forked RemoteIR
Fork of RemoteIR by
Embed:
(wiki syntax)
Show/hide line numbers
ReceiverIR.h
00001 /** 00002 * IR receiver (Version 0.0.4) 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 #ifndef _RECEIVER_IR_H_ 00009 #define _RECEIVER_IR_H_ 00010 00011 #include <mbed.h> 00012 00013 #include "RemoteIR.h" 00014 00015 /** 00016 * IR receiver class. 00017 */ 00018 class ReceiverIR { 00019 public: 00020 00021 /** 00022 * Constructor. 00023 * 00024 * @param rxpin Pin for receive IR signal. 00025 */ 00026 explicit ReceiverIR(PinName rxpin, bool rxInversion = false); 00027 bool RxInversion; 00028 00029 /** 00030 * Destructor. 00031 */ 00032 ~ReceiverIR(); 00033 00034 /** 00035 * State. 00036 */ 00037 typedef enum { 00038 Idle, 00039 Receiving, 00040 Received 00041 } State; 00042 00043 /** 00044 * Get state. 00045 * 00046 * @return Current state. 00047 */ 00048 State getState(); 00049 00050 /** 00051 * Get data. 00052 * 00053 * @param format Pointer to format. 00054 * @param buf Buffer of a data. 00055 * @param bitlength Bit length of the buffer. 00056 * 00057 * @return Data bit length. 00058 */ 00059 int getData(RemoteIR::Format *format, uint8_t *buf, int bitlength); 00060 00061 private: 00062 00063 typedef struct { 00064 RemoteIR::Format format; 00065 int bitcount; 00066 uint8_t buffer[64]; 00067 } data_t; 00068 00069 typedef struct { 00070 State state; 00071 int c1; 00072 int c2; 00073 int c3; 00074 int d1; 00075 int d2; 00076 } work_t; 00077 00078 InterruptIn evt; /**< Interrupt based input for input. */ 00079 Timer timer; /**< Timer for WDT. */ 00080 Ticker ticker; /**< Tciker for tick. */ 00081 Timeout timeout; /**< Timeout for tail. */ 00082 00083 data_t data; 00084 work_t work; 00085 00086 void init_state(void); 00087 00088 void isr_wdt(void); 00089 void isr_fall(void); 00090 void isr_rise(void); 00091 00092 /** 00093 * ISR timeout for tail detection. 00094 */ 00095 void isr_timeout(void); 00096 00097 }; 00098 00099 #endif
Generated on Thu Jul 21 2022 05:20:31 by
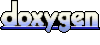