
example for using the rgb_color_sensor class
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* Discrete RGB color sensor 00002 * 00003 * - uses single-channel light-dependent resistor (via ADC) 00004 * and a RGB LED. 00005 * - compensates background light 00006 * 00007 * Copyright (c) 2014 ARM Limited 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 00022 #include <mbed.h> 00023 #include "rgb_sensor.h" 00024 00025 #define COUNT(x) (sizeof(x)/sizeof(x[0])) 00026 #define RGB_VALUES 250 00027 00028 /* serial console */ 00029 static uint32_t g_buffer_pos; 00030 static TRGB g_buffer[RGB_VALUES]; 00031 static Serial console(USBTX, USBRX); 00032 00033 static bool rgb_callback(const TRGB &color) 00034 { 00035 if(g_buffer_pos>=RGB_VALUES) 00036 return false; 00037 00038 g_buffer[g_buffer_pos++] = color; 00039 return true; 00040 } 00041 00042 static void rgb_print(const TRGB &color) 00043 { 00044 int i; 00045 00046 console.printf("\t["); 00047 for(i=0; i<COUNT(color.data); i++) 00048 console.printf("%s%4i", i?",":"", color.data[i] / RGB_OVERSAMPLING); 00049 console.printf("]"); 00050 } 00051 00052 int main() { 00053 int i; 00054 TRGB color; 00055 00056 console.baud(115200); 00057 00058 /* R,G,B pins and ADC for light dependent resistor */ 00059 RGB_Sensor rgb(p23,p24,p25,p20); 00060 00061 /* detect a single RGB value to demo synchronous API */ 00062 rgb.capture(color); 00063 rgb_print(color); 00064 console.printf(";\r\n"); 00065 00066 /* needed for time measurement */ 00067 Timer timer; 00068 00069 g_buffer_pos = 0; 00070 00071 while(1) { 00072 00073 /* start four channel RGB conversion */ 00074 timer.reset(); 00075 timer.start(); 00076 00077 g_buffer_pos = 0; 00078 rgb.capture(rgb_callback); 00079 00080 rgb.wait(); 00081 00082 /* stop time measurement */ 00083 timer.stop(); 00084 00085 console.printf("// captured %i values in %i ms (%i/s)\r\nvar test = [\r\n", 00086 RGB_VALUES, 00087 timer.read_ms(), 00088 (RGB_VALUES*1000UL)/timer.read_ms()); 00089 00090 for(i=0; i<RGB_VALUES; i++) 00091 { 00092 rgb_print(g_buffer[i]); 00093 console.printf(i<(RGB_VALUES-1) ? ",\r\n":"];\r\n\r\n"); 00094 } 00095 00096 /* visible intersection between captures */ 00097 wait_ms(100); 00098 } 00099 }
Generated on Tue Aug 2 2022 21:57:32 by
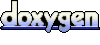