
Test for platforms with only analog inputs not outputs.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 AnalogOut testAnalog(p18); 00004 InterruptIn control(p21); 00005 Serial target (p28,p27); 00006 Serial pc(USBTX, USBRX); 00007 DigitalOut led1(LED1); 00008 DigitalOut led2(LED2); 00009 float value = 0; 00010 00011 void goUp() { 00012 value += 0.1; 00013 testAnalog.write(value); 00014 printf("Value: %.2f\r\n", value); 00015 00016 if (value >= 1.00f) { 00017 value = 0; 00018 } 00019 00020 while (control.read() == 1); 00021 } 00022 00023 int main() { 00024 target.baud(9600); 00025 testAnalog.write(0); 00026 control.rise(&goUp); 00027 00028 printf("Ready. \r\n"); 00029 00030 while(1) { 00031 if (pc.readable()) { 00032 target.putc(pc.getc()); 00033 led1 = !led1; 00034 } 00035 00036 if (target.readable()) { 00037 pc.putc(target.getc()); 00038 led2 = !led2; 00039 } 00040 } 00041 }
Generated on Fri Jul 22 2022 17:12:43 by
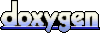