 00090 virtual void IoIrqInit( DioIrqHandler *irqHandlers ); 00091 00092 /*! 00093 * @brief De-initializes the radio I/Os pins interface. 00094 * 00095 * \remark Useful when going in MCU lowpower modes 00096 */ 00097 virtual void IoDeInit( void ); 00098 00099 /*! 00100 * @brief Gets the board PA selection configuration 00101 * 00102 * @param [IN] channel Channel frequency in Hz 00103 * @retval PaSelect RegPaConfig PaSelect value 00104 */ 00105 virtual uint8_t GetPaSelect( uint32_t channel ); 00106 00107 /*! 00108 * @brief Set the RF Switch I/Os pins in Low Power mode 00109 * 00110 * @param [IN] status enable or disable 00111 */ 00112 virtual void SetAntSwLowPower( bool status ); 00113 00114 /*! 00115 * @brief Initializes the RF Switch I/Os pins interface 00116 */ 00117 virtual void AntSwInit( void ); 00118 00119 /*! 00120 * @brief De-initializes the RF Switch I/Os pins interface 00121 * 00122 * \remark Needed to decrease the power consumption in MCU lowpower modes 00123 */ 00124 virtual void AntSwDeInit( void ); 00125 00126 /*! 00127 * @brief Controls the antena switch if necessary. 00128 * 00129 * \remark see errata note 00130 * 00131 * @param [IN] rxTx [1: Tx, 0: Rx] 00132 */ 00133 virtual void SetAntSw( uint8_t rxTx ); 00134 00135 public: 00136 /*! 00137 * @brief Detect the board connected by reading the value of the antenna switch pin 00138 */ 00139 virtual uint8_t DetectBoardType( void ); 00140 00141 /*! 00142 * @brief Checks if the given RF frequency is supported by the hardware 00143 * 00144 * @param [IN] frequency RF frequency to be checked 00145 * @retval isSupported [true: supported, false: unsupported] 00146 */ 00147 virtual bool CheckRfFrequency( uint32_t frequency ); 00148 00149 /*! 00150 * @brief Writes the radio register at the specified address 00151 * 00152 * @param [IN]: addr Register address 00153 * @param [IN]: data New register value 00154 */ 00155 virtual void Write ( uint8_t addr, uint8_t data ) ; 00156 00157 /*! 00158 * @brief Reads the radio register at the specified address 00159 * 00160 * @param [IN]: addr Register address 00161 * @retval data Register value 00162 */ 00163 virtual uint8_t Read ( uint8_t addr ) ; 00164 00165 /*! 00166 * @brief Writes multiple radio registers starting at address 00167 * 00168 * @param [IN] addr First Radio register address 00169 * @param [IN] buffer Buffer containing the new register's values 00170 * @param [IN] size Number of registers to be written 00171 */ 00172 virtual void Write( uint8_t addr, uint8_t *buffer, uint8_t size ) ; 00173 00174 /*! 00175 * @brief Reads multiple radio registers starting at address 00176 * 00177 * @param [IN] addr First Radio register address 00178 * @param [OUT] buffer Buffer where to copy the registers data 00179 * @param [IN] size Number of registers to be read 00180 */ 00181 virtual void Read ( uint8_t addr, uint8_t *buffer, uint8_t size ) ; 00182 00183 /*! 00184 * @brief Writes the buffer contents to the SX1276 FIFO 00185 * 00186 * @param [IN] buffer Buffer containing data to be put on the FIFO. 00187 * @param [IN] size Number of bytes to be written to the FIFO 00188 */ 00189 virtual void WriteFifo( uint8_t *buffer, uint8_t size ) ; 00190 00191 /*! 00192 * @brief Reads the contents of the SX1276 FIFO 00193 * 00194 * @param [OUT] buffer Buffer where to copy the FIFO read data. 00195 * @param [IN] size Number of bytes to be read from the FIFO 00196 */ 00197 virtual void ReadFifo( uint8_t *buffer, uint8_t size ) ; 00198 00199 /*! 00200 * @brief Reset the SX1276 00201 */ 00202 virtual void Reset( void ); 00203 }; 00204 00205 #endif // __SX1276_HAL_H__
Generated on Wed Jul 13 2022 22:23:26 by
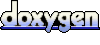