
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
mainFunctions.cpp
00001 //#include "mainFunctions.hpp" 00002 // 00003 ///******************************************************************************/ 00004 ///*** <Function: cyclePrograms> ***/ 00005 ///******************************************************************************/ 00006 // 00007 //int cyclePrograms(vector<string> files, int SIZE, int currIndex, int direction){ 00008 // 00009 // int nextIndex = 0; 00010 // switch(direction){ 00011 // case 0: //Cycle Back one File 00012 // if ((currIndex - 1) < 0) 00013 // nextIndex = SIZE - 1; 00014 // else 00015 // nextIndex = currIndex - 1; 00016 // break; 00017 // case 1: //Cycle Forward one File 00018 // if ((currIndex + 1) >= SIZE) 00019 // nextIndex = 0; 00020 // else 00021 // nextIndex = currIndex + 1; 00022 // break; 00023 // case -1: //set the selectedFile to the currIndex (used for initialization) 00024 // nextIndex = currIndex; 00025 // break; 00026 // } 00027 // 00028 // //Output file on Display 00029 // lcd.setAddress(0,3); 00030 // lcd.printf(" "); // Clear the Line using Spaces (Emptyness) - Note one line is 20 Characters 00031 // wait(.2); 00032 // lcd.setAddress(0,3); 00033 // lcd.printf("%s", files[nextIndex]); 00034 // 00035 // return nextIndex; // Return the file index in the Array 00036 //} 00037 // 00038 ///******************************************************************************/ 00039 ///*** <Function: resetLineData> ***/ 00040 ///******************************************************************************/ 00041 // 00042 //void resetLineData(LineData &lineData){ 00043 // 00044 // lineData.lineNumber = 0; 00045 // lineData.numWords = 0; 00046 // lineData.lineAddress = 0; 00047 //} 00048 // 00049 ///******************************************************************************/ 00050 ///*** <Function: interpretCommand> ***/ 00051 ///******************************************************************************/ 00052 // 00053 //int interpretCommand(FILE *selectedFile, LineData &lineData){ 00054 // 00055 // if (lineData.word[0].compare("device") == 0){ 00056 // 00057 // int i = 0, deviceFound = -1; 00058 // for (i = 0; i < numDevices; i++){ 00059 // if (lineData.word[2].compare(DeviceNames[i]) == 0){ 00060 // deviceFound = i; 00061 // } 00062 // } 00063 // 00064 // //if the device type does not match any known type, error out 00065 // if (deviceFound == -1){ 00066 // //Error Out since the device Name was not matched with anything ************************* 00067 // } 00068 // 00069 // //Add device to the array of devices and initialize it 00070 // else{ 00071 // devices.push_back(Device::newDevice(deviceFound, lineData.word[1], lineData)); 00072 // devices.back()->name = lineData.word[1]; 00073 // } 00074 // } 00075 // 00076 // else if (lineData.word[0].compare("delay") == 0){ 00077 // string duration = lineData.word[1]; 00078 // int durationValue = 0; 00079 // sscanf(duration.c_str(), "%d", &durationValue); 00080 // 00081 // if (durationValue){ 00082 // timer.reset(); 00083 // timer.start(); 00084 // while (timer.read_ms() < durationValue); //Do Nothing while the timer has not reached the duration 00085 // timer.stop(); //Stop the Timer 00086 // } 00087 // else{ 00088 // //Error Out 00089 // return -1; 00090 // } 00091 // } 00092 // 00093 // else if (lineData.word[0].compare("loop") == 0){ 00094 // int checkLoopEnd = loopCommand(selectedFile, lineData); 00095 // if (checkLoopEnd == 1) 00096 // return 1; 00097 // } 00098 // 00099 // else if (lineData.word[0].compare("condition") == 0){ 00100 // int checkLoopEnd = conditionCommand(selectedFile, lineData); 00101 // if (checkLoopEnd == 1) 00102 // return 2; 00103 // } 00104 // // end with custom return value for specific function 00105 // else if (lineData.word[0].compare("end") == 0){ 00106 // if (lineData.word[1].compare("program") == 0){ 00107 // return 2; 00108 // } 00109 // else if (lineData.word[1].compare("loop") == 0){ 00110 // return 3; 00111 // } 00112 // else if (lineData.word[1].compare("condition") == 0){ 00113 // return 4; 00114 // } 00115 // } 00116 // 00117 // //not a keyword so check if it's a localName for a device 00118 // else{ 00119 // 00120 // int i = 0, deviceFound = -1; 00121 // for (i = 0; i < devices.size(); i++){ 00122 // if (lineData.word[0].compare(devices[i]->name) == 0) 00123 // deviceFound = i; 00124 // } 00125 // 00126 // //no device was found that matched the local name, and this is also the last error check, meaning it can match no other potential keywords 00127 // if (deviceFound == -1){ 00128 // lcd.setAddress(0,3); 00129 // lcd.printf("Final ERROR!"); 00130 // wait(10); 00131 // } 00132 // 00133 // //Local Name matches a device, send line to that device in order to process the functionality 00134 // else{ 00135 // //addDevice(deviceFound); 00136 // return devices[deviceFound]->interpret(lineData); 00137 // } 00138 // } 00139 // 00140 // return -1; 00141 //} 00142 // 00143 ///******************************************************************************/ 00144 ///*** <Function: loopCommand> ***/ 00145 ///******************************************************************************/ 00146 // 00147 //int loopCommand(FILE *selectedFile, LineData &lineData){ 00148 // 00149 // //Get the Condition value for number of times to loop 00150 // string loopCondition = lineData.word[1]; 00151 // int loopConditionValue = 0; 00152 // sscanf(loopCondition.c_str(), "%d", &loopConditionValue); 00153 // 00154 // int loopStartAddress = 0, loopLineNumber = 0, firstLineOfLoop = 1; 00155 // 00156 // lcd.setAddress(0,0); 00157 // lcd.printf("Cycle 1 of %d", loopConditionValue); 00158 // 00159 // Timer cycleTimer; 00160 // float totalLoopTime = 0; 00161 // cycleTimer.reset(); 00162 // cycleTimer.start(); 00163 // 00164 // int counter = 1, checkEnd = 0; 00165 // while (counter <= loopConditionValue){ 00166 // 00167 // getNextLine(selectedFile, lineData); 00168 // 00169 // //Must get the address before entering the interpret command 00170 // // if a Condition command is immediately after, and the condition fails, then 00171 // // the interpret command will return the line at the "end condition" line, and therefore 00172 // // set the loop's first line to be the "end condition" line, if interpretCommand is called BEFORE setting the first loop line address 00173 // if (firstLineOfLoop){ 00174 // loopStartAddress = lineData.lineAddress; //Save the Line Address 00175 // loopLineNumber = lineData.lineNumber; //Save the Line Number 00176 // firstLineOfLoop = 0; 00177 // } 00178 // 00179 // checkEnd = interpretCommand(selectedFile, lineData); 00180 // 00181 // //Increase the loop counter and go back to the beginning of the loop 00182 // if (checkEnd == 3){ 00183 // 00184 // //Output the Avg Cycle Time 00185 // cycleTimer.stop(); 00186 // totalLoopTime += cycleTimer.read(); 00187 // 00188 // lcd.setAddress(0,1); 00189 // lcd.printf("Avg t(sec): %1.3f", (totalLoopTime / counter)); 00190 // 00191 // //Output Cycle Number 00192 // counter++; 00193 // lcd.setAddress(0,0); 00194 // lcd.printf("Cycle %d of %d", counter, loopConditionValue); 00195 // 00196 // fseek(selectedFile, loopStartAddress, SEEK_SET); 00197 // lineData.lineNumber = loopLineNumber - 2; 00198 // checkEnd = 0; 00199 // 00200 // //Restart the timer for the next loop 00201 // cycleTimer.reset(); 00202 // cycleTimer.start(); 00203 // } 00204 // } 00205 // 00206 // return 1; 00207 // } 00208 // 00209 ///******************************************************************************/ 00210 ///*** <Function: conditionCommand> ***/ 00211 ///******************************************************************************/ 00212 // 00213 //int conditionCommand(FILE *selectedFile, LineData &lineData){ 00214 // 00215 // //Get the number of condition parameters 00216 // string numConditionVals = lineData.word[1]; 00217 // int numConditionValues = 0; 00218 // sscanf(numConditionVals.c_str(), "%d", &numConditionValues); 00219 // 00220 // //LineData tempLineData; 00221 // LineData param[15]; 00222 // //vector<LineData> param; 00223 // vector<ConditionOp> paramCondition; 00224 // 00225 // 00226 // //Fill the param Vector with Line structs of each individual device, this way we can send the Line struct to the appropriate interpret function without modification within the function itself 00227 // int i = 2, numParam = 0, paramNumWords = 0; 00228 // for (i = 2; i < lineData.numWords; i++){ 00229 // 00230 // // if the word is not an AND or an OR, it must mean it's for the specific function 00231 // // set the current parameter's next word to be equal to the current word we're checking 00232 // // increase number of words that the parameter has 00233 // if (lineData.word[i] != "AND" && lineData.word[i] != "xAND" && lineData.word[i] != "OR" && lineData.word[i] != "xOR"){ 00234 // 00235 // //tempLineData.word[paramNumWords] = lineData.word[i]; 00236 // param[numParam].word[paramNumWords] = lineData.word[i]; 00237 // paramNumWords++; 00238 // 00239 // //if this is the last word in the line.... 00240 // if(i == (lineData.numWords - 1)){ 00241 // param[numParam].numWords = paramNumWords; 00242 // paramCondition[numParam].op = NONE; 00243 // numParam++; 00244 // } 00245 // 00246 // } 00247 // 00248 // // if the word is an AND or an OR, it must mean the last function has been completely identified 00249 // // set the parameters number of Words value to the calculated value 00250 // // increase the number of Parameters (the current parameter function we're filling) 00251 // else if (lineData.word[i].compare("AND") == 0 || lineData.word[i].compare("xAND") == 0 || lineData.word[i].compare("OR") == 0 || lineData.word[i].compare("xOR") == 0){ 00252 // 00253 // //tempLineData.numWords = paramNumWords; 00254 // param[numParam].numWords = paramNumWords; 00255 // 00256 // paramCondition.push_back(ConditionOp()); 00257 // if (lineData.word[i].compare("AND") == 0) 00258 // paramCondition[numParam].op = AND; 00259 // else if (lineData.word[i].compare("xAND") == 0) 00260 // paramCondition[numParam].op = xAND; 00261 // else if (lineData.word[i].compare("OR") == 0) 00262 // paramCondition[numParam].op = OR; 00263 // else if (lineData.word[i].compare("xOR") == 0) 00264 // paramCondition[numParam].op = xOR; 00265 // 00266 // //param.push_back(LineData()); 00267 // //param[numParam] = tempLineData; 00268 // //param.push_back(tempLineData); //add it to the vector list of parameters 00269 // //tempLineData = LineData(); //reset 00270 // numParam++; // increase the index of param 00271 // paramNumWords = 0; // reset the number of words 00272 // } 00273 // } 00274 // 00275 // 00276 // vector<ConditionOp> combinedCondition; 00277 // ConditionOp tempCombinedCondition; 00278 // int j = 0, k = 0; 00279 // for (j = 0; j < numParam; j++){ 00280 // paramCondition[j].value = interpretCommand(selectedFile, param[j]); 00281 // } 00282 // 00283 // //create the combined Condition vector (take care of this xAND and xOR statements and combine them into one so that the whole vector is just AND's and OR's) 00284 // //this should make the xAND's / xOR's into a single member of the combinedCondition vector 00285 // enum ConditionType prevCondition = NONE; 00286 // int first = 1, last = 0; 00287 // for (k = 0; k < numParam; k++){ 00288 // 00289 // if (k == numParam - 1) 00290 // last = 1; 00291 // 00292 // if (!last){ 00293 // if (paramCondition[k].op != xAND && paramCondition[k].op != xOR && paramCondition[k + 1].op != xAND && paramCondition[k + 1].op != xOR){ 00294 // //AND 00295 // if (paramCondition[k].op == AND){ 00296 // if (!first && prevCondition != xAND && prevCondition != xOR) 00297 // combinedCondition.back().value = combinedCondition.back().value && paramCondition[k + 1].value; 00298 // else if (first || prevCondition == xAND || prevCondition == xOR){ 00299 // tempCombinedCondition.value = paramCondition[k].value && paramCondition[k + 1].value; 00300 // combinedCondition.push_back(tempCombinedCondition); 00301 // first = 0; 00302 // } 00303 // prevCondition = AND; 00304 // } 00305 // 00306 // //OR 00307 // else if (paramCondition[k].op == OR){ 00308 // if (!first && prevCondition != xAND && prevCondition != xOR) 00309 // combinedCondition.back().value = combinedCondition.back().value || paramCondition[k + 1].value; 00310 // else if (first || prevCondition == xAND || prevCondition == xOR){ 00311 // tempCombinedCondition.value = paramCondition[k].value || paramCondition[k + 1].value; 00312 // combinedCondition.push_back(tempCombinedCondition); 00313 // first = 0; 00314 // } 00315 // prevCondition = OR; 00316 // } 00317 // } 00318 // 00319 // // first value is something, not exclusive, but next values are exclusive 00320 // else if (first && (paramCondition[k].op == AND || paramCondition[k].op == OR) && (paramCondition[k + 1].op == xAND || paramCondition[k + 1].op == xOR)){ 00321 // tempCombinedCondition.value = paramCondition[k].value; 00322 // tempCombinedCondition.op = paramCondition[k].op; 00323 // combinedCondition.push_back(tempCombinedCondition); 00324 // prevCondition = paramCondition[k].op; 00325 // first = 0; 00326 // } 00327 // 00328 // else{ 00329 // //xAND 00330 // if (paramCondition[k].op == xAND){ 00331 // if (combinedCondition.size() == 0){ // No values so start a new combinedCondition 00332 // tempCombinedCondition.value = paramCondition[k].value && paramCondition[k + 1].value; 00333 // tempCombinedCondition.op = xAND; 00334 // combinedCondition.push_back(tempCombinedCondition); 00335 // prevCondition = xAND; 00336 // } 00337 // else{ 00338 // if (combinedCondition.back().op == xAND){ // AND the value to the back most combinedCondition 00339 // combinedCondition.back().value = combinedCondition.back().value && paramCondition[k + 1].value; 00340 // prevCondition = xAND; 00341 // } 00342 // else if (combinedCondition.back().op != xAND){ // Start a new combinedCondition 00343 // tempCombinedCondition.value = paramCondition[k].value && paramCondition[k + 1].value; 00344 // tempCombinedCondition.op = xAND; 00345 // combinedCondition.push_back(tempCombinedCondition); 00346 // prevCondition = xAND; 00347 // } 00348 // } 00349 // 00350 // } 00351 // 00352 // //xOR 00353 // else if (paramCondition[k].op == xOR){ 00354 // if (combinedCondition.size() == 0){ // No values so start a new combinedCondition 00355 // tempCombinedCondition.value = paramCondition[k].value || paramCondition[k + 1].value; 00356 // tempCombinedCondition.op = xOR; 00357 // combinedCondition.push_back(tempCombinedCondition); 00358 // prevCondition = xOR; 00359 // } 00360 // else{ 00361 // if (combinedCondition.back().op == xOR){ // OR the value to the back most combinedCondition 00362 // combinedCondition.back().value = combinedCondition.back().value || paramCondition[k + 1].value; 00363 // prevCondition = xOR; 00364 // } 00365 // else if (combinedCondition.back().op != xOR){ // Start a new combinedCondition 00366 // tempCombinedCondition.value = paramCondition[k].value || paramCondition[k + 1].value; 00367 // tempCombinedCondition.op = xOR; 00368 // combinedCondition.push_back(tempCombinedCondition); 00369 // prevCondition = xOR; 00370 // } 00371 // } 00372 // 00373 // } 00374 // 00375 // // Since the k + 1 value is included in the xAND or xOR exclusively, skip checking that value, and add the appropriate AND / OR as the 00376 // // operator of this exclusive xAND / xOR set 00377 // if ((paramCondition[k + 1].op == AND || paramCondition[k + 1].op == OR) && (prevCondition == xAND || prevCondition == xOR)){ 00378 // combinedCondition.back().op = paramCondition[k + 1].op; 00379 // k++; 00380 // } 00381 // 00382 // } 00383 // } 00384 // 00385 // 00386 // // the last value was not included in any combination, since directly before the last value was an xAND / xOR set that 00387 // // included the very last AND / OR as the set's operator, yet there is still another value that has not been combined, as it is supposed 00388 // // to be AND /OR to the exclusive xAND / xOR set 00389 // else if (last && (prevCondition == xAND || prevCondition == xOR) && (combinedCondition.back().op == AND || combinedCondition.back().op == OR)){ 00390 // tempCombinedCondition.value = paramCondition[k].value; 00391 // tempCombinedCondition.op = NONE; 00392 // combinedCondition.push_back(tempCombinedCondition); 00393 // } 00394 // 00395 // //reset the tempCombinedCondition variable 00396 // tempCombinedCondition = ConditionOp(); 00397 // } 00398 // 00399 // 00400 // // run through all values in the combined Condition vector, AND'ing / OR'ing as appropriate 00401 // // in the end, the last value in the array should be the final condition of the Condition statement... whether it was successful or failed 00402 // for (i = 0; i < (combinedCondition.size() - 1); i++){ 00403 // if (combinedCondition[i].op == AND) 00404 // combinedCondition[i + 1].value = combinedCondition[i].value && combinedCondition[i + 1].value; 00405 // else if (combinedCondition[i].op == OR) 00406 // combinedCondition[i + 1].value = combinedCondition[i].value || combinedCondition[i + 1].value; 00407 // } 00408 // 00409 // int conditionSuccess = combinedCondition.back().value; //value is the success(1) or failure(0) of the condition statement 00410 // 00411 // int checkEnd = 0; 00412 // if (!conditionSuccess){ 00413 // 00414 // while (checkEnd != 4){ 00415 // 00416 // getNextLine(selectedFile, lineData); 00417 // 00418 // // check if the first word is an end command (avoids interpreting functions that perform actions) 00419 // if (lineData.word[0].compare("end") == 0) 00420 // checkEnd = interpretCommand(selectedFile, lineData); 00421 // 00422 // if (checkEnd == 4) // custom return value for this function 00423 // return 0; 00424 // } 00425 // } 00426 // 00427 // // Return success as the function either met the condition and will continue from the next line, or 00428 // // failed to meet the condition and ran through the lines inside the condition until "end condition" was found, therefore 00429 // // the program will proceed from the line after the "end condition" line 00430 // return 1; 00431 //}
Generated on Sun Jul 24 2022 01:49:11 by
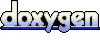