SDL standard library
Embed:
(wiki syntax)
Show/hide line numbers
SDL_syswm.h
Go to the documentation of this file.
00001 /* 00002 Simple DirectMedia Layer 00003 Copyright (C) 1997-2014 Sam Lantinga <slouken@libsdl.org> 00004 00005 This software is provided 'as-is', without any express or implied 00006 warranty. In no event will the authors be held liable for any damages 00007 arising from the use of this software. 00008 00009 Permission is granted to anyone to use this software for any purpose, 00010 including commercial applications, and to alter it and redistribute it 00011 freely, subject to the following restrictions: 00012 00013 1. The origin of this software must not be misrepresented; you must not 00014 claim that you wrote the original software. If you use this software 00015 in a product, an acknowledgment in the product documentation would be 00016 appreciated but is not required. 00017 2. Altered source versions must be plainly marked as such, and must not be 00018 misrepresented as being the original software. 00019 3. This notice may not be removed or altered from any source distribution. 00020 */ 00021 00022 /** 00023 * \file SDL_syswm.h 00024 * 00025 * Include file for SDL custom system window manager hooks. 00026 */ 00027 00028 #ifndef _SDL_syswm_h 00029 #define _SDL_syswm_h 00030 00031 #include "SDL_stdinc.h" 00032 #include "SDL_error.h" 00033 #include "SDL_video.h" 00034 #include "SDL_version.h" 00035 00036 #include "begin_code.h" 00037 /* Set up for C function definitions, even when using C++ */ 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /** 00043 * \file SDL_syswm.h 00044 * 00045 * Your application has access to a special type of event ::SDL_SYSWMEVENT, 00046 * which contains window-manager specific information and arrives whenever 00047 * an unhandled window event occurs. This event is ignored by default, but 00048 * you can enable it with SDL_EventState(). 00049 */ 00050 #ifdef SDL_PROTOTYPES_ONLY 00051 struct SDL_SysWMinfo; 00052 #else 00053 00054 #if defined(SDL_VIDEO_DRIVER_WINDOWS) 00055 #define WIN32_LEAN_AND_MEAN 00056 #include <windows.h> 00057 #endif 00058 00059 /* This is the structure for custom window manager events */ 00060 #if defined(SDL_VIDEO_DRIVER_X11) 00061 #if defined(__APPLE__) && defined(__MACH__) 00062 /* conflicts with Quickdraw.h */ 00063 #define Cursor X11Cursor 00064 #endif 00065 00066 #include <X11/Xlib.h> 00067 #include <X11/Xatom.h> 00068 00069 #if defined(__APPLE__) && defined(__MACH__) 00070 /* matches the re-define above */ 00071 #undef Cursor 00072 #endif 00073 00074 #endif /* defined(SDL_VIDEO_DRIVER_X11) */ 00075 00076 #if defined(SDL_VIDEO_DRIVER_DIRECTFB) 00077 #include <directfb.h> 00078 #endif 00079 00080 #if defined(SDL_VIDEO_DRIVER_COCOA) 00081 #ifdef __OBJC__ 00082 #include <Cocoa/Cocoa.h> 00083 #else 00084 typedef struct _NSWindow NSWindow; 00085 #endif 00086 #endif 00087 00088 #if defined(SDL_VIDEO_DRIVER_UIKIT) 00089 #ifdef __OBJC__ 00090 #include <UIKit/UIKit.h> 00091 #else 00092 typedef struct _UIWindow UIWindow; 00093 #endif 00094 #endif 00095 00096 #if defined(SDL_VIDEO_DRIVER_MIR) 00097 #include <mir_toolkit/mir_client_library.h> 00098 #endif 00099 00100 00101 /** 00102 * These are the various supported windowing subsystems 00103 */ 00104 typedef enum 00105 { 00106 SDL_SYSWM_UNKNOWN, 00107 SDL_SYSWM_WINDOWS, 00108 SDL_SYSWM_X11, 00109 SDL_SYSWM_DIRECTFB, 00110 SDL_SYSWM_COCOA, 00111 SDL_SYSWM_UIKIT, 00112 SDL_SYSWM_WAYLAND, 00113 SDL_SYSWM_MIR, 00114 } SDL_SYSWM_TYPE; 00115 00116 /** 00117 * The custom event structure. 00118 */ 00119 struct SDL_SysWMmsg 00120 { 00121 SDL_version version; 00122 SDL_SYSWM_TYPE subsystem; 00123 union 00124 { 00125 #if defined(SDL_VIDEO_DRIVER_WINDOWS) 00126 struct { 00127 HWND hwnd; /**< The window for the message */ 00128 UINT msg; /**< The type of message */ 00129 WPARAM wParam; /**< WORD message parameter */ 00130 LPARAM lParam; /**< LONG message parameter */ 00131 } win; 00132 #endif 00133 #if defined(SDL_VIDEO_DRIVER_X11) 00134 struct { 00135 XEvent event; 00136 } x11; 00137 #endif 00138 #if defined(SDL_VIDEO_DRIVER_DIRECTFB) 00139 struct { 00140 DFBEvent event; 00141 } dfb; 00142 #endif 00143 #if defined(SDL_VIDEO_DRIVER_COCOA) 00144 struct 00145 { 00146 /* No Cocoa window events yet */ 00147 } cocoa; 00148 #endif 00149 #if defined(SDL_VIDEO_DRIVER_UIKIT) 00150 struct 00151 { 00152 /* No UIKit window events yet */ 00153 } uikit; 00154 #endif 00155 /* Can't have an empty union */ 00156 int dummy; 00157 } msg; 00158 }; 00159 00160 /** 00161 * The custom window manager information structure. 00162 * 00163 * When this structure is returned, it holds information about which 00164 * low level system it is using, and will be one of SDL_SYSWM_TYPE. 00165 */ 00166 struct SDL_SysWMinfo 00167 { 00168 SDL_version version; 00169 SDL_SYSWM_TYPE subsystem; 00170 union 00171 { 00172 #if defined(SDL_VIDEO_DRIVER_WINDOWS) 00173 struct 00174 { 00175 HWND window; /**< The window handle */ 00176 } win; 00177 #endif 00178 #if defined(SDL_VIDEO_DRIVER_X11) 00179 struct 00180 { 00181 Display *display; /**< The X11 display */ 00182 Window window; /**< The X11 window */ 00183 } x11; 00184 #endif 00185 #if defined(SDL_VIDEO_DRIVER_DIRECTFB) 00186 struct 00187 { 00188 IDirectFB *dfb; /**< The directfb main interface */ 00189 IDirectFBWindow *window; /**< The directfb window handle */ 00190 IDirectFBSurface *surface; /**< The directfb client surface */ 00191 } dfb; 00192 #endif 00193 #if defined(SDL_VIDEO_DRIVER_COCOA) 00194 struct 00195 { 00196 NSWindow *window; /* The Cocoa window */ 00197 } cocoa; 00198 #endif 00199 #if defined(SDL_VIDEO_DRIVER_UIKIT) 00200 struct 00201 { 00202 UIWindow *window; /* The UIKit window */ 00203 } uikit; 00204 #endif 00205 #if defined(SDL_VIDEO_DRIVER_WAYLAND) 00206 struct 00207 { 00208 struct wl_display *display; /**< Wayland display */ 00209 struct wl_surface *surface; /**< Wayland surface */ 00210 struct wl_shell_surface *shell_surface; /**< Wayland shell_surface (window manager handle) */ 00211 } wl; 00212 #endif 00213 #if defined(SDL_VIDEO_DRIVER_MIR) 00214 struct 00215 { 00216 MirConnection *connection; /**< Mir display server connection */ 00217 MirSurface *surface; /**< Mir surface */ 00218 } mir; 00219 #endif 00220 00221 /* Can't have an empty union */ 00222 int dummy; 00223 } info; 00224 }; 00225 00226 #endif /* SDL_PROTOTYPES_ONLY */ 00227 00228 typedef struct SDL_SysWMinfo SDL_SysWMinfo; 00229 00230 /* Function prototypes */ 00231 /** 00232 * \brief This function allows access to driver-dependent window information. 00233 * 00234 * \param window The window about which information is being requested 00235 * \param info This structure must be initialized with the SDL version, and is 00236 * then filled in with information about the given window. 00237 * 00238 * \return SDL_TRUE if the function is implemented and the version member of 00239 * the \c info struct is valid, SDL_FALSE otherwise. 00240 * 00241 * You typically use this function like this: 00242 * \code 00243 * SDL_SysWMinfo info; 00244 * SDL_VERSION(&info.version); 00245 * if ( SDL_GetWindowWMInfo(window, &info) ) { ... } 00246 * \endcode 00247 */ 00248 extern DECLSPEC SDL_bool SDLCALL SDL_GetWindowWMInfo(SDL_Window * window, 00249 SDL_SysWMinfo * info); 00250 00251 00252 /* Ends C function definitions when using C++ */ 00253 #ifdef __cplusplus 00254 } 00255 #endif 00256 #include "close_code.h" 00257 00258 #endif /* _SDL_syswm_h */ 00259 00260 /* vi: set ts=4 sw=4 expandtab: */
Generated on Tue Jul 12 2022 13:56:25 by
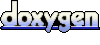