
App to configure a DDS (AD9854) using a K64F. USB an Ethernet were used as interface.
Dependencies: jro mbed AD9854 mbed-rtos I2CLCD k64f_EthLink SerialDriver FreescaleIAP EthernetInterface
main.cpp
00001 /* 00002 * Author: MIGUEL URCO 00003 * Date: 10/11/2014 00004 * Notes: Checks the Ethernet cable connection 00005 */ 00006 #if 1 00007 00008 #include "mbed.h" 00009 #include "rtos.h" 00010 #include "EthernetInterface.h" 00011 #include "SerialDriver.h" 00012 #include "I2CLCD.h" 00013 00014 #include "k64f_EthLink.h" 00015 #include "EthUtils.h" 00016 #include "JroIpdata.h" 00017 #include "JroSIR.h" 00018 #include "AD9854.h" 00019 00020 #define mbed_reset NVIC_SystemReset 00021 00022 #define BUFFER_SIZE 256 00023 static char rx_buffer[BUFFER_SIZE]; 00024 static char tx_buffer[BUFFER_SIZE]; 00025 00026 //THREADS 00027 Thread *ser_thread_ptr; 00028 Thread *eth_thread_ptr; 00029 Thread *lcd_thread_ptr; 00030 Mutex dds_mutex; 00031 Mutex eth_mutex; 00032 Mutex lcd_mutex; 00033 00034 //SERIAL 00035 #define SERIAL_BAUDRATE 1000000 00036 const char* UART_MSG_OK = "DDS Successful"; 00037 const char* UART_MSG_KO = "DDS Unsuccessful"; 00038 const char* UART_MSG_NC = "DDS Not connected"; 00039 00040 Serial screen(USBTX, USBRX); 00041 SerialDriver uart(D1, D0); 00042 00043 00044 //LCD 00045 I2C i2c_device(I2C_SDA, I2C_SCL); 00046 I2CLCD lcd(i2c_device, 0x78, LCD20x2); 00047 00048 //const char LCD_WELCOME[] = " JICAMARCA - DDS "; 00049 const char LCD_IP_INI[] = "IP : checking... "; 00050 const char LCD_IP_NC[] = "IP : Not connected "; 00051 const char LCD_IP_NI[] = "IP : Not initialized"; 00052 const char LCD_IP_RST[] = "IP : Connect&Restart"; 00053 00054 const char LCD_DAC_INI[] = "DAC: checking... "; 00055 const char LCD_DAC_NC[] = "DAC: No detected "; 00056 const char LCD_DAC_NO_CLK[] = "DAC: Verify CLK_IN "; 00057 const char LCD_DAC_ENA[] = " RF Enabled "; 00058 const char LCD_DAC_DIS[] = " RF Disabled "; 00059 00060 //ETHERNET 00061 #define ECHO_SERVER_PORT 2000 00062 const char DEVICE_NAME[] = "jrodds"; 00063 00064 IpData ipData(tx_buffer); 00065 00066 k64fEthLink eth_link; 00067 EthernetInterface eth; 00068 TCPSocketServer server; 00069 00070 int ethIni = 0; 00071 00072 //DDS 00073 SPI spi_device(D11, D12, D13); 00074 00075 DigitalOut dds_mreset(D4); 00076 DigitalOut dds_outramp(D5); 00077 DigitalOut dds_sp_mode(D6); 00078 DigitalOut dds_cs(D7); 00079 DigitalOut dds_io_reset(D9); 00080 DigitalInOut dds_updclk(D10); 00081 00082 DDS dds_device(&spi_device, &dds_mreset, &dds_outramp, &dds_sp_mode, &dds_cs, &dds_io_reset, &dds_updclk); 00083 00084 //LEDS 00085 DigitalOut LedADC(PTB11); 00086 DigitalOut LedUART(PTB10); 00087 DigitalOut LedIP(PTB3); 00088 00089 DigitalIn IPResetButton(PTB2, PullUp); 00090 00091 int printAddr(char *_ip, char *_mask, char *_gateway){ 00092 lcd.printf(_ip, 0, 0); 00093 lcd.printf(_mask, 0, 1); 00094 00095 screen.putc(0x0A); 00096 screen.putc(0x0D); 00097 00098 for (int i=0; i<20; i++){ 00099 screen.putc(_ip[i]); 00100 } 00101 screen.putc(0x0A); 00102 screen.putc(0x0D); 00103 00104 for (int i=0; i<20; i++){ 00105 screen.putc(_mask[i]); 00106 } 00107 00108 screen.putc(0x0A); 00109 screen.putc(0x0D); 00110 00111 for (int i=0; i<20; i++){ 00112 screen.putc(_gateway[i]); 00113 } 00114 00115 screen.putc(0x0A); 00116 screen.putc(0x0D); 00117 00118 return 1; 00119 } 00120 00121 int wasIPResetPressed(){ 00122 00123 if (IPResetButton) 00124 return 0; 00125 00126 //Wait until button will be released 00127 while(!IPResetButton){ 00128 LedADC = 0; 00129 LedIP = 0; 00130 Thread::wait(50); 00131 } 00132 00133 return 1; 00134 } 00135 00136 00137 void waitSerialData_thread(void const *args){ 00138 00139 int n; 00140 bool successful; 00141 00142 //Thread::signal_wait(0x1); 00143 00144 LedUART = 0; 00145 00146 uart.baud(SERIAL_BAUDRATE); 00147 00148 while(1){ 00149 LedUART = 1; 00150 successful = false; 00151 00152 if (uart.isRxBufferEmpty()){ 00153 Thread::wait(100); 00154 continue; 00155 } 00156 00157 Thread::wait(10); 00158 n = uart.read(rx_buffer, 255, false); 00159 00160 //******************** BLINK LED ***************************** 00161 for (int i=0; i<n; i++){ 00162 LedUART = !LedUART; 00163 Thread::wait(10); 00164 } 00165 lcd.printf("Serial command received", 0, 1); 00166 //******************** DDS NOT INITIALIZED ******************* 00167 if (!dds_device.wasInitialized()){ 00168 for (int i=0; i<strlen(UART_MSG_NC); i++){ 00169 uart.putc(UART_MSG_NC[i]); 00170 } 00171 continue; 00172 } 00173 //Lock dds_device before execute any command 00174 dds_mutex.lock(); 00175 00176 //********************* SUCCESSFUL DATA ********************** 00177 if (n == 40) 00178 if (dds_device.writeAllDevice(rx_buffer) == 1) 00179 successful = true; 00180 00181 //******************** REPLY UART***************************** 00182 if (successful) 00183 //for (int i=0; i<strlen(UART_MSG_OK); i++) 00184 uart.printf(UART_MSG_OK); 00185 00186 else 00187 uart.printf(UART_MSG_KO); 00188 00189 dds_mutex.unlock(); 00190 00191 } 00192 00193 } 00194 00195 void waitEthData_thread(void const *args){ 00196 00197 TCPSocketConnection client; 00198 //int status; 00199 int n, totalSize=0; 00200 char _ip[MAX_IP_LEN], _mask[MAX_IP_LEN], _gateway[MAX_IP_LEN]; 00201 00202 //Lock ethernet resource until initial configuration will be loaded 00203 eth_mutex.lock(); 00204 00205 readIpConfig(_ip, _mask, _gateway); 00206 ethIni = 0; 00207 LedIP = 0; 00208 00209 if (eth.init(_ip, _mask, _gateway) != 0){ 00210 //mbed_reset(); 00211 } 00212 00213 eth.setName(DEVICE_NAME); 00214 eth.connect(); 00215 Thread::wait(200); 00216 eth_mutex.unlock(); 00217 00218 //If ethernet connection fails then try again 00219 while(eth_link.GetELink() != 0){ 00220 eth.connect(); 00221 Thread::wait(200); 00222 } 00223 00224 server.bind(ECHO_SERVER_PORT); 00225 server.listen(1); 00226 00227 ethIni = 1; 00228 00229 while(1) 00230 { 00231 LedIP = 1; 00232 n = 0; 00233 totalSize = 0; 00234 00235 server.accept(client); 00236 00237 client.set_blocking(false, 500); // Timeout after (0.5)s 00238 00239 while (true) { 00240 LedIP = !LedIP; 00241 n = client.receive(rx_buffer, sizeof(rx_buffer)); 00242 if (n <= 0) break; 00243 totalSize += n; 00244 Thread::wait(10); 00245 } 00246 00247 LedIP = 1; 00248 00249 if (totalSize < 1){ 00250 client.close(); 00251 continue; 00252 } 00253 00254 if (ipData.decode(rx_buffer, totalSize) == 0){ 00255 client.send(ipData.getKOData(0x00), ipData.getKODataLen()); 00256 client.close(); 00257 continue; 00258 } 00259 lcd.printf("Eth command received", 0, 1); 00260 //******************** DDS NOT INITIALIZED ******************* 00261 00262 if (!dds_device.wasInitialized()){ 00263 client.send(ipData.getNIData(ipData.getCmd()), ipData.getNIDataLen()); 00264 Thread::wait(10); 00265 client.close(); 00266 continue; 00267 } 00268 00269 00270 //******************** REPLY REQ ***************************** 00271 00272 //********* ECHO **** 00273 if (ipData.getCmd() == CMD_ECHO){ 00274 00275 //Sending data 00276 client.send(ipData.getOKData(ipData.getCmd()), ipData.getOKDataLen()); 00277 Thread::wait(10); 00278 client.close(); 00279 00280 continue; 00281 } 00282 00283 //********* IP CONFIG **** 00284 if (ipData.getCmd() == CMD_CHANGE_IP){ 00285 00286 if ( splitIpConf(ipData.getPayload(), _ip, _mask, _gateway) ){ 00287 //changing ip and reseting device 00288 client.send(ipData.getOKData(ipData.getCmd()), ipData.getOKDataLen()); 00289 Thread::wait(200); 00290 client.close(); 00291 00292 saveIpConfig(_ip, _mask, _gateway); 00293 eth.setNewAddr(_ip, _mask, _gateway); 00294 Thread::wait(500); 00295 //mbed_reset(); 00296 continue; 00297 } 00298 else{ 00299 client.send(ipData.getKOData(ipData.getCmd()), ipData.getKODataLen()); 00300 Thread::wait(200); 00301 client.close(); 00302 continue; 00303 } 00304 } 00305 00306 //Lock dds_device before of execute any other command 00307 dds_mutex.lock(); 00308 00309 // ********** OTHER COMMANDS 00310 dds_device.setCommand(ipData.getCmd(), ipData.getPayload(), ipData.getPayloadLen()); 00311 ipData.encode(ipData.getCmd(), dds_device.getCmdAnswer(), dds_device.getCmdAnswerLen()); 00312 00313 client.send(ipData.getTxData(), ipData.getTxDataLen()); 00314 Thread::wait(10); 00315 client.close(); 00316 00317 dds_mutex.unlock(); 00318 } 00319 00320 } 00321 00322 void lcdView_thread(void const *args){ 00323 00324 char c=0; 00325 char lcd_status[2]="\xF7"; 00326 00327 lcd.printf(LCD_DAC_INI, 0, 0); 00328 lcd.printf(LCD_IP_INI, 0, 1); 00329 Thread::wait(2000); 00330 00331 while(1){ 00332 /* 00333 if (lcd_mutex.trylock()==false){ 00334 Thread::wait(1000); 00335 continue; 00336 } 00337 */ 00338 00339 if (dds_device.wasInitialized()){ 00340 00341 //RF ENABLED OR DISABLED 00342 if (c==1){ 00343 if(dds_device.isRFEnabled()){ 00344 lcd.printf(LCD_DAC_ENA, 0, 0); 00345 } 00346 else{ 00347 lcd.printf(LCD_DAC_DIS, 0, 0); 00348 } 00349 lcd_status[0] = 0x01; 00350 lcd.printf(lcd_status, 19, 0); 00351 00352 } 00353 //FREQUENCY 00354 if (c==3){ 00355 char tmp[21]; 00356 sprintf(tmp, "Fout = %7.5f*Clk", dds_device.getFreqFactor1()); 00357 lcd.printf(tmp, 0, 0); 00358 } 00359 if (c==5){ 00360 char tmp[21]; 00361 sprintf(tmp, "Modulation = %s", dds_device.getModeStr() ); 00362 lcd.printf(tmp, 0, 0); 00363 } 00364 } 00365 else{ 00366 if (c==0){ 00367 lcd.printf(LCD_DAC_NC, 0, 0); 00368 } 00369 if (c==3){ 00370 lcd.printf(LCD_DAC_NO_CLK, 0, 0); 00371 } 00372 lcd_status[0] = lcd_status[0] ^ 0xFF; 00373 lcd.printf(lcd_status, 19, 0); 00374 } 00375 00376 //Verifying if ethernet resource is free 00377 if (eth_mutex.trylock()){ 00378 if (eth_link.GetELink() == 0){ 00379 //Eth connected 00380 LedIP = 1; 00381 00382 if (c==0){ 00383 lcd.printf("IP : ", 0, 1); 00384 lcd.printf(eth.getIPAddress(), 5, 1); 00385 } 00386 if (c==2){ 00387 lcd.printf("MSK: ", 0, 1); 00388 lcd.printf(eth.getNetworkMask(), 5, 1); 00389 } 00390 if (c==4){ 00391 lcd.printf("GW : ", 0, 1); 00392 lcd.printf(eth.getGateway(), 5, 1); 00393 } 00394 } 00395 else{ 00396 00397 LedIP = 0; 00398 00399 if (ethIni==0){ 00400 //IP not initialized 00401 if (c==0){ 00402 lcd.printf(LCD_IP_NI, 0, 1); 00403 } 00404 if (c==3){ 00405 lcd.printf(LCD_IP_RST, 0, 1); 00406 } 00407 } 00408 else{ 00409 //IP initialized but unconnected 00410 lcd.printf(LCD_IP_NC, 0, 1); 00411 } 00412 00413 } 00414 eth_mutex.unlock(); 00415 } 00416 else{ 00417 lcd.printf(LCD_IP_INI, 0, 1); 00418 } 00419 c++; 00420 if (c>5) c=0; 00421 //lcd_mutex.unlock(); 00422 Thread::wait(1000); 00423 } 00424 00425 } 00426 00427 int main() 00428 { 00429 LedADC = 0; 00430 00431 screen.baud(9600); 00432 00433 screen.putc(0x0A); 00434 screen.putc(0x0D); 00435 screen.putc(0x33); 00436 screen.putc(0x33); 00437 00438 ser_thread_ptr = new Thread(&waitSerialData_thread); 00439 eth_thread_ptr = new Thread(&waitEthData_thread); 00440 lcd_thread_ptr = new Thread(&lcdView_thread); 00441 00442 screen.putc(0x33); 00443 screen.putc(0x32); 00444 00445 while(true){ 00446 if (dds_device.init()) 00447 break; 00448 00449 LedADC = !LedADC; 00450 Thread::wait(250); 00451 00452 if (wasIPResetPressed()){ 00453 eraseIpConfig(); 00454 mbed_reset(); 00455 } 00456 } 00457 00458 /* 00459 screen.putc(0x33); 00460 screen.putc(0x31); 00461 */ 00462 00463 dds_device.defaultSettings(); 00464 /* 00465 screen.putc(0x33); 00466 screen.putc(0x30); 00467 */ 00468 Thread::wait(1000); 00469 00470 //int c=0; 00471 while(true){ 00472 00473 if (dds_device.isRFEnabled()){ 00474 LedADC = 1; 00475 } 00476 else{ 00477 LedADC = 0; 00478 } 00479 00480 if (wasIPResetPressed()){ 00481 eraseIpConfig(); 00482 mbed_reset(); 00483 } 00484 00485 Thread::wait(200); 00486 } 00487 00488 } 00489 00490 #endif
Generated on Fri Jul 22 2022 04:19:33 by
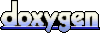