
First Publish
Dependencies: BridgeDriver2 FrontPanelButtons MAX31855 MCP23017 SDFileSystem TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "BridgeDriver.h" 00003 #include "FrontPanelButtons.h" 00004 #include "SDFileSystem.h" 00005 #include "LocalPinNames.h" 00006 #include "TextLCD.h" 00007 #include "max31855.h" 00008 #include <string> 00009 #include <stdio.h> 00010 using std::string; 00011 00012 #include "DS18S20.h" 00013 #include "DS18B20.h" 00014 #include "OneWireDefs.h" 00015 00016 #define THERMOMETER DS18B20 00017 // device( crcOn, useAddress, parasitic, mbed pin ) 00018 THERMOMETER device(true, true, false, p25); 00019 00020 Serial pc(USBTX, USBRX); 00021 00022 Timer timer; // general purpose timer 00023 I2C i2c( P0_10, P0_11 ); // I2C bus (SDA, SCL) 00024 //TextLCD_I2C lcd( &i2c, MCP23008_SA0, TextLCD::lcd20x4 ); // lcd 00025 FrontPanelButtons buttons( &i2c ); // front panel buttons 00026 00027 Timer debounceTimer; 00028 Timer avgCycTimer; 00029 00030 DigitalIn signal(DIO0, PullUp); 00031 CAN can(p9, p10); 00032 00033 //SPI Interfaces 00034 SPI testSPI(P0_9, P0_8, P0_7); // mosi(out), miso(in), sclk(clock) //SPI Bus 00035 //SPI testSPI(P0_9, DIO3, DIO1); // mosi(out), miso(in), sclk(clock) //SPI Bus 00036 00037 00038 CANMessage readBuffer; 00039 int numBuffMsg = 0; 00040 00041 float totaltime = 0; 00042 int cycleCount = 1; 00043 int numCycles = 1000; 00044 00045 float currTemp = 0; //Float value to hold temperature returned 00046 00047 //Function Definitions 00048 void waitSwitch(char [], int); 00049 void waitSwitchRelease(char [], int); 00050 void waitLatch(char [], int); 00051 int checkLatchStatus(); 00052 float getTemp(); 00053 00054 00055 /******************************************************************************/ 00056 /************ CAN Commands *************/ 00057 /******************************************************************************/ 00058 00059 void openDoorCommand(char dataBytes[]){ 00060 dataBytes[3] = 0x03; 00061 can.write(CANMessage(534, dataBytes, 4)); // open the door 00062 wait(0.1); 00063 dataBytes[3] = 0x00; 00064 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00065 } 00066 00067 void closeDoorCommand(char dataBytes[]){ 00068 dataBytes[3] = 0x02; 00069 can.write(CANMessage(534, dataBytes, 4)); // close the door 00070 wait(0.1); 00071 dataBytes[3] = 0x00; 00072 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00073 } 00074 00075 /******************************************************************************/ 00076 /************ Receieve *************/ 00077 /******************************************************************************/ 00078 00079 void receive(){ 00080 CANMessage msg; 00081 if (can.read(msg)){ 00082 if (msg.id == 534){ 00083 readBuffer = msg; 00084 numBuffMsg = 1; 00085 } 00086 } 00087 } 00088 00089 00090 /******************************************************************************/ 00091 /************ Full Init *************/ 00092 /******************************************************************************/ 00093 00094 void fullInit(){ 00095 // i2c.frequency(1000000); 00096 // lcd.setBacklight(TextLCD::LightOn); 00097 // wait(.6); 00098 // lcd.cls(); //clear the display 00099 00100 can.frequency(500000); 00101 can.attach(&receive, CAN::RxIrq); 00102 00103 00104 //********* Init the Thermocouple **********// 00105 00106 while (!device.initialize()); // keep calling until it works 00107 00108 device.setResolution(nineBit); 00109 } 00110 00111 00112 /******************************************************************************/ 00113 /************ waitSwitch *************/ 00114 /******************************************************************************/ 00115 00116 void waitSwitch(char dataBytes[], int openTimeout){ 00117 00118 int openComplete = 0; 00119 while (!openComplete){ 00120 00121 openDoorCommand(dataBytes); //send CAN message to open the door 00122 00123 timer.reset(); 00124 timer.start(); 00125 int flag = 0; 00126 while (!flag){ 00127 if (signal.read() == 0){ 00128 while (!flag){ 00129 debounceTimer.reset(); 00130 debounceTimer.start(); 00131 while (debounceTimer.read_ms() < 40); 00132 if ( signal.read() == 0){ 00133 flag = 1; 00134 openComplete = 1; 00135 } 00136 } 00137 } 00138 else if (timer.read() >= openTimeout) 00139 flag = 1; 00140 } 00141 00142 timer.stop(); 00143 00144 // timeout on opening 00145 if (timer.read() >= openTimeout){ 00146 00147 avgCycTimer.stop(); //pause 00148 00149 dataBytes[3] = 0x01; 00150 can.write(CANMessage(534, dataBytes, 4)); // stop the door 00151 wait(0.1); 00152 dataBytes[3] = 0x00; 00153 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00154 00155 //Error Message 00156 // pc.setAddress ( 0, 1 ); 00157 pc.printf( "<Press Button to Resume>\r\n" ); 00158 // pc.setAddress( 0, 2 ); 00159 pc.printf( "ERROR Open Timeout\r\n" ); 00160 00161 while (!buttons.readSel()); //Do nothing until the button is selected 00162 00163 avgCycTimer.start(); //start 00164 00165 //Remove Error Message 00166 // pc.cls(); //clear the display 00167 00168 // pc.setAddress( 0, 0 ); 00169 pc.printf( "Cycle %d of %3d\r\n", cycleCount, numCycles ); 00170 // pc.setAddress( 0, 1 ); 00171 pc.printf( "Avg t(sec): %1.3f\r\n", (totaltime / cycleCount)); 00172 // pc.setAddress( 0, 2 ); 00173 pc.printf( "STATUS: OPENING\r\n"); 00174 } 00175 } 00176 } 00177 00178 00179 /******************************************************************************/ 00180 /************ Close Door / Switch Release *************/ 00181 /******************************************************************************/ 00182 00183 void waitSwitchRelease(char dataBytes[], int switchReleaseTimeout){ 00184 00185 int switchReleaseComplete = 0; 00186 while (!switchReleaseComplete){ 00187 00188 closeDoorCommand(dataBytes); //send CAN message to close the door 00189 00190 timer.reset(); 00191 timer.start(); 00192 int flag = 0; 00193 while (!flag){ 00194 if (signal.read() == 1){ 00195 while (!flag){ 00196 debounceTimer.reset(); 00197 debounceTimer.start(); 00198 while (debounceTimer.read_ms() < 40); 00199 if ( signal.read() == 1){ 00200 flag = 1; 00201 switchReleaseComplete = 1; 00202 } 00203 } 00204 } 00205 else if (timer.read() >= switchReleaseTimeout) 00206 flag = 1; 00207 } 00208 00209 timer.stop(); 00210 00211 // timeout on switch release 00212 if (timer.read() >= switchReleaseTimeout){ 00213 00214 avgCycTimer.stop(); //pause 00215 00216 dataBytes[3] = 0x01; 00217 can.write(CANMessage(534, dataBytes, 4)); // stop the door 00218 wait(0.1); 00219 dataBytes[3] = 0x00; 00220 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00221 00222 //Error Message 00223 // pc.setAddress ( 0, 1 ); 00224 pc.printf( "<Press Sel to Resume>\r\n" ); 00225 // pc.setAddress( 0, 2 ); 00226 pc.printf( "ERROR Release Switch Timeout\r\n" ); 00227 00228 while (!buttons.readSel()); //Do nothing until the button is selected 00229 00230 avgCycTimer.start(); //start 00231 00232 //Remove Error Message 00233 // pc.cls(); //clear the display 00234 00235 // pc.setAddress( 0, 0 ); 00236 pc.printf( "Cycle %d of %3d\r\n", cycleCount, numCycles ); 00237 // pc.setAddress( 0, 1 ); 00238 pc.printf( "Avg t(sec): %1.3f\r\n", (totaltime / cycleCount)); 00239 // pc.setAddress( 0, 2 ); 00240 pc.printf( "STATUS: SWITCH\r\n"); 00241 } 00242 } 00243 } 00244 00245 00246 /******************************************************************************/ 00247 /************ Close Door to Latch *************/ 00248 /******************************************************************************/ 00249 00250 void waitLatch(char dataBytes[], int closeTimeout){ 00251 00252 //Loop through the close sequence until the latch is closed 00253 int closeComplete = 0; 00254 while (!closeComplete){ 00255 00256 closeDoorCommand(dataBytes); //send CAN message to close the door 00257 00258 timer.reset(); 00259 timer.start(); 00260 int flag = 0; 00261 while (!flag){ 00262 00263 if (checkLatchStatus()){ 00264 flag = 1; 00265 closeComplete = 1; 00266 } 00267 00268 //if the timer goes off 00269 else if (timer.read() >= closeTimeout) 00270 flag = 1; 00271 } 00272 00273 timer.stop(); 00274 00275 // timeout on closing 00276 if (timer.read() >= closeTimeout){ 00277 00278 avgCycTimer.stop(); //pause 00279 00280 //Error Message 00281 // pc.setAddress ( 0, 1 ); 00282 pc.printf( "<Press Sel to Resume>\r\n" ); 00283 // pc.setAddress( 0, 2 ); 00284 pc.printf( "ERROR Close Timeout\r\n" ); 00285 00286 dataBytes[3] = 0x01; 00287 can.write(CANMessage(534, dataBytes, 4)); // stop the door 00288 wait(0.1); 00289 dataBytes[3] = 0x00; 00290 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00291 00292 while (!buttons.readSel()); //Do nothing until the button is selected 00293 00294 avgCycTimer.start(); //start 00295 00296 //Remove Error Message 00297 // pc.cls(); //clear the display 00298 00299 // pc.setAddress( 0, 0 ); 00300 pc.printf( "Cycle %d of %3d\r\n", cycleCount, numCycles ); 00301 // pc.setAddress( 0, 1 ); 00302 pc.printf( "Avg t(sec): %1.3f\r\n", (totaltime / cycleCount)); 00303 // pc.setAddress( 0, 2 ); 00304 pc.printf( "STATUS: CLOSING\r\n"); 00305 } 00306 } 00307 } 00308 00309 00310 /******************************************************************************/ 00311 /************ Check Latch Status *************/ 00312 /******************************************************************************/ 00313 00314 // Returns 1 if latch is closed, 0 if it is not closed 00315 int checkLatchStatus(){ 00316 00317 //convert the data array into a single 64 bit value so that we can grab the range of bits we desire, no matter where they're located 00318 long long allData = 0; 00319 for (int j = 0; j < 8; j++){ 00320 long long tempData1 = (long long)readBuffer.data[j]; 00321 tempData1 <<= (8 * j); //shift data bits into there proper position in the 64 bit long long 00322 allData |= tempData1; 00323 } 00324 00325 int _startBitValue = 4, _numBitsValue = 4; //get the latch status bits 00326 //isolate the desired range of bits for comparison 00327 // (_numBitsValue - 1) makes it so the following, startBit = 13, numBites = 5 would mean you want up to bit 17, but (13 + 5) brings you to 18, therefore do (numBits - 1) 00328 int compareBits = (allData >> _startBitValue) & ~(~0 << ((_startBitValue+(_numBitsValue-1))-_startBitValue+1)); 00329 00330 int compareValue = 0; //value to watch (i.e. 0 for latch closed) 00331 00332 //if the latch is closed 00333 if (compareBits == compareValue) 00334 return 1; 00335 00336 return 0; 00337 } 00338 00339 00340 /******************************************************************************/ 00341 /************ getTemp *************/ 00342 /******************************************************************************/ 00343 00344 float getTemp(){ 00345 00346 return device.readTemperature(); 00347 } 00348 00349 /******************************************************************************/ 00350 /************ Main *************/ 00351 /******************************************************************************/ 00352 00353 int main() { 00354 00355 fullInit(); 00356 00357 int openTimeout = 10; //10sec timeout 00358 int switchReleaseTimeout = 2; 00359 int closeTimeout = 10; 00360 00361 char dataBytes[4]; 00362 dataBytes[0] = 0x00; 00363 dataBytes[1] = 0x00; 00364 dataBytes[2] = 0x00; 00365 00366 00367 // pc.setAddress ( 0, 3 ); 00368 pc.printf( "<Press Button to start>\r\n" ); 00369 00370 00371 // while (!buttons.readSel()){ 00372 // if(buttons.readUp() && numCycles < 999999 ){ 00373 // numCycles = numCycles + 100; 00374 // wait(0.2); 00375 // } 00376 // else if (buttons.readDown() && numCycles > 0 ){ 00377 // numCycles = numCycles - 100; 00378 // wait(0.2); 00379 // } 00380 // pc.setAddress ( 0, 0 ); 00381 // pc.printf( "<Num Cycles:%5d >" , numCycles ); 00382 // } 00383 00384 // pc.cls(); //clear the display 00385 00386 // while(1){ 00387 // currTemp = getTemp(); 00388 // printf("%f",currTemp); 00389 // wait(1); 00390 // } 00391 00392 /******************************************************************************/ 00393 /************ Cycle Loop *************/ 00394 /******************************************************************************/ 00395 00396 while (cycleCount <= numCycles){ 00397 00398 // pc.setAddress( 0, 0 ); 00399 pc.printf( "Cycle %d of %3d\r\n", cycleCount, numCycles ); 00400 avgCycTimer.reset(); 00401 avgCycTimer.start(); 00402 00403 00404 /******************************************************************************/ 00405 /************ Open *************/ 00406 /******************************************************************************/ 00407 00408 openDoorCommand(dataBytes); 00409 00410 // pc.setAddress( 0, 2 ); 00411 pc.printf( "STATUS: OPENING\r\n"); 00412 00413 waitSwitch(dataBytes, openTimeout); 00414 00415 /******************************************************************************/ 00416 /************ waitSwitchRelease *************/ 00417 /******************************************************************************/ 00418 00419 closeDoorCommand(dataBytes); 00420 00421 // pc.setAddress( 0, 2 ); 00422 pc.printf( "STATUS: SWITCH\r\n"); 00423 00424 waitSwitchRelease(dataBytes, switchReleaseTimeout); 00425 00426 /******************************************************************************/ 00427 /************ waitSwitchRelease *************/ 00428 /******************************************************************************/ 00429 00430 // pc.setAddress( 0, 2 ); 00431 pc.printf( "STATUS: CLOSING\r\n"); 00432 00433 waitLatch(dataBytes, closeTimeout); 00434 00435 /******************************************************************************/ 00436 /************ End Cycle *************/ 00437 /******************************************************************************/ 00438 00439 totaltime += avgCycTimer.read(); 00440 // pc.setAddress( 0, 1 ); 00441 pc.printf( "Avg t(sec): %1.3f\r\n", (totaltime / cycleCount)); 00442 wait(0.2); 00443 cycleCount++; 00444 } 00445 } 00446 00447 00448 00449 00450 00451 00452 00453 00454 00455 00456 00457 00458 00459 00460 /* 00461 pc.setAddress ( 0, 0 ); 00462 pc.printf( "^ Up = Open" ); 00463 pc.setAddress ( 0, 1 ); 00464 pc.printf( "v Down = Close" ); 00465 pc.setAddress ( 0, 2 ); 00466 pc.printf( "> Right = Stop" ); 00467 00468 while(1){ 00469 if (buttons.readUp()){ 00470 dataBytes[3] = 0x03; 00471 can.write(CANMessage(534, dataBytes, 4)); // open the door 00472 wait(0.1); 00473 dataBytes[3] = 0x00; 00474 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00475 } 00476 else if (buttons.readDown()){ 00477 dataBytes[3] = 0x02; 00478 can.write(CANMessage(534, dataBytes, 4)); // close the door 00479 wait(0.1); 00480 dataBytes[3] = 0x00; 00481 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00482 } 00483 else if (buttons.readRight()){ 00484 dataBytes[3] = 0x01; 00485 can.write(CANMessage(534, dataBytes, 4)); // stop the door 00486 wait(0.1); 00487 dataBytes[3] = 0x00; 00488 can.write(CANMessage(534, dataBytes, 4)); // Set to IDLE 00489 } 00490 }*/ 00491 /* 00492 while (1){ 00493 //convert the data array into a single 64 bit value so that we can grab the range of bits we desire, no matter where they're located 00494 long long allData = 0; //((long long)*readMsg[k].data); 00495 for (int j = 0; j < 8; j++){ 00496 long long tempData1 = (long long)readBuffer.data[j]; 00497 tempData1 <<= (8 * j); //shift data bits into there proper position in the 64 bit long long 00498 allData |= tempData1; 00499 } 00500 00501 int _startBitValue = 4, _numBitsValue = 4; //get the latch status bits 00502 //isolate the desired range of bits for comparison 00503 // (_numBitsValue - 1) makes it so the following, startBit = 13, numBites = 5 would mean you want up to bit 17, but (13 + 5) brings you to 18, therefore do (numBits - 1) 00504 int compareBits = (allData >> _startBitValue) & ~(~0 << ((_startBitValue+(_numBitsValue-1))-_startBitValue+1)); 00505 00506 int compareValue = 0; //value to watch (i.e. 0 for latch closed) 00507 //if the latch is closed 00508 if (compareBits == compareValue){ 00509 pc.setAddress ( 0, 0 ); 00510 pc.printf( "Latch: Closed " ); 00511 } 00512 else{ 00513 pc.setAddress ( 0, 0 ); 00514 pc.printf( "Latch: Not Closed " ); 00515 } 00516 }*/
Generated on Wed Jul 20 2022 22:06:16 by
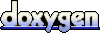