
mbed HRM11017を使ってkonashi.jsでナイトライダー
Dependencies: BLE_API_Native_IRC mbed
Fork of BLE_RCBController by
GattService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef __GATT_SERVICE_H__ 00019 #define __GATT_SERVICE_H__ 00020 00021 #include "blecommon.h" 00022 #include "UUID.h" 00023 #include "GattCharacteristic.h" 00024 00025 #define BLE_SERVICE_MAX_CHARACTERISTICS (5) 00026 00027 /**************************************************************************/ 00028 /*! 00029 \brief GATT service 00030 */ 00031 /**************************************************************************/ 00032 class GattService 00033 { 00034 private: 00035 00036 public: 00037 GattService(uint8_t[16]); /* 128-bit Base UUID */ 00038 GattService(uint16_t); /* 16-bit BLE UUID */ 00039 virtual ~GattService(void); 00040 00041 UUID primaryServiceID; 00042 uint8_t characteristicCount; 00043 GattCharacteristic* characteristics[BLE_SERVICE_MAX_CHARACTERISTICS]; 00044 uint16_t handle; 00045 00046 ble_error_t addCharacteristic(GattCharacteristic &); 00047 00048 enum { 00049 UUID_ALERT_NOTIFICATION_SERVICE = 0x1811, 00050 UUID_BATTERY_SERVICE = 0x180F, 00051 UUID_BLOOD_PRESSURE_SERVICE = 0x1810, 00052 UUID_CURRENT_TIME_SERVICE = 0x1805, 00053 UUID_CYCLING_SPEED_AND_CADENCE = 0x1816, 00054 UUID_DEVICE_INFORMATION_SERVICE = 0x180A, 00055 UUID_GLUCOSE_SERVICE = 0x1808, 00056 UUID_HEALTH_THERMOMETER_SERVICE = 0x1809, 00057 UUID_HEART_RATE_SERVICE = 0x180D, 00058 UUID_HUMAN_INTERFACE_DEVICE_SERVICE = 0x1812, 00059 UUID_IMMEDIATE_ALERT_SERVICE = 0x1802, 00060 UUID_LINK_LOSS_SERVICE = 0x1803, 00061 UUID_NEXT_DST_CHANGE_SERVICE = 0x1807, 00062 UUID_PHONE_ALERT_STATUS_SERVICE = 0x180E, 00063 UUID_REFERENCE_TIME_UPDATE_SERVICE = 0x1806, 00064 UUID_RUNNING_SPEED_AND_CADENCE = 0x1814, 00065 UUID_SCAN_PARAMETERS_SERVICE = 0x1813, 00066 UUID_TX_POWER_SERVICE = 0x1804 00067 }; 00068 }; 00069 00070 #endif
Generated on Tue Jul 12 2022 18:49:54 by
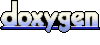