
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
TextFile.h
00001 #ifndef TEXTFILE_HPP 00002 #define TEXTFILE_HPP 00003 00004 #include "mbed.h" 00005 #include "TextLCD.h" 00006 #include "SDFileSystem.h" 00007 #include <stdio.h> 00008 #include <string> 00009 #include <stdlib.h> 00010 #include <vector> 00011 using std::string; 00012 00013 #include <iostream> 00014 #include <istream> 00015 #include <ostream> 00016 #include <iterator> 00017 #include <sstream> 00018 00019 00020 00021 //vector<string> filenames; //filenames are stored in a vector string 00022 00023 vector<string> readFileNames(char []); 00024 int getFileNamesWithoutExt(string[], vector<string> &); 00025 string getPreviousLine(istream &); 00026 00027 00028 00029 /******************************************************************************/ 00030 /*** <readFileNames> ***/ 00031 /******************************************************************************/ 00032 // read file names into vector of strings 00033 vector<string> readFileNames(char *dir) { 00034 00035 vector<string> filenames; 00036 DIR *dp; 00037 struct dirent *dirp; 00038 00039 00040 dp = opendir(dir); 00041 00042 //if no directory was found, don't try and get the fileNames and return an empty vector of 0 00043 if (dp != NULL){ 00044 00045 //read all directory and file names in current directory into filename vector 00046 while((dirp = readdir(dp)) != NULL) { 00047 filenames.push_back(string(dirp->d_name)); 00048 } 00049 closedir(dp); 00050 } 00051 return filenames; 00052 } 00053 00054 00055 00056 /******************************************************************************/ 00057 /*** <getFileNamesWithoutExt> ***/ 00058 /******************************************************************************/ 00059 // save filename strings to array from vector using an iterator 00060 int getFileNamesWithoutExt(vector<string> &textFiles, vector<string> filenames) { 00061 00062 //Cycle through all files listed in the directoy (strings in the vector list) 00063 int n = 0; 00064 for(vector<string>::iterator it=filenames.begin(); it < filenames.end(); it++){ 00065 00066 vector<string> fileName; //filename[0] = filename, filename[1] = extension 00067 00068 stringstream ss; 00069 ss << (*it); 00070 00071 //parse the array based on a '.' delimeter, effectively getting the file name and extension 00072 string item; 00073 while (getline(ss, item, '.')) { 00074 fileName.push_back(item); 00075 } 00076 00077 // if the fileName vector has two items (a name and an extension) and is a .txt file, then save it to the array 00078 if (fileName.size() == 2 && fileName[1].compare("txt") == 0) { 00079 textFiles.push_back(fileName[0]); 00080 n++; 00081 } 00082 } 00083 00084 return n; //Return the number of txt files that were found in the directory 00085 } 00086 00087 /******************************************************************************/ 00088 /*** <getNextLine> ***/ 00089 /******************************************************************************/ 00090 // 00091 00092 int getNextLine(FILE *selectedFile, LineData &lineData) { 00093 00094 int validLine = 0; 00095 while (!validLine){ 00096 00097 lineData.lineAddress = ftell(selectedFile); 00098 00099 if (lineData.lineAddress == -1L){ 00100 ErrorOut("Unable to get address of line, SD Card Removed?", lineData.lineNumber + 1); 00101 return -1; 00102 } 00103 00104 //char *newLine; 00105 //getline(selectedFile, newLine); 00106 char newLine[MAX_LINE_LENGTH]; 00107 00108 fgets(newLine, MAX_LINE_LENGTH, selectedFile); 00109 00110 if (newLine == NULL){ 00111 ErrorOut("Unable to get the next line, SD Card Removed?", lineData.lineNumber + 1); 00112 return -1; 00113 } 00114 00115 lineData.lineNumber++; // new line successfully found, increase lineNumber 00116 00117 //pull out each individual word (separated by any white space), and place it in the vector 00118 stringstream newLineStrm(newLine); 00119 istream_iterator<string> it(newLineStrm); 00120 istream_iterator<string> end; 00121 vector<string> results(it, end); 00122 00123 //Get the size of the results vector, if it is 0 then while loop will loop again, 00124 //if it has a value, then it will accept the line and end looping cycles 00125 validLine = results.size(); 00126 00127 //copy the vector of results into the array of words 00128 copy(results.begin(), results.end(), lineData.word); 00129 00130 lineData.numWords = results.size(); //Record the number of words in the line 00131 00132 results.erase(results.begin(), results.end()); //remove the results vector from memory 00133 } 00134 00135 //Update the Current Line Number 00136 lcd.setAddress(0,2); 00137 lcd.printf("Current Line#: %d ", lineData.lineNumber); 00138 00139 //Update the cmd/dvc label for the line so that the user has an idea of what's going on 00140 lcd.setAddress(0,3); 00141 lcd.printf(" "); // Clear the Line using Spaces (Emptyness) - Note one line is 20 Characters 00142 lcd.setAddress(0,3); 00143 lcd.printf("cmd/dvc: %s", lineData.word[0]); 00144 00145 /* 00146 lcd.cls(); //clear the display 00147 lcd.setAddress(0,0); 00148 lcd.printf("wrd1: %s", lineData.word[0]); 00149 lcd.setAddress(0,1); 00150 lcd.printf("wrd2: %s", lineData.word[1]); 00151 lcd.setAddress(0,2); 00152 lcd.printf("wrd3: %s", lineData.word[2]); 00153 lcd.setAddress(0,3); 00154 lcd.printf("wrd4: %s", lineData.word[3]); 00155 wait(1);*/ 00156 } 00157 00158 00159 #endif 00160 00161 00162 00163 00164
Generated on Sun Jul 24 2022 01:49:11 by
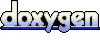