
Median filtered & triggered + buffered RGB readings
Dependencies: mbed rgb_sensor_buffer
main.cpp
00001 /* Discrete RGB color sensor 00002 * 00003 * - uses single-channel light-dependent resistor (via ADC) 00004 * and a RGB LED. 00005 * - compensates background light 00006 * 00007 * Copyright (c) 2014 ARM Limited 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 00022 #include <mbed.h> 00023 #include "rgb_sensor_buffer.h" 00024 00025 #define DEBUG 00026 00027 #define RGB_TRESHOLD 220 00028 #define COUNT(x) (sizeof(x)/sizeof(x[0])) 00029 #define RGB_VALUES 512 00030 00031 /* serial console */ 00032 static TRGB g_buffer[RGB_VALUES]; 00033 static int g_median[RGB_VALUES]; 00034 static Serial console(USBTX, USBRX); 00035 00036 #ifdef DEBUG 00037 static void rgb_print(const TRGB &color) 00038 { 00039 int i; 00040 00041 console.printf("\t["); 00042 for(i=0; i<COUNT(color.data); i++) 00043 console.printf("%s%4i", i?",":"", color.data[i] / RGB_OVERSAMPLING); 00044 console.printf("]"); 00045 } 00046 #endif/*DEBUG*/ 00047 00048 static int median_compare(const void* a, const void *b) 00049 { 00050 return *((int*)a) - *((int*)b); 00051 } 00052 00053 static bool median(TRGB &color, int count) 00054 { 00055 int channel, i; 00056 00057 if(count<=0) 00058 return false; 00059 00060 /* sort all three RGB channels to get median */ 00061 for(channel=0; channel<3; channel++) 00062 { 00063 for(i=0; i<count; i++) 00064 g_median[i] = g_buffer[i].data[channel]; 00065 00066 qsort(&g_median, count, sizeof(g_median[0]), &median_compare); 00067 00068 color.data[channel] = g_median[count/2]; 00069 } 00070 00071 return true; 00072 } 00073 00074 00075 int main() { 00076 int res; 00077 double magnitude; 00078 TRGB color; 00079 00080 console.baud(115200); 00081 00082 /* R,G,B pins and ADC for light dependent resistor */ 00083 RGB_SensorBuffer rgb(p23,p24,p25,p20); 00084 00085 /* needed for time measurement */ 00086 Timer timer; 00087 00088 while(1) { 00089 00090 /* start four channel RGB conversion */ 00091 timer.reset(); 00092 timer.start(); 00093 00094 res = rgb.trigger(g_buffer, COUNT(g_buffer), RGB_TRESHOLD); 00095 00096 /* stop time measurement */ 00097 timer.stop(); 00098 00099 if(res<=0) 00100 { 00101 console.printf("// failed to capture RGB values (%i)\r\n", res); 00102 while(1); 00103 } 00104 00105 /* calculate RGB median */ 00106 median(color, res); 00107 /* print normalized median */ 00108 magnitude = sqrt( 00109 ( (double)color.ch.red * color.ch.red )+ 00110 ( (double)color.ch.green * color.ch.green )+ 00111 ( (double)color.ch.blue * color.ch.blue ) 00112 ); 00113 console.printf("\t[%1.4f,%1.4f,%1.4f,%5i], // %i values in %ims (%i/s)\n\r", 00114 color.ch.red / magnitude, 00115 color.ch.green / magnitude, 00116 color.ch.blue / magnitude, 00117 (int)((magnitude / RGB_OVERSAMPLING)+0.5), 00118 res, 00119 timer.read_ms(), 00120 (res*1000UL)/timer.read_ms() 00121 ); 00122 00123 #ifdef DEBUG 00124 int i; 00125 console.printf("\r\nvar test = [\r\n", 00126 res, 00127 timer.read_ms(), 00128 (res*1000UL)/timer.read_ms()); 00129 00130 for(i=0; i<res; i++) 00131 { 00132 rgb_print(g_buffer[i]); 00133 console.printf(i<(res-1) ? ",\r\n":"];\r\n\r\n"); 00134 } 00135 #endif/*DEBUG*/ 00136 00137 } 00138 }
Generated on Fri Jul 22 2022 13:08:09 by
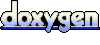