
ADT7410 or TMP102 with mbed HRM1017 sample program konashi.js http://jsdo.it/micutil/7GnE
Dependencies: ADT7410 BLE_API_Native_IRC TMP102 mbed
Fork of BLE_Health_Thermometer_IRC by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2014 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF51822n.h" 00019 00020 nRF51822n nrf; /* BLE radio driver */ 00021 00022 #define UseADT7410 1 00023 #if UseADT7410 00024 #include "ADT7410.h" 00025 ADT7410 healthThemometer(p22, p20, 0x90, 400000); 00026 #else 00027 #include "TMP102.h" 00028 TMP102 healthThemometer(p22, p20, 0x90); /* The TMP102 connected to our board */ 00029 #endif 00030 00031 #define DBG 0 00032 #if DBG 00033 Serial pc(USBTX, USBRX); 00034 #endif 00035 00036 /* LEDs for indication: */ 00037 DigitalOut oneSecondLed(LED1); /* LED1 is toggled every second. */ 00038 DigitalOut advertisingStateLed(LED2); /* LED2 is on when we are advertising, otherwise off. */ 00039 00040 00041 #define KONASHI 1 00042 #if KONASHI 00043 /* Health Thermometer Service */ 00044 uint8_t thermTempPayload[2] = { 0, 0 }; 00045 static const uint16_t konashi_service_uuid = 0xFF00; 00046 static const uint16_t konashi_i2c_read_uuid = 0x300F; 00047 GattService thermService (konashi_service_uuid); 00048 GattCharacteristic thermTemp (konashi_i2c_read_uuid,2, 2, 00049 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE); 00050 #else 00051 /* Health Thermometer Service */ 00052 uint8_t thermTempPayload[5] = { 0, 0, 0, 0, 0 }; 00053 GattService thermService (GattService::UUID_HEALTH_THERMOMETER_SERVICE); 00054 GattCharacteristic thermTemp (GattCharacteristic::UUID_TEMPERATURE_MEASUREMENT_CHAR, 00055 5, 5, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE); 00056 00057 /* Battery Level Service */ 00058 uint8_t batt = 100; /* Battery level */ 00059 uint8_t read_batt = 0; /* Variable to hold battery level reads */ 00060 GattService battService ( GattService::UUID_BATTERY_SERVICE ); 00061 GattCharacteristic battLevel ( GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, 1, 1, 00062 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY | 00063 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00064 00065 #endif 00066 00067 00068 00069 /* Advertising data and parameters */ 00070 GapAdvertisingData advData; 00071 GapAdvertisingData scanResponse; 00072 GapAdvertisingParams advParams ( GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED ); 00073 00074 uint16_t uuid16_list[] = {GattService::UUID_HEALTH_THERMOMETER_SERVICE, 00075 GattService::UUID_BATTERY_SERVICE}; 00076 00077 uint32_t quick_ieee11073_from_float(float temperature); 00078 void updateServiceValues(void); 00079 00080 /**************************************************************************/ 00081 /*! 00082 @brief This custom class can be used to override any GapEvents 00083 that you are interested in handling on an application level. 00084 */ 00085 /**************************************************************************/ 00086 class GapEventHandler : public GapEvents 00087 { 00088 //virtual void onTimeout(void) {} 00089 00090 virtual void onConnected(void) 00091 { 00092 #if DBG 00093 pc.printf("Connected\n\r"); 00094 #endif 00095 advertisingStateLed = 0; 00096 } 00097 00098 /* When a client device disconnects we need to start advertising again. */ 00099 virtual void onDisconnected(void) 00100 { 00101 nrf.getGap().startAdvertising(advParams); 00102 advertisingStateLed = 1; 00103 } 00104 }; 00105 00106 /**************************************************************************/ 00107 /*! 00108 @brief Program entry point 00109 */ 00110 /**************************************************************************/ 00111 int main(void) 00112 { 00113 00114 /* Setup blinky led */ 00115 oneSecondLed=1; 00116 00117 /* Setup an event handler for GAP events i.e. Client/Server connection events. */ 00118 nrf.getGap().setEventHandler(new GapEventHandler()); 00119 00120 /* Initialise the nRF51822 */ 00121 nrf.init(); 00122 00123 /* Make sure we get a clean start */ 00124 nrf.reset(); 00125 00126 /* Add BLE-Only flag and complete service list to the advertising data */ 00127 advData.addFlags(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00128 advData.addData(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, 00129 (uint8_t*)uuid16_list, sizeof(uuid16_list)); 00130 advData.addAppearance(GapAdvertisingData::GENERIC_THERMOMETER); 00131 nrf.getGap().setAdvertisingData(advData, scanResponse); 00132 00133 /* Health Thermometer Service */ 00134 thermService.addCharacteristic(thermTemp); 00135 nrf.getGattServer().addService(thermService); 00136 00137 #if KONASHI 00138 #else 00139 /* Add the Battery Level service */ 00140 battService.addCharacteristic(battLevel); 00141 nrf.getGattServer().addService(battService); 00142 #endif 00143 00144 /* Start advertising (make sure you've added all your data first) */ 00145 nrf.getGap().startAdvertising(advParams); 00146 advertisingStateLed = 1; 00147 00148 for (;;) 00149 { 00150 /* Now that we're live, update the battery level & temperature characteristics */ 00151 updateServiceValues(); 00152 wait(1); 00153 } 00154 } 00155 00156 /**************************************************************************/ 00157 /*! 00158 @brief Ticker callback to switch advertisingStateLed state 00159 */ 00160 /**************************************************************************/ 00161 void updateServiceValues(void) 00162 { 00163 /* Toggle the one second LEDs */ 00164 oneSecondLed = !oneSecondLed; 00165 00166 #if KONASHI 00167 #else 00168 /* Update battery level */ 00169 nrf.getGattServer().updateValue(battLevel.handle, (uint8_t*)&batt, sizeof(batt)); 00170 /* Decrement the battery level. */ 00171 batt <=50 ? batt=100 : batt--;; 00172 #endif 00173 00174 /* Update the temperature. Note that we need to convert to an ieee11073 format float. */ 00175 #if UseADT7410 00176 float temperature = healthThemometer.getTemp(); 00177 #else 00178 float temperature = healthThemometer.read(); 00179 #endif 00180 #if KONASHI 00181 uint16_t temp_ieee11073 = temperature; 00182 //memcpy(thermTempPayload, &temp_ieee11073, 2); 00183 thermTempPayload[0]=floor(temperature); 00184 thermTempPayload[1]=floor(10*(temperature-(float)thermTempPayload[0])); 00185 #else 00186 uint32_t temp_ieee11073 = quick_ieee11073_from_float(temperature); 00187 memcpy(thermTempPayload+1, &temp_ieee11073, 4); 00188 #endif 00189 #if DBG 00190 pc.printf("DATA:%d\n\r",temp_ieee11073); 00191 #endif 00192 nrf.getGattServer().updateValue(thermTemp.handle, thermTempPayload, sizeof(thermTempPayload)); 00193 } 00194 00195 /** 00196 * @brief A very quick conversion between a float temperature and 11073-20601 FLOAT-Type. 00197 * @param temperature The temperature as a float. 00198 * @return The temperature in 11073-20601 FLOAT-Type format. 00199 */ 00200 uint32_t quick_ieee11073_from_float(float temperature) 00201 { 00202 uint8_t exponent = 0xFF; //exponent is -1 00203 uint32_t mantissa = (uint32_t)(temperature*10); 00204 00205 return ( ((uint32_t)exponent) << 24) | mantissa; 00206 } 00207
Generated on Fri Jul 15 2022 11:36:01 by
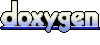