
mbed HRM11017を使ってkonashi.jsでナイトライダー
Dependencies: BLE_API_Native_IRC mbed
Fork of BLE_RCBController by
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVER_H__ 00018 #define __GATT_SERVER_H__ 00019 00020 #include "mbed.h" 00021 #include "blecommon.h" 00022 #include "GattService.h" 00023 #include "GattServerEvents.h" 00024 00025 /**************************************************************************/ 00026 /*! 00027 \brief 00028 The base class used to abstract GATT Server functionality to a specific 00029 radio transceiver, SOC or BLE Stack. 00030 */ 00031 /**************************************************************************/ 00032 class GattServer 00033 { 00034 private: 00035 GattServerEvents *m_pEventHandler; 00036 00037 public: 00038 /* These functions must be defined in the sub-class */ 00039 virtual ble_error_t addService(GattService &) = 0; 00040 virtual ble_error_t readValue(uint16_t, uint8_t[], uint16_t) = 0; 00041 virtual ble_error_t updateValue(uint16_t, uint8_t[], uint16_t) = 0; 00042 00043 // ToDo: For updateValue, check the CCCD to see if the value we are 00044 // updating has the notify or indicate bits sent, and if BOTH are set 00045 // be sure to call sd_ble_gatts_hvx() twice with notify then indicate! 00046 // Strange use case, but valid and must be covered! 00047 00048 /* Event callback handlers */ 00049 void setEventHandler(GattServerEvents *pEventHandler) {m_pEventHandler = pEventHandler;} 00050 void handleEvent(GattServerEvents::gattEvent_e type, uint16_t charHandle) { 00051 if (NULL == m_pEventHandler) 00052 return; 00053 switch(type) { 00054 case GattServerEvents::GATT_EVENT_DATA_SENT: 00055 m_pEventHandler->onDataSent(charHandle); 00056 break; 00057 case GattServerEvents::GATT_EVENT_DATA_WRITTEN: 00058 m_pEventHandler->onDataWritten(charHandle); 00059 break; 00060 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00061 m_pEventHandler->onUpdatesEnabled(charHandle); 00062 break; 00063 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00064 m_pEventHandler->onUpdatesDisabled(charHandle); 00065 break; 00066 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00067 m_pEventHandler->onConfirmationReceived(charHandle); 00068 break; 00069 } 00070 } 00071 00072 uint8_t serviceCount; 00073 uint8_t characteristicCount; 00074 }; 00075 00076 #endif
Generated on Tue Jul 12 2022 18:49:54 by
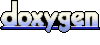