
LWM2M Weather Station
Dependencies: EthernetInterfaceUpdate mbed-rtos mbed nanoservice_client_1_12_X
gas_sensor.cpp
00001 // IPSO Prototype gas sensor resource implementation 00002 00003 #include "mbed.h" 00004 #include "nsdl_support.h" 00005 00006 #define GAS_RES_ID "3300/0/5700" 00007 #define GAS_RES_RT "urn:X-mbed:gas-sensor" 00008 00009 extern Serial pc; 00010 uint8_t gas_max_age = 0; 00011 uint8_t gas_content_type = 50; 00012 00013 AnalogIn gasSensor(A0); 00014 int gas_percent; 00015 char gasPctString[5]; 00016 00017 /* Only GET method allowed */ 00018 static uint8_t gas_sensor_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00019 { 00020 sn_coap_hdr_s *coap_res_ptr = 0; 00021 00022 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) 00023 { 00024 gas_percent = gasSensor.read() * 100; 00025 sprintf(gasPctString,"%d", gas_percent); 00026 pc.printf("gas percent %s\r\n", gasPctString); 00027 00028 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00029 00030 coap_res_ptr->payload_len = strlen(gasPctString); 00031 coap_res_ptr->payload_ptr = (uint8_t*)gasPctString; 00032 00033 coap_res_ptr->content_type_ptr = &gas_content_type; 00034 coap_res_ptr->content_type_len = sizeof(gas_content_type); 00035 00036 coap_res_ptr->options_list_ptr = (sn_coap_options_list_s*)nsdl_alloc(sizeof(sn_coap_options_list_s)); 00037 if(!coap_res_ptr->options_list_ptr) 00038 { 00039 pc.printf("cant alloc option list for max-age\r\n"); 00040 coap_res_ptr->options_list_ptr = NULL; //FIXME report error and recover 00041 } 00042 memset(coap_res_ptr->options_list_ptr, 0, sizeof(sn_coap_options_list_s)); 00043 coap_res_ptr->options_list_ptr->max_age_ptr = &gas_max_age; 00044 coap_res_ptr->options_list_ptr->max_age_len = sizeof(gas_max_age); 00045 00046 sn_nsdl_send_coap_message(address, coap_res_ptr); 00047 nsdl_free(coap_res_ptr->options_list_ptr); 00048 coap_res_ptr->options_list_ptr = NULL; 00049 coap_res_ptr->content_type_ptr = NULL;// parser_release below tries to free this memory 00050 00051 } 00052 00053 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00054 00055 return 0; 00056 } 00057 00058 int create_gas_sensor_resource(sn_nsdl_resource_info_s *resource_ptr) 00059 { 00060 nsdl_create_dynamic_resource(resource_ptr, sizeof(GAS_RES_ID)-1, (uint8_t*)GAS_RES_ID, sizeof(GAS_RES_RT)-1, (uint8_t*)GAS_RES_RT, 0, &gas_sensor_resource_cb, (SN_GRS_GET_ALLOWED)); 00061 return 0; 00062 }
Generated on Tue Jul 12 2022 21:24:03 by
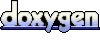