
show 3 axis acceleration, temperature, pressure using FRDM-FXS-MULTI and Nucleo board
Dependencies: FXLS8471Q MPL3115A2 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "FXLS8471Q.h" 00003 #include "MPL3115A2.h" 00004 00005 #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00006 00007 /* NOTE 00008 * Nucleo boards need extra jumpers for this code to function properly. 00009 * See (3) in the "Shields Support Notes" section of http://developer.mbed.org/platforms/ST-Nucleo-F401RE/ 00010 */ 00011 00012 int main() { 00013 // accelerometer 00014 FXLS8471Q acc(D11, D12, D13, D10); 00015 float acc_data[3]; 00016 00017 // temperature/pressure sensor 00018 MPL3115A2 tmp(D14, D15, MPL3115A2_I2C_ADDRESS, D3, D4); 00019 tmp.Barometric_Mode(); 00020 00021 while (true) { 00022 acc.ReadXYZ(acc_data); 00023 printf("x: %1.4f g\ty: %1.4f g\tz: %1.4f g\r\n", acc_data[0], acc_data[1], acc_data[2]); 00024 00025 printf("temperature: %f C\tpressure: %f Pa\r\n", tmp.getTemperature(), tmp.getPressure()); 00026 00027 wait(2); 00028 } 00029 00030 return 0; 00031 }
Generated on Tue Jul 19 2022 23:25:21 by
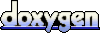