SDL standard library
Embed:
(wiki syntax)
Show/hide line numbers
SDL_joystick.h
Go to the documentation of this file.
00001 /* 00002 Simple DirectMedia Layer 00003 Copyright (C) 1997-2014 Sam Lantinga <slouken@libsdl.org> 00004 00005 This software is provided 'as-is', without any express or implied 00006 warranty. In no event will the authors be held liable for any damages 00007 arising from the use of this software. 00008 00009 Permission is granted to anyone to use this software for any purpose, 00010 including commercial applications, and to alter it and redistribute it 00011 freely, subject to the following restrictions: 00012 00013 1. The origin of this software must not be misrepresented; you must not 00014 claim that you wrote the original software. If you use this software 00015 in a product, an acknowledgment in the product documentation would be 00016 appreciated but is not required. 00017 2. Altered source versions must be plainly marked as such, and must not be 00018 misrepresented as being the original software. 00019 3. This notice may not be removed or altered from any source distribution. 00020 */ 00021 00022 /** 00023 * \file SDL_joystick.h 00024 * 00025 * Include file for SDL joystick event handling 00026 * 00027 * The term "device_index" identifies currently plugged in joystick devices between 0 and SDL_NumJoysticks, with the exact joystick 00028 * behind a device_index changing as joysticks are plugged and unplugged. 00029 * 00030 * The term "instance_id" is the current instantiation of a joystick device in the system, if the joystick is removed and then re-inserted 00031 * then it will get a new instance_id, instance_id's are monotonically increasing identifiers of a joystick plugged in. 00032 * 00033 * The term JoystickGUID is a stable 128-bit identifier for a joystick device that does not change over time, it identifies class of 00034 * the device (a X360 wired controller for example). This identifier is platform dependent. 00035 * 00036 * 00037 */ 00038 00039 #ifndef _SDL_joystick_h 00040 #define _SDL_joystick_h 00041 00042 #include "SDL_stdinc.h" 00043 #include "SDL_error.h" 00044 00045 #include "begin_code.h" 00046 /* Set up for C function definitions, even when using C++ */ 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 /** 00052 * \file SDL_joystick.h 00053 * 00054 * In order to use these functions, SDL_Init() must have been called 00055 * with the ::SDL_INIT_JOYSTICK flag. This causes SDL to scan the system 00056 * for joysticks, and load appropriate drivers. 00057 * 00058 * If you would like to receive joystick updates while the application 00059 * is in the background, you should set the following hint before calling 00060 * SDL_Init(): SDL_HINT_JOYSTICK_ALLOW_BACKGROUND_EVENTS 00061 */ 00062 00063 /* The joystick structure used to identify an SDL joystick */ 00064 struct _SDL_Joystick; 00065 typedef struct _SDL_Joystick SDL_Joystick; 00066 00067 /* A structure that encodes the stable unique id for a joystick device */ 00068 typedef struct { 00069 Uint8 data[16]; 00070 } SDL_JoystickGUID; 00071 00072 typedef Sint32 SDL_JoystickID; 00073 00074 00075 /* Function prototypes */ 00076 /** 00077 * Count the number of joysticks attached to the system right now 00078 */ 00079 extern DECLSPEC int SDLCALL SDL_NumJoysticks(void); 00080 00081 /** 00082 * Get the implementation dependent name of a joystick. 00083 * This can be called before any joysticks are opened. 00084 * If no name can be found, this function returns NULL. 00085 */ 00086 extern DECLSPEC const char *SDLCALL SDL_JoystickNameForIndex(int device_index); 00087 00088 /** 00089 * Open a joystick for use. 00090 * The index passed as an argument refers tothe N'th joystick on the system. 00091 * This index is the value which will identify this joystick in future joystick 00092 * events. 00093 * 00094 * \return A joystick identifier, or NULL if an error occurred. 00095 */ 00096 extern DECLSPEC SDL_Joystick *SDLCALL SDL_JoystickOpen(int device_index); 00097 00098 /** 00099 * Return the name for this currently opened joystick. 00100 * If no name can be found, this function returns NULL. 00101 */ 00102 extern DECLSPEC const char *SDLCALL SDL_JoystickName(SDL_Joystick * joystick); 00103 00104 /** 00105 * Return the GUID for the joystick at this index 00106 */ 00107 extern DECLSPEC SDL_JoystickGUID SDLCALL SDL_JoystickGetDeviceGUID(int device_index); 00108 00109 /** 00110 * Return the GUID for this opened joystick 00111 */ 00112 extern DECLSPEC SDL_JoystickGUID SDLCALL SDL_JoystickGetGUID(SDL_Joystick * joystick); 00113 00114 /** 00115 * Return a string representation for this guid. pszGUID must point to at least 33 bytes 00116 * (32 for the string plus a NULL terminator). 00117 */ 00118 extern DECLSPEC void SDLCALL SDL_JoystickGetGUIDString(SDL_JoystickGUID guid, char *pszGUID, int cbGUID); 00119 00120 /** 00121 * convert a string into a joystick formatted guid 00122 */ 00123 extern DECLSPEC SDL_JoystickGUID SDLCALL SDL_JoystickGetGUIDFromString(const char *pchGUID); 00124 00125 /** 00126 * Returns SDL_TRUE if the joystick has been opened and currently connected, or SDL_FALSE if it has not. 00127 */ 00128 extern DECLSPEC SDL_bool SDLCALL SDL_JoystickGetAttached(SDL_Joystick * joystick); 00129 00130 /** 00131 * Get the instance ID of an opened joystick or -1 if the joystick is invalid. 00132 */ 00133 extern DECLSPEC SDL_JoystickID SDLCALL SDL_JoystickInstanceID(SDL_Joystick * joystick); 00134 00135 /** 00136 * Get the number of general axis controls on a joystick. 00137 */ 00138 extern DECLSPEC int SDLCALL SDL_JoystickNumAxes(SDL_Joystick * joystick); 00139 00140 /** 00141 * Get the number of trackballs on a joystick. 00142 * 00143 * Joystick trackballs have only relative motion events associated 00144 * with them and their state cannot be polled. 00145 */ 00146 extern DECLSPEC int SDLCALL SDL_JoystickNumBalls(SDL_Joystick * joystick); 00147 00148 /** 00149 * Get the number of POV hats on a joystick. 00150 */ 00151 extern DECLSPEC int SDLCALL SDL_JoystickNumHats(SDL_Joystick * joystick); 00152 00153 /** 00154 * Get the number of buttons on a joystick. 00155 */ 00156 extern DECLSPEC int SDLCALL SDL_JoystickNumButtons(SDL_Joystick * joystick); 00157 00158 /** 00159 * Update the current state of the open joysticks. 00160 * 00161 * This is called automatically by the event loop if any joystick 00162 * events are enabled. 00163 */ 00164 extern DECLSPEC void SDLCALL SDL_JoystickUpdate(void); 00165 00166 /** 00167 * Enable/disable joystick event polling. 00168 * 00169 * If joystick events are disabled, you must call SDL_JoystickUpdate() 00170 * yourself and check the state of the joystick when you want joystick 00171 * information. 00172 * 00173 * The state can be one of ::SDL_QUERY, ::SDL_ENABLE or ::SDL_IGNORE. 00174 */ 00175 extern DECLSPEC int SDLCALL SDL_JoystickEventState(int state); 00176 00177 /** 00178 * Get the current state of an axis control on a joystick. 00179 * 00180 * The state is a value ranging from -32768 to 32767. 00181 * 00182 * The axis indices start at index 0. 00183 */ 00184 extern DECLSPEC Sint16 SDLCALL SDL_JoystickGetAxis(SDL_Joystick * joystick, 00185 int axis); 00186 00187 /** 00188 * \name Hat positions 00189 */ 00190 /* @{ */ 00191 #define SDL_HAT_CENTERED 0x00 00192 #define SDL_HAT_UP 0x01 00193 #define SDL_HAT_RIGHT 0x02 00194 #define SDL_HAT_DOWN 0x04 00195 #define SDL_HAT_LEFT 0x08 00196 #define SDL_HAT_RIGHTUP (SDL_HAT_RIGHT|SDL_HAT_UP) 00197 #define SDL_HAT_RIGHTDOWN (SDL_HAT_RIGHT|SDL_HAT_DOWN) 00198 #define SDL_HAT_LEFTUP (SDL_HAT_LEFT|SDL_HAT_UP) 00199 #define SDL_HAT_LEFTDOWN (SDL_HAT_LEFT|SDL_HAT_DOWN) 00200 /* @} */ 00201 00202 /** 00203 * Get the current state of a POV hat on a joystick. 00204 * 00205 * The hat indices start at index 0. 00206 * 00207 * \return The return value is one of the following positions: 00208 * - ::SDL_HAT_CENTERED 00209 * - ::SDL_HAT_UP 00210 * - ::SDL_HAT_RIGHT 00211 * - ::SDL_HAT_DOWN 00212 * - ::SDL_HAT_LEFT 00213 * - ::SDL_HAT_RIGHTUP 00214 * - ::SDL_HAT_RIGHTDOWN 00215 * - ::SDL_HAT_LEFTUP 00216 * - ::SDL_HAT_LEFTDOWN 00217 */ 00218 extern DECLSPEC Uint8 SDLCALL SDL_JoystickGetHat(SDL_Joystick * joystick, 00219 int hat); 00220 00221 /** 00222 * Get the ball axis change since the last poll. 00223 * 00224 * \return 0, or -1 if you passed it invalid parameters. 00225 * 00226 * The ball indices start at index 0. 00227 */ 00228 extern DECLSPEC int SDLCALL SDL_JoystickGetBall(SDL_Joystick * joystick, 00229 int ball, int *dx, int *dy); 00230 00231 /** 00232 * Get the current state of a button on a joystick. 00233 * 00234 * The button indices start at index 0. 00235 */ 00236 extern DECLSPEC Uint8 SDLCALL SDL_JoystickGetButton(SDL_Joystick * joystick, 00237 int button); 00238 00239 /** 00240 * Close a joystick previously opened with SDL_JoystickOpen(). 00241 */ 00242 extern DECLSPEC void SDLCALL SDL_JoystickClose(SDL_Joystick * joystick); 00243 00244 00245 /* Ends C function definitions when using C++ */ 00246 #ifdef __cplusplus 00247 } 00248 #endif 00249 #include "close_code.h" 00250 00251 #endif /* _SDL_joystick_h */ 00252 00253 /* vi: set ts=4 sw=4 expandtab: */
Generated on Tue Jul 12 2022 13:56:24 by
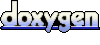