
STM_Schalter
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut Led1(LED1); 00004 DigitalOut Led2(LED2); 00005 DigitalOut Led3(LED3); 00006 DigitalOut Led4(LED4); 00007 DigitalIn SW2(p15); //up 00008 DigitalIn SW3(p12); //down 00009 DigitalIn SW4(p16); //right 00010 InterruptIn SW1(p14); //center 00011 00012 bool pressed =false; 00013 00014 enum State {ST_AUS = 0, ST_EIN}; 00015 00016 State state; 00017 00018 void rise(void) 00019 { 00020 pressed =true; 00021 } 00022 00023 bool CheckFlag() 00024 { 00025 if (pressed) { 00026 pressed = false; 00027 return true; 00028 } 00029 return false; 00030 } 00031 00032 00033 void blink(void) 00034 { 00035 for(int i=0; i<4; i++) { 00036 if(i<4) 00037 Led4=!Led4; 00038 } 00039 } 00040 00041 void ST_Ein(void) 00042 { 00043 while(true) { 00044 Led1 =1; 00045 blink(); 00046 00047 if(CheckFlag()) { 00048 state = ST_AUS; 00049 return; 00050 } 00051 } 00052 } 00053 00054 void ST_Aus(void) 00055 { 00056 while(true) { 00057 Led1 =0; 00058 if (CheckFlag()) { 00059 state = ST_EIN; 00060 return; 00061 } 00062 } 00063 } 00064 00065 void stateMachine(); 00066 00067 00068 00069 00070 int main() 00071 { 00072 00073 SW1.rise(&rise); 00074 while(1) { 00075 stateMachine(); 00076 } 00077 00078 } 00079 00080 void stateMachine() 00081 { 00082 switch (state) { 00083 case ST_AUS: 00084 ST_Aus(); 00085 break; 00086 case ST_EIN: 00087 ST_Ein(); 00088 break; 00089 //default: ST_Error(); 00090 // break; 00091 } 00092 }
Generated on Tue Jul 19 2022 02:03:20 by
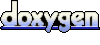